In this article, we will delve into the advantages of using TypeScript alongside React, discussing how this powerful combination can enhance your development experience.
TypeScript and React a Lethal Combination
If you have been considering incorporating TypeScript into your React projects, this comprehensive analysis aims to provide you with the information you need to make an informed decision.
We will examine the key benefits of TypeScript and React, including type checking, code readability, autocompletion and IntelliSense, refactoring, and scalability. By the end of this article, you will have a clear understanding of how TypeScript can improve your React projects, and you may be inspired to adopt this useful language. Let's begin our exploration of TypeScript and React.
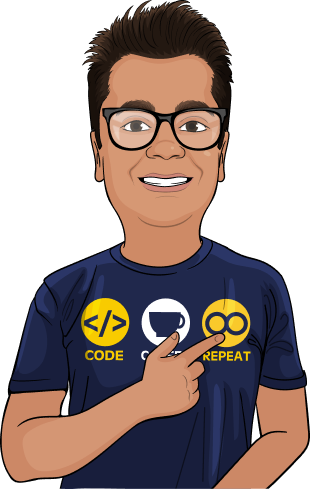
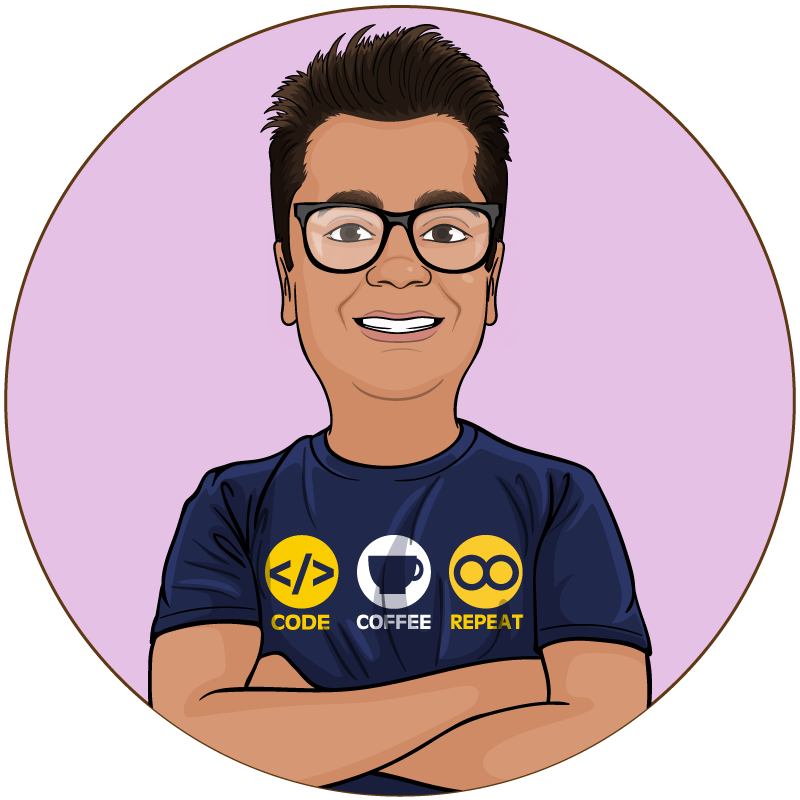
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSAdvantages of using TypeScript with React
Certainly! Here's an in-depth explanation of each advantage of using TypeScript with React:
Type checking
TypeScript's static type checking ensures that variables, function parameters, and object properties adhere to their expected types. This enables early detection of potential errors, such as type mismatches or incorrect property access, during development. By catching these errors before runtime, you can reduce the number of bugs that end-users may encounter.
For example, when using TypeScript with React, you can create interfaces or type aliases to define the shape and types of your component props. This makes it clear which props are required and what type they should be, ensuring that components are used correctly throughout the application.
Example: Let's say we have a User
component that displays user details.
interface UserProps {
id: number;
name: string;
email: string;
}
const User: React.FC<UserProps> = ({ id, name, email }) => {
return (
<div>
<h2>{name}</h2>
<p>ID: {id}</p>
<p>Email: {email}</p>
</div>
);
};
If we mistakenly pass a string instead of a number for the id
prop, TypeScript
will catch the error during development:
// This will cause a TypeScript error,
// as id should be a number, not a string.
