Truthiness Narrowing is particularly useful when dealing with optional types, union types, or null/undefined values.
Understanding Truthiness Narrowing ๐คโ
In TypeScript, "truthiness narrowing" refers to the process of refining the type of a variable based on a condition that checks for its "truthiness" or " falsiness." This is particularly useful when dealing with optional types, union types, or null/undefined values.
In JavaScript (and by extension, TypeScript), a value is considered "truthy" if
it coerces to true in a boolean context, and "falsy" if it coerces to false.
Common falsy values include null
, undefined
, false
, 0
, NaN
, and empty
strings (''
), while everything else is considered truthy.
When TypeScript encounters a truthiness check, it narrows down the type of the variable based on the outcome of the check. Let's look at an example.
Consider the following TypeScript code:
type Person = {
name: string;
age?: number;
};
function printAge(person: Person) {
if (person.age) {
console.log(`Age: ${person.age}`); // person.age is narrowed to 'number'
} else {
console.log('Age unknown');
}
}
In this example, we have a Person
type with an optional age
property.
The printAge
function takes a Person
object and checks if the age
property
is truthy. If it is, TypeScript narrows the type of person.age
from number | undefined
to number
within the if
block, as it is now
certain that person.age
has a value. If the age
property is falsy (
i.e., undefined
), the else
block will execute.
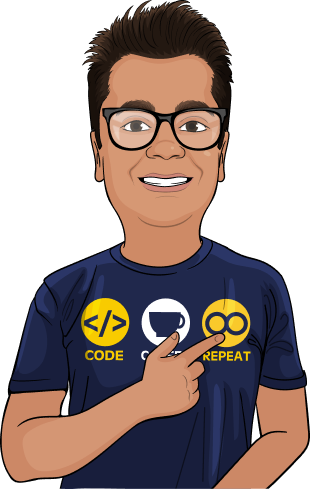
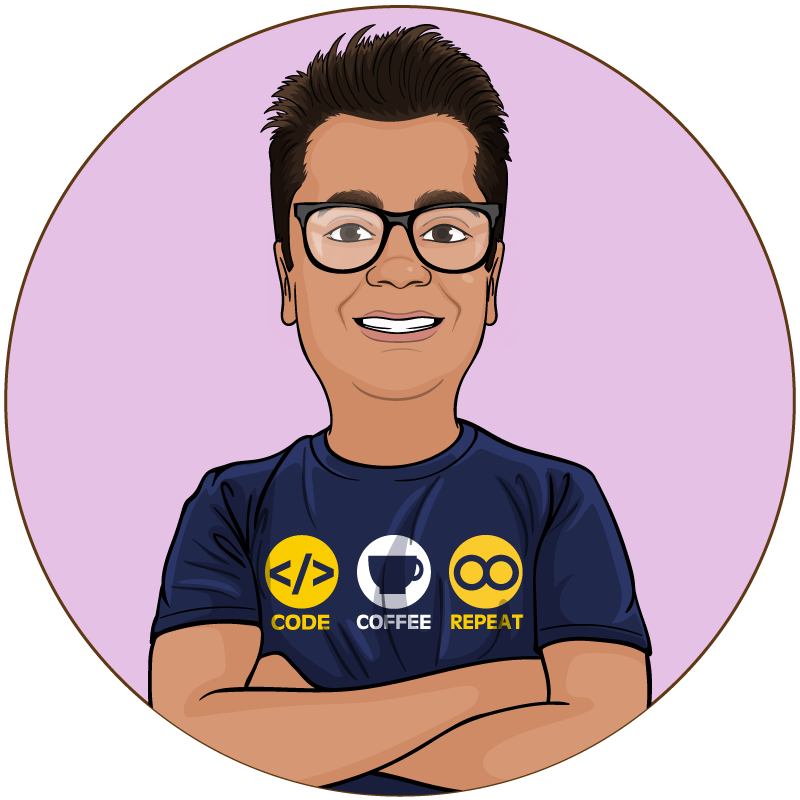
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSHow is It Different From JavaScript ๐โ
Truthiness narrowing in TypeScript is different from JavaScript in that it adds static type checking and type inference capabilities to the language. While JavaScript also checks for truthiness and falsiness of values, it does not provide compile-time type information or type checking. TypeScript leverages this concept to refine the types of variables based on truthiness checks, providing better type safety and improved developer experience.
The additional value that truthiness narrowing provides in TypeScript includes:
Type safety: TypeScript's type system narrows down the types of variables based on truthiness checks, helping catch potential type-related issues during the compile-time. This can help prevent runtime errors caused by incorrect types being used in certain operations.
Type inference: TypeScript can infer the types of variables within the narrowed scope of a truthiness check, providing better type information and reducing the need for manual type annotations.
Improved developer experience: TypeScript's truthiness narrowing allows for better code completion, type hinting, and error detection in integrated development environments (IDEs), making it easier for developers to write correct and efficient code.
Self-documenting code: By using TypeScript's type system and truthiness narrowing, developers can more effectively communicate their intentions for a variable or function, making the code more readable and maintainable. Tools like TSDoc and Compodoc can help generate documentation from your TypeScript code and provide more detailed information about your codebase.
- TSDoc: TSDoc is a documentation standard for TypeScript, aimed at providing a consistent way to write comments and generate API documentation for TypeScript projects. It's similar to JSDoc but tailored specifically for TypeScript's syntax and features. TSDoc provides a syntax for writing comments that include type annotations, descriptions, and tags (such as @param, @returns, and @example) to provide detailed information about a function or class.
- Compodoc: Compodoc is a documentation generator specifically designed for Angular applications. It helps developers generate documentation for Angular projects by analyzing the TypeScript code, HTML templates, and CSS styles. Compodoc can generate documentation that includes information about components, directives, services, pipes, modules, and more. It supports features such as automatic table of contents generation, search functionality, and even live reloading during development.
In summary, truthiness narrowing in TypeScript enhances the value of JavaScript's truthiness checks by integrating them into the type system, improving type safety, type inference, developer experience, and code maintainability.
What Can You Do Next ๐๐โ
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.