Deep Dive Into Open Closed Principle (OCP)
"The Open-Closed Principle states that "software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification." -- Robert C. Martin
The Principle
This principle dictates that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that you should be able to add new features or functionality to a system without altering its existing code. You can extend the behavior of the object, but you should not modify its source code.
Sure, I can explain the Open-Closed Principle (OCP) with a literal definition and then a more detailed explanation.
Literal Definition: The Open-Closed Principle states that "software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification." It's one of the five design principles of SOLID, a popular set of guidelines for object-oriented programming, and was introduced by Bertrand Meyer in 1988.
Detailed Explanation:
Open for extension: This means that the behavior of the software entity can be extended, that is, we should be able to add new features or behavior to it.
Closed for modification: This means that once the software entity is developed and it has been reviewed and tested, the code should not be touched (to correct the software behavior).
The idea behind the OCP is that it promotes greater flexibility in your code. When you need to add new behavior or features, instead of modifying existing code (and thus possibly introducing new bugs), you write new code that extends the old code.
How to Apply the Open-Closed Principle
Now, let's look at a TypeScript code example. Suppose you're building an online shopping application, and you want to implement a discount system. The discount will be different for different types of customers. Initially, we have only two types of customers, "Regular" and "Premium".
class Discount {
giveDiscount(customerType: string): number {
if (customerType === "Regular") {
return 10;
} else if (customerType === "Premium") {
return 20;
}
}
}
In this example, if we want to introduce a new type of customer, let's say a " Gold" customer with a different discount, we would have to modify the giveDiscount method in the Discount class:
class Discount {
giveDiscount(customer: Customer): number {
if (customer.type === "Regular") {
return 10;
} else if (customer.type === "Premium") {
return 20;
} else if (customer.type === "Gold") {
return 30;
}
return 0;
}
}
This violates the Open-Closed Principle because we're altering existing code to accommodate the new functionality (a new customer type). According to the Open-Closed Principle, we should be able to add this new functionality by adding new code (extending), not by changing existing code.
interface Customer {
giveDiscount(): number;
}
class RegularCustomer implements Customer {
giveDiscount(): number {
return 10;
}
}
class PremiumCustomer implements Customer {
giveDiscount(): number {
return 20;
}
}
class Discount {
giveDiscount(customer: Customer): number {
return customer.giveDiscount();
}
}
In the above example, we've defined a Customer
interface with a giveDiscount
method. RegularCustomer
and PremiumCustomer
classes implement the Customer
interface.
The Discount
class now remains closed for modification as it does not need to
be modified every time a new customer type is added. We've made it open for
extension because we can easily add more customer types by creating more classes
that implement the Customer
interface.
So, this is a brief explanation of the Open-Closed Principle (OCP) in SOLID principles with a TypeScript code example. This principle, when followed correctly, makes the code more robust, flexible, and less prone to bugs.
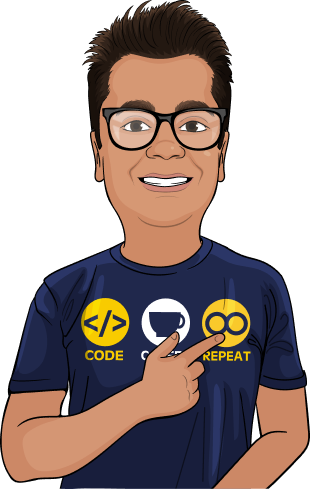
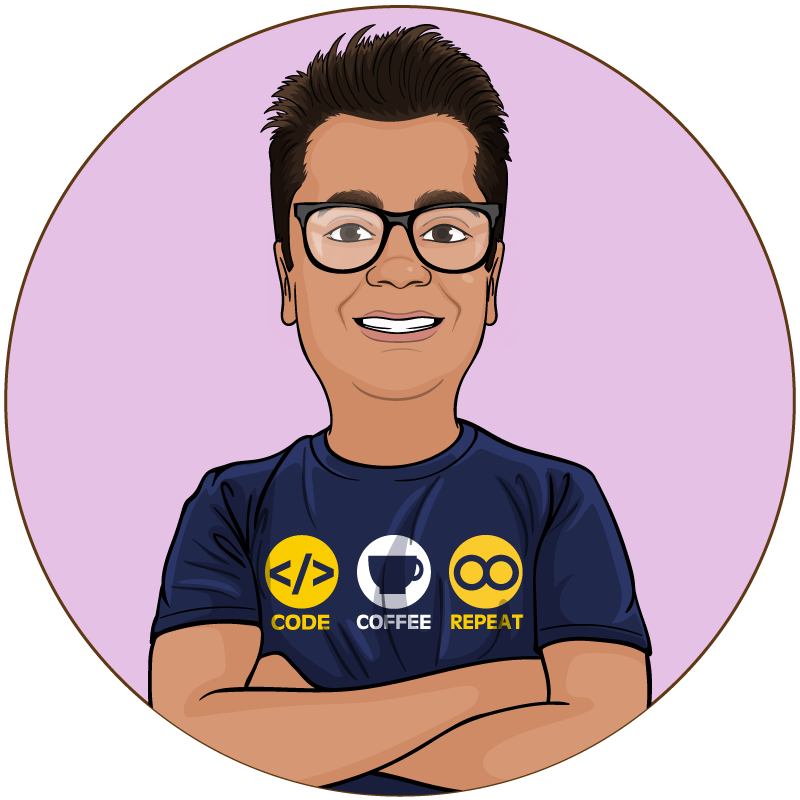
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.