It's possible to to use React with TypeScript and in my personal opinion its a must to use TypeScript with React. Using TypeScript with React gives you the advantage of strict type checking, better code readability, autocompletion, intellisense, easier refactoring and makes your code base scalable.
Can You Use React With Typescript?
React can be easily used with TypeScript to create robust and maintainable web applications. TypeScript is a statically-typed superset of JavaScript that adds optional type annotations, interfaces, and other features that help catch errors during development, improve code readability, and provide better tooling support.
Using React With TypeScript
To use React with TypeScript, follow these steps:
Step One
Set up a new React project with TypeScript support:
You can create a new React project with TypeScript support using the Create React App (CRA) tool by running the following command:
npx create-react-app my-app --template typescript
This command will create a new React project with TypeScript support and the necessary configurations.
Step Two
Use TypeScript files:
Rename your .js
and .jsx
files to .ts
and .tsx
, respectively.
The .tsx
extension is used for React components that include JSX syntax.
Step Three
Update base component definitions:
In this step we will update the pdate the base App
component created by Create
React App (CRA) to comply with TypeScript's strict typing:
- First, open the
App.tsx
file created by CRA. The initial code should look something like this:
import React from "react";
import logo from "./logo.svg";
import "./App.css";
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.tsx</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
- Update the component definition to use TypeScript's strict typing:
To do this, we'll change the function App()
syntax to a const App: React.FC
syntax:
import React from "react";
import logo from "./logo.svg";
import "./App.css";
// Update the component definition to use TypeScript's strict typing
const App: React.FC = () => {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>Hello World! From app.tsx</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
};
export default App;
In this example, we've updated the base App
component to use the React.FC
(
Function Component) type, which enforces strict typing for functional components
in TypeScript. By using React.FC
, we're ensuring that the App
component is
typed correctly and adheres to React's best practices.
Note that the React.FC
type definition is optional and not strictly required,
but it can be useful for enforcing strict typing, especially in larger projects
or when working with a team.
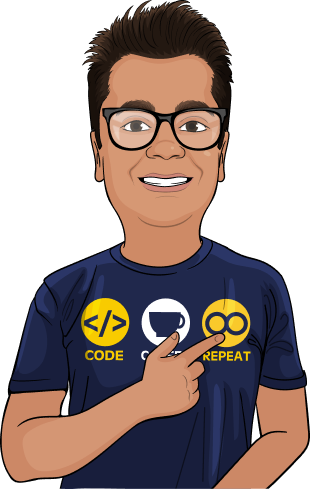
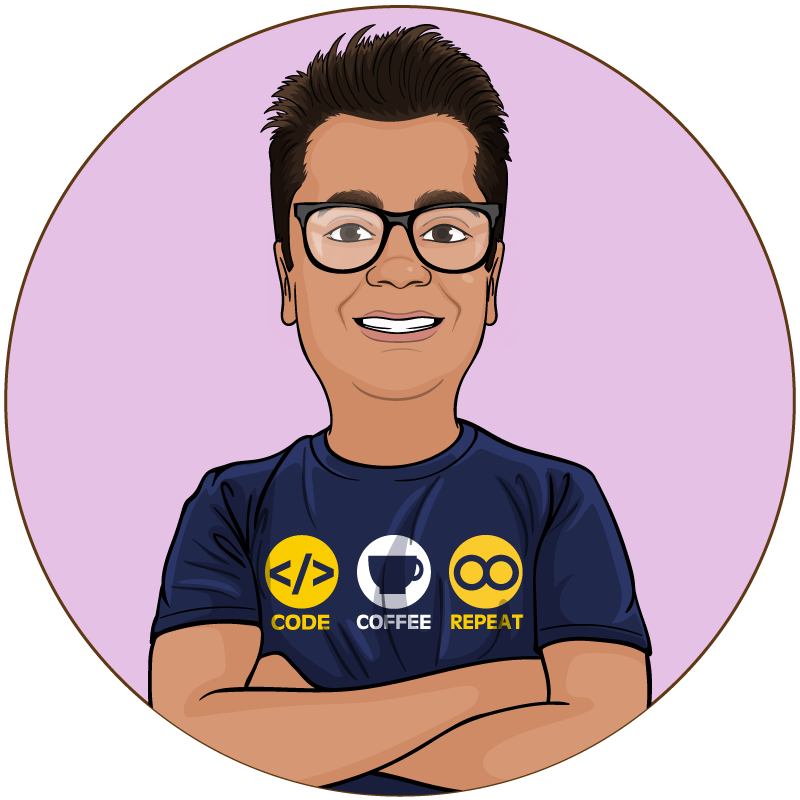
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSCreating a Custom Component
Here's an example of a custom React component using TypeScript. This example
demonstrates a simple Greeting
component that takes two props: name
and age
.
Create a new file called
Greeting.tsx
.Add the following TypeScript code to the
Greeting.tsx
file:
import React from "react";
// Define the props interface
interface GreetingProps {
name: string;
age: number;
}
// Create the Greeting component
const Greeting: React.FC<GreetingProps> = ({ name, age }) => {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
};
export default Greeting;
In this example, we've defined a custom React component called Greeting
that
takes two props: name
(a string) and age
(a number). We use an interface
called GreetingProps
to describe the expected shape and types of these props.
We then create the Greeting
component using the React.FC
type and
the GreetingProps
interface, which ensures that the component is correctly
typed and adheres to React best practices. The component renders an h1
element
with a greeting message and a p
element displaying the user's age.
To use this custom Greeting
component in another component, such as App
, you
can import it and include it in the JSX:
import React from "react";
import "./App.css";
import Greeting from "./Greeting";
const App: React.FC = () => {
return (
<div className="App">
<header className="App-header">
<Greeting name="John Doe" age={30} />
</header>
</div>
);
};
export default App;
In this example, the Greeting
component is included in the App
component's
JSX, and the name
and age
props are passed to it.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.