TypeScript compiler infer a more specific type based on the usage of
equality checks (e.g., ===
, !==
, ==
, !=
).
Understanding Equality Narrowing ๐คโ
In TypeScript, "equality narrowing" refers to a type narrowing technique that
helps the TypeScript compiler infer a more specific type based on the usage of
equality checks (e.g., ===
, !==
, ==
, !=
). Type narrowing is the process
of refining a broader type to a more specific one, often used in conditional
branches, which allows the compiler to provide better type safety and catch
potential bugs.
Equality narrowing is particularly useful when working with union types, which are types that represent one of several possible types. When the code contains a conditional expression that checks for a specific value or type within the union type, TypeScript narrows the type within that branch accordingly.
Here's an example to illustrate equality narrowing:
type Shape =
{ kind: 'circle', radius: number }
| { kind: 'square', sideLength: number };
function getArea(shape: Shape): number {
if (shape.kind === 'circle') {
// TypeScript narrows the type of 'shape' to { kind: 'circle', radius: number }
return Math.PI * shape.radius ** 2;
} else {
// TypeScript narrows the type of 'shape' to { kind: 'square', sideLength: number }
return shape.sideLength ** 2;
}
}
In this example, Shape
is a union type that can represent either a circle or a
square. When we check for shape.kind === 'circle'
, TypeScript narrows the type
of shape
within that block to { kind: 'circle', radius: number }
. In
the else
block, TypeScript narrows the type of shape
to { kind: 'square', sideLength: number }
. With these narrowed types,
TypeScript can ensure that we are accessing the correct properties (
e.g., radius
for circles and sideLength
for squares) and catch potential
bugs at compile time.
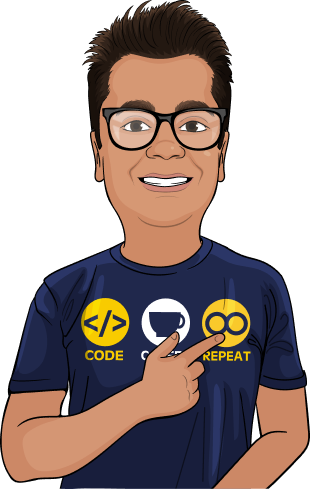
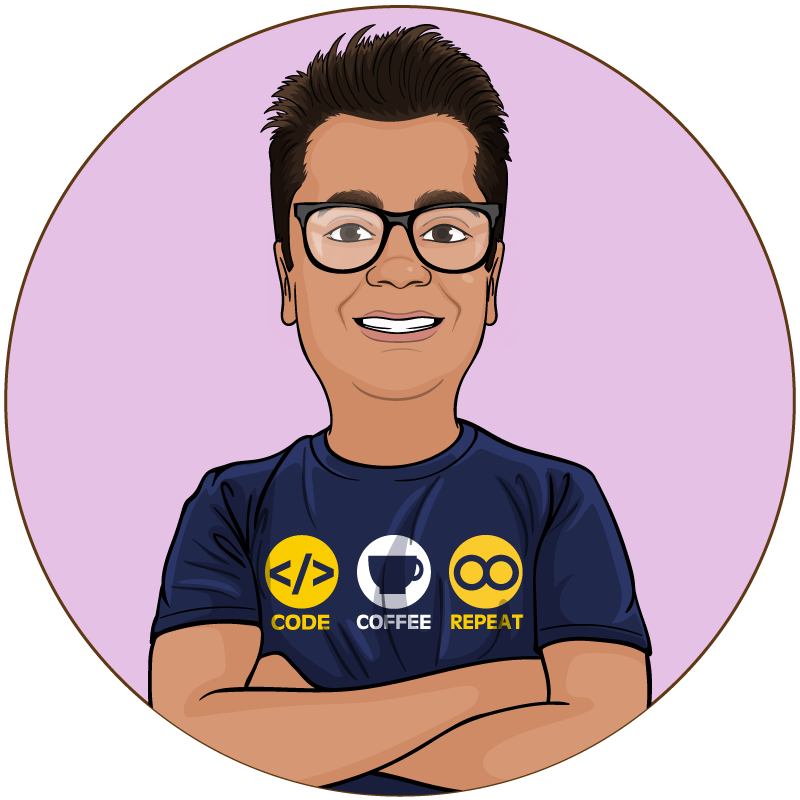
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Example ๐โ
Equality narrowing is useful in real-world applications when dealing with different types of data that have common properties but require different handling based on their specific type. Let's consider an example of an e-commerce platform that supports various types of discounts for customers.
type FixedDiscount = {
kind: 'fixed',
discountAmount: number,
};
type PercentageDiscount = {
kind: 'percentage',
discountPercentage: number,
};
type VolumeDiscount = {
kind: 'volume',
minQuantity: number,
discountPercentage: number,
};
type Discount = FixedDiscount | PercentageDiscount | VolumeDiscount;
function calculateDiscountedPrice(price: number, quantity: number, discount: Discount): number {
if (discount.kind === 'fixed') {
// TypeScript narrows the type of 'discount' to FixedDiscount
return Math.max(0, price - discount.discountAmount) * quantity;
} else if (discount.kind === 'percentage') {
// TypeScript narrows the type of 'discount' to PercentageDiscount
return price * (1 - discount.discountPercentage / 100) * quantity;
} else {
// TypeScript narrows the type of 'discount' to VolumeDiscount
return quantity >= discount.minQuantity
? price * (1 - discount.discountPercentage / 100) * quantity
: price * quantity;
}
}
In this example, the e-commerce platform supports three types of discounts:
fixed amount, percentage, and volume-based discounts. Each discount type has a
unique property (discountAmount
, discountPercentage
, or minQuantity
) that
affects how the discount is applied.
Using equality narrowing, we can safely access the relevant properties of each
discount type based on the kind
property. The TypeScript compiler will catch
any type errors, ensuring that our function is correct and preventing potential
bugs in the application logic. This increases the robustness of our code and
reduces the chance of introducing errors during development or refactoring.
In summary, equality narrowing helps improve the maintainability, safety, and correctness of real-world applications that deal with different data types with common properties but require specific handling based on their type.
What Can You Do Next ๐๐โ
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.