<User id="1" name="John Doe" email="john.doe@example.com" />
Code readability
TypeScript enhances code readability by providing clear type annotations and interfaces that describe the expected structure and behavior of variables, components, and functions. These annotations serve as a form of documentation, making it easier for developers to understand what a piece of code does and how it should be used.
In React applications, using TypeScript can clarify the relationships between components, their props, and any shared state or utility functions. This can be particularly helpful in large projects or when working with a team, as it simplifies the process of understanding and maintaining the codebase.
Example: The User
component from the previous example already illustrates
code
readability by clearly defining the expected props and their types using an
interface. This makes the component easier to understand and use.
Autocompletion and IntelliSense
TypeScript's type information enables better autocompletion and IntelliSense features in modern code editors like Visual Studio Code. As you type, the editor can suggest available properties, methods, or variables based on the context and expected types. This speeds up the development process and reduces the likelihood of typos or incorrect usage.
Additionally, TypeScript-powered IntelliSense provides inline documentation, allowing developers to see the expected types, descriptions, and parameter information of functions or components without having to consult external documentation.
Example: Imagine we have a utility function that takes a user object and returns a formatted full name.
interface User {
firstName: string;
lastName: string;
}
export function getFullName(user: User): string {
return `${user.firstName} ${user.lastName}`;
}
When using this function in another file, TypeScript-powered autocompletion will
suggest the getFullName
function, display its expected parameter
type (User
),
and show its return type (string
).
Refactoring
TypeScript makes refactoring code safer and more efficient. When you change the structure, types, or properties of your code, TypeScript's type checking ensures that any affected parts of the application are updated accordingly. This helps prevent potential issues that could arise from incomplete or incorrect refactoring.
For instance, if you change the name of a property in a component's props interface, TypeScript will alert you to any places in the code where the old property name is still being used, allowing you to update it accordingly.
Example: Suppose we decide to change the UserProps
interface for the
User
component, renaming the email prop to userEmail
.
interface User {
firstName: string;
lastName: string;
}
export function getFullName(user: User): string {
return `${user.firstName} ${user.lastName}`;
}
With TypeScript, we'll get an error if we still try to use the old email
prop:
// This will cause a TypeScript error,
// as email no longer exists in UserProps.
<User id={1} name="John Doe" email="john.doe@example.com" />
This makes it easy to identify and fix any places in the code where the old prop name is still being used.
Scalability
TypeScript's strong typing, interfaces, and other features make it well-suited for large-scale projects. As applications grow, TypeScript can help maintain organization and structure, making it easier to navigate and understand the codebase.
In React applications, TypeScript can enforce consistent patterns for components, state management, and utility functions, which can prevent bugs and simplify maintenance. By promoting a modular architecture, TypeScript makes it easier to split a large application into smaller, more manageable parts.
Example: Imagine we have a large application with multiple user-related
components, such as UserList
, UserDetail
, and UserForm
. We can create a
shared
User
interface and use it consistently across these components.
// src/types/User.ts
export interface User {
id: number;
name: string;
email: string;
}
// src/components/UserList.tsx
import { User } from "../types/User";
interface UserListProps {
users: User[];
}
const UserList: React.FC<UserListProps> = ({ users }) => {
//... (component code)
};
// src/components/UserDetail.tsx
import { User } from "../types/User";
interface UserDetailProps {
user: User;
}
const UserDetail: React.FC<UserDetailProps> = ({ user }) => {
//... (component code)
};
// src/components/UserForm.tsx
import { User } from "../types/User";
interface UserFormProps {
onSubmit: (user: User) => void;
}
const UserForm: React.FC<UserFormProps> = ({ onSubmit }) => {
//... (component code)
};
By using the shared User
interface across the application, we ensure consistent
typing and structure for user-related data, making the codebase more manageable
and maintainable. This is especially important for large applications, where
TypeScript's features can significantly improve organization and scalability.
In summary, using TypeScript with React offers numerous benefits, including improved type checking, enhanced code readability, better tooling support, safer refactoring, and increased scalability.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.