Advantages of Using Dependency Inversion Principle in TypeScript
Advatanges Of Using Dependency Inversion Principle
The Dependency Inversion Principle (DIP) is crucial to building scalable, maintainable, and robust software systems. Here are some of the concrete advantages of using DIP in your programming:
Decoupling of Code
By depending on abstractions rather than on concrete implementations, modules in the system are less interlinked. This reduces the risk that changes in one module will affect others.
interface IDatabase {
save(data: string): void;
}
class MySQLDatabase implements IDatabase {
save(data: string): void {
// logic to save data to a MySQL database
}
}
class MongoDBDatabase implements IDatabase {
save(data: string): void {
// logic to save data to a MongoDB database
}
}
class HighLevelModule {
private database: IDatabase;
constructor(database: IDatabase) {
this.database = database;
}
execute(data: string): void {
// high-level logic
this.database.save(data);
}
}
In the given example, the HighLevelModule
class is
decoupled from the specific database
implementations (MySQLDatabase
, MongoDBDatabase
). It depends only on
the IDatabase
abstraction, which means changes to specific database classes
don't affect the HighLevelModule
.
Ease of Modification and Extension
Because modules depend on abstractions, you can easily introduce new functionality or change existing functionality by creating new implementations of those abstractions.
If you need to add a new database
type, you can easily create a new class implementing IDatabase
and pass it
to HighLevelModule
. The HighLevelModule
class doesn't need to change,
demonstrating how DIP facilitates easy modification and extension.
Improved Testability
Testing becomes easier because you can provide mock implementations of your abstractions during the testing phase. This means you can test components in isolation, without needing to set up and coordinate complex real versions of all their dependencies.
DIP makes it easier to test HighLevelModule
. You
can create a mock implementation of IDatabase
and use it for
testing HighLevelModule
, ensuring that your tests are not dependent on a
live database.
Here's how you might create a mock implementation of IDatabase
for testing
purposes in TypeScript:
class MockDatabase implements IDatabase {
save(data: string): void {
console.log("Mocked save method called with data: " + data);
}
}
You would use this MockDatabase
class in your tests to make sure
the HighLevelModule
is functioning correctly without needing a real database.
For example:
let mockDatabase: IDatabase = new MockDatabase();
let highLevelModule: HighLevelModule = new HighLevelModule(mockDatabase);
// Now when you call the execute method, instead of actually writing to a database, it will simply log the operation.
highLevelModule.execute("Test Data");
In a real-world testing situation, you would likely use a testing framework like
Jest, and instead of simply logging the data in the mock, you would use the
framework's features to assert that the save
method was called correctly. This
was a simplified demonstration of the concept.
Code Reusability
Since higher-level and lower-level modules are not directly dependent on each other, it's easier to reuse these modules in different parts of the application or in different applications.
The HighLevelModule
class can work with any class
that implements IDatabase
, making it more reusable. You can use it with
different database types across different parts of your application or even
different applications.
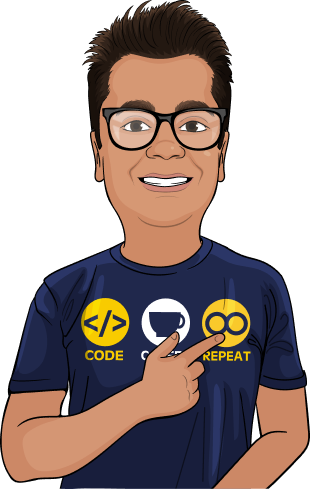
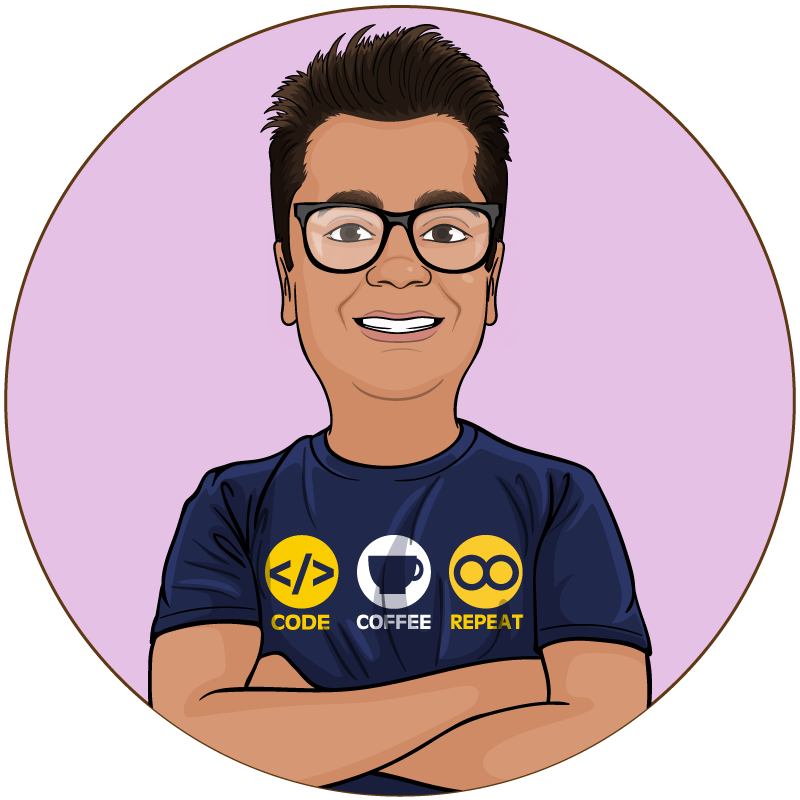
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSSystem Scalability
By structuring the codebase in such a way that lower-level details depend on high-level strategies, it becomes easier to scale up the system in the future. For example, you might start with a simple data store like a file system, and then later swap it out for a full database without changing the high-level code.
As your system grows and you decide to introduce a
new database system, DIP makes it easy. You would only need to add a new
implementation of IDatabase
and wouldn't need to touch
the HighLevelModule
.
To add a new database type to the system, we'd simply create a new class
that implements the IDatabase
interface.
Here's an example of how you might introduce PostgreSQL to your system:
class PostgreSQLDatabase implements IDatabase {
save(data: string): void {
// logic to save data to a PostgreSQL database
console.log("Data saved to PostgreSQL database: " + data);
}
}
Now, you can create a HighLevelModule
that uses this new PostgreSQLDatabase
:
let postgreSQLDatabase: IDatabase = new PostgreSQLDatabase();
let highLevelModule: HighLevelModule = new HighLevelModule(postgreSQLDatabase);
// Now use the module to execute some high-level function.
highLevelModule.execute("Some Data for PostgreSQL");
In this way, you've introduced a new database type to your system without having
to modify existing HighLevelModule
code, demonstrating the scalability offered
by the Dependency Inversion Principle.
More Understandable and Maintainable Code
As a result of reduced dependencies, code that follows the Dependency Inversion Principle is typically easier to understand and maintain. Each component can be understood in isolation, and the interactions between them are straightforward and based on clearly-defined interfaces.
Each module (HighLevelModule
, MySQLDatabase
, MongoDBDatabase
) can be
understood, developed, and maintained independently. This clear separation of
concerns makes the code easier to understand and maintain.
Remember, the SOLID principles (including DIP) are guidelines, not hard rules. It's essential to understand them and apply them appropriately, but also to recognize that there might be valid reasons in some circumstances to choose a different approach.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.