They are a powerful and expressive feature borrowed from the decorator pattern in other languages, such as Python.
Understanding Decorators ๐คโ
Decorators in TypeScript are a special kind of declaration that can be attached to a class, method, property, or parameter to modify their behavior or add metadata to them.
Decorators are functions that can be used to alter or enhance the behavior of the entity they are attached to. They are invoked with the decorated item as an argument, and they can modify, wrap, or replace the original functionality of that item.
In TypeScript, decorators are prefixed with the @
symbol, followed by the
decorator function name. They can be applied to various elements of your code,
such as:
- Class decorators: Applied to the class constructor.
- Method decorators: Applied to a method within the class.
- Property decorators: Applied to a property within the class.
- Parameter decorators: Applied to a parameter within a method of the class.
Here's a simple example of a class decorator:
function LogClass(target: Function) {
console.log(`Class ${target.name} has been decorated.`);
}
@LogClass
class MyClass {
// Class implementation
}
In this example, the LogClass
decorator will log a message to the console when
the MyClass
class is defined.
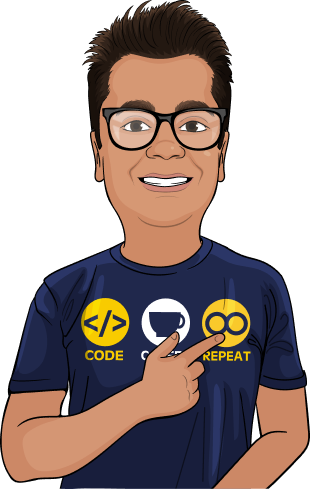
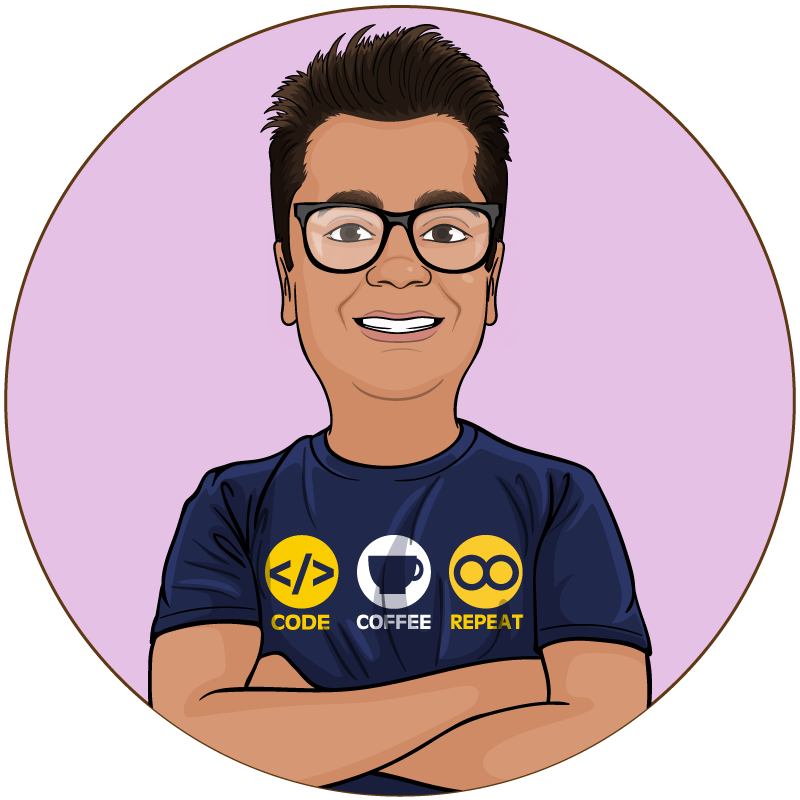
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSDecorator Factories ๐คโ
Decorator factories in TypeScript are functions that return a decorator. They can be used to parameterize decorators, allowing you to pass additional arguments to customize the behavior of the decorator based on the provided parameters. This can make decorators more flexible and reusable.
Here's the previous example, converted to use a decorator factory:
function LogClassFactory(logMessage: string) {
return function (target: Function) {
console.log(`${logMessage}: Class ${target.name} has been decorated.`);
};
}
@LogClassFactory("Custom message")
class MyClass {
// Class implementation
}
In this example, LogClassFactory
is a decorator factory that takes
a logMessage
parameter. It returns a new function (the actual decorator) that
takes a target
parameter (the class constructor). When the decorator is
applied to the MyClass
class, the logMessage
is passed to the decorator
factory, which then returns the decorator function that logs the custom message
along with the class name.
Now, when the MyClass
class is defined, the console log will show the custom
message specified in the decorator factory call:
What Can You Do Next ๐๐โ
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.