In TypeScript, instanceof
is a type guard that allows you to narrow down the
type of an object within a conditional block.
Understanding instanceof
Type Guard ๐คโ
The instanceof
type guard is especially useful when you're working with
classes and inheritance, as it checks if an object is an instance of a specific
class or any of its subclasses. When you use instanceof
in a conditional
expression, TypeScript automatically narrows the type of the object within the
block, allowing you to access and use properties and methods specific to that
class without causing type errors.
Here's an example to illustrate the concept:
class Animal {
makeSound(): void {
console.log("Some generic sound");
}
}
class Dog extends Animal {
bark(): void {
console.log("Woof!");
}
}
class Cat extends Animal {
meow(): void {
console.log("Meow!");
}
}
function handleAnimal(animal: Animal): void {
if (animal instanceof Dog) {
// TypeScript knows that 'animal' is a Dog within this block
animal.bark(); // No type error
} else if (animal instanceof Cat) {
// TypeScript knows that 'animal' is a Cat within this block
animal.meow(); // No type error
} else {
animal.makeSound(); // No type error, as it's a method of Animal
}
}
const dog = new Dog();
const cat = new Cat();
handleAnimal(dog);
handleAnimal(cat);
In this example, we define three classes: Animal
, Dog
, and Cat
. The Dog
and Cat
classes extend the Animal
class. In the handleAnimal
function, we
use the instanceof
type guard to determine the specific type of the animal
parameter. Within each conditional block, TypeScript automatically narrows the
type of animal
, allowing us to call the appropriate methods without causing
any type errors.
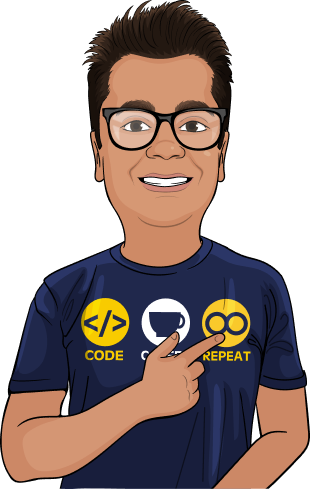
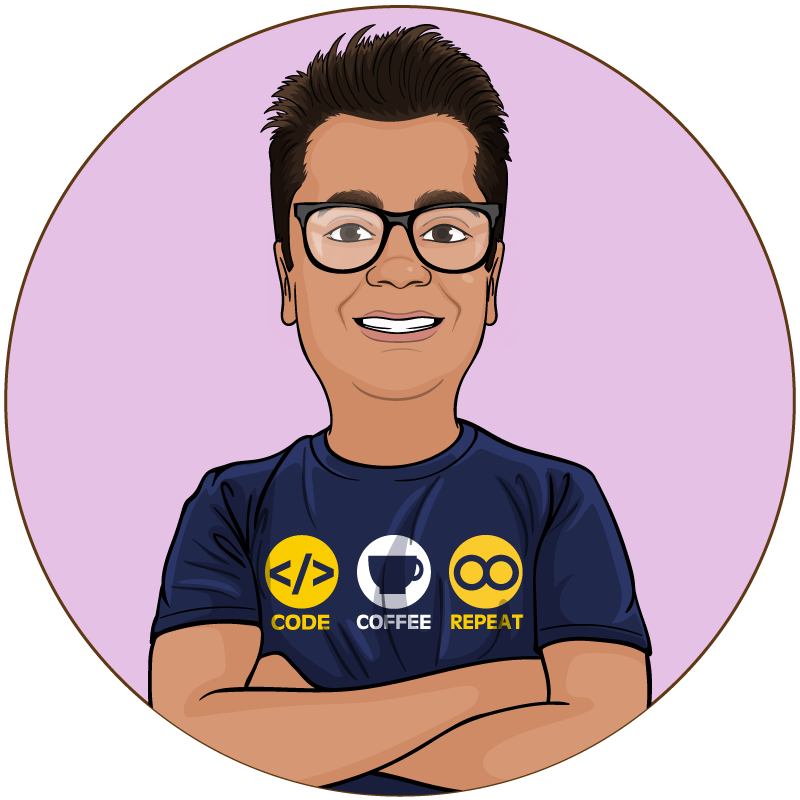
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Example ๐โ
Let's consider a real-world example of an e-commerce application where
the instanceof
type guard can be useful. In this application, we have
different types of products with unique properties and methods. We will use
the instanceof
type guard to process these products accordingly.
abstract class Product {
constructor(public name: string, public price: number) {
}
abstract getPrice(): number;
}
class Electronics extends Product {
constructor(name: string, price: number, public warranty: number) {
super(name, price);
}
getPrice(): number {
return this.price + this.warranty * 10;
}
}
class Clothing extends Product {
constructor(
name: string,
price: number,
public size: string,
public material: string
) {
super(name, price);
}
getPrice(): number {
return this.price * (this.material === "Cotton" ? 1.1 : 1.2);
}
}
function displayProductDetails(product: Product): void {
console.log(`Product: ${product.name}`);
console.log(`Price: $${product.getPrice()}`);
if (product instanceof Electronics) {
console.log(`Warranty: ${product.warranty} months`);
} else if (product instanceof Clothing) {
console.log(`Size: ${product.size}`);
console.log(`Material: ${product.material}`);
}
}
const smartphone = new Electronics("Smartphone", 500, 12);
const tshirt = new Clothing("T-Shirt", 30, "M", "Cotton");
displayProductDetails(smartphone);
displayProductDetails(tshirt);
In this example, we have an abstract Product
class with two
subclasses, Electronics
and Clothing
. Each subclass has its unique
properties and methods. In the displayProductDetails
function, we use
the instanceof
type guard to check the specific type of the product
parameter.
When the product
is an instance of Electronics
, TypeScript knows that the
object is of type Electronics
within that block, allowing us to access
the warranty
property without any type errors. Similarly, when the product
is an instance of Clothing
, TypeScript narrows the type to Clothing
, and we
can safely access the size
and material
properties.
This example demonstrates how the instanceof
type guard can be used to
differentiate between different classes and their properties in a real-world
application, making the code more type-safe and easier to maintain.
What Can You Do Next ๐๐โ
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.