Type predicates are used to determine if a given value belongs to a certain type or not, providing a hint to the TypeScript compiler about the type information.
What are Type Predicates ๐คโ
Type predicates are a specific kind of function that allows you to narrow down the type of a variable or a parameter within a specific branch of your code. They are used to determine if a given value belongs to a certain type or not, providing a hint to the TypeScript compiler about the type information.
A type predicate has a specific syntax that includes the is
keyword, followed
by the type you want to check against. Here's an example of a type predicate:
function isString(value: any): value is string {
return typeof value === 'string';
}
In this example, isString
is a type predicate that checks if a given value is
a string. The type predicate is defined by value is string
. This tells the
TypeScript compiler that if the function returns true
, the value
parameter
should be considered a string
within the scope where the type predicate is
used.
Here's an example of how you would use a type predicate:
function processValue(value: string | number) {
if (isString(value)) {
// TypeScript now knows that 'value' is a string within this block
console.log(value.toUpperCase());
} else {
// TypeScript now knows that 'value' is a number within this block
console.log(value.toFixed(2));
}
}
processValue('hello');
processValue(42);
In this example, the processValue
function accepts a parameter value
of
type string | number
. By using the isString
type predicate within the
conditional, the TypeScript compiler can infer that the type of value
is
narrowed down to string
in the first branch and number
in the second branch.
This allows you to use type-specific methods and properties without type errors.
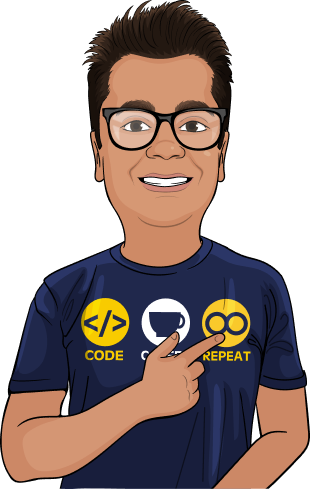
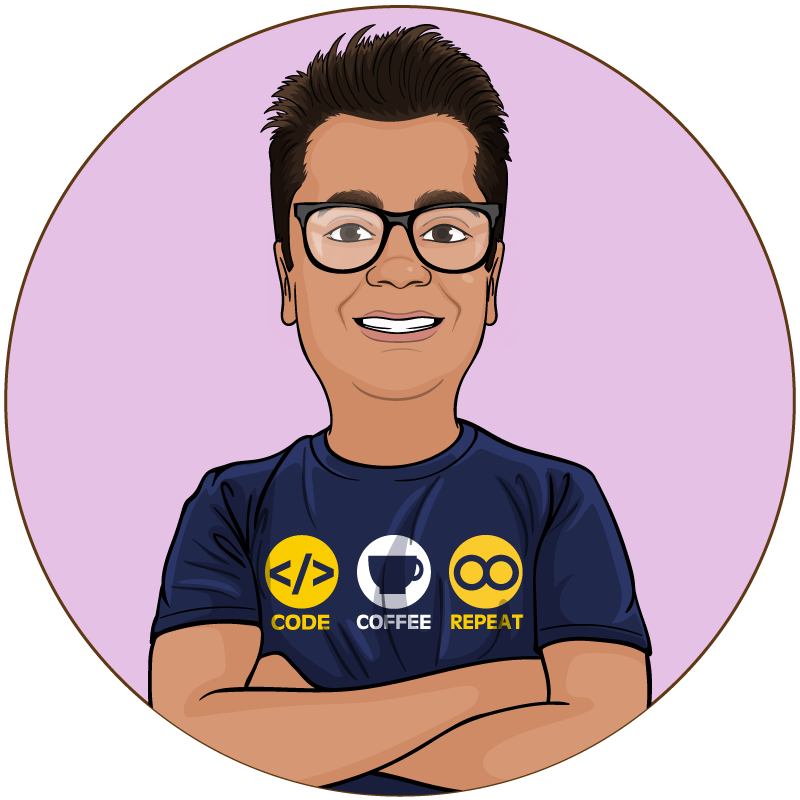
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal World Application ๐โ
In a real-life application, type predicates can be particularly useful when working with complex data structures or when interacting with APIs that return mixed types. Let's take an example of an e-commerce application that receives a list of products from an API. Each product can have different types of properties based on its category.
Here's an example of the data structure:
interface Book {
type: 'book';
title: string;
author: string;
pageCount: number;
}
interface Electronics {
type: 'electronics';
name: string;
brand: string;
model: string;
powerUsage: number;
}
type Product = Book | Electronics;
We have two types of products: Book
and Electronics
. Both have a unique
property type
. We can create type predicates to narrow down the type of
product:
function isBook(product: Product): product is Book {
return product.type === 'book';
}
function isElectronics(product: Product): product is Electronics {
return product.type === 'electronics';
}
Now, let's say we need to display product details based on their type:
function displayProductDetails(product: Product) {
if (isBook(product)) {
console.log(`Title: ${product.title}`);
console.log(`Author: ${product.author}`);
console.log(`Page count: ${product.pageCount}`);
} else if (isElectronics(product)) {
console.log(`Name: ${product.name}`);
console.log(`Brand: ${product.brand}`);
console.log(`Model: ${product.model}`);
console.log(`Power usage: ${product.powerUsage}W`);
}
}
In the displayProductDetails
function, we use our type predicates isBook
and isElectronics
to narrow down the product type. TypeScript now understands
the specific type of product
in each branch, allowing us to access properties
unique to each type without causing type errors.
What Can You Do Next ๐๐โ
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.