Advantages of Using Interface Segregation Principle in TypeScript
Consider This Code
Let's use the code from our previous example to illustrate the advantages of ISP.
interface BlogService {
createPost(post: Post): void;
commentOnPost(comment: Comment): void;
sharePost(post: Post): void;
}
interface PostCreator {
createPost(post: Post): void;
}
interface CommentCreator {
commentOnPost(comment: Comment): void;
}
interface PostSharer {
sharePost(post: Post): void;
}
class Admin implements PostCreator, CommentCreator, PostSharer {
createPost(post: Post): void {
// Actual implementation
}
commentOnPost(comment: Comment): void {
// Actual implementation
}
sharePost(post: Post): void {
// Actual implementation
}
}
class RegularUser implements CommentCreator, PostSharer {
commentOnPost(comment: Comment): void {
// Actual implementation
}
sharePost(post: Post): void {
// Actual implementation
}
}
class ReadOnlyUser {
// Doesn't implement any of the interfaces because they can't perform any of these actions.
}
Using the Interface Segregation Principle (ISP) provides several concrete advantages in software development:
Improved maintainability
ISP promotes smaller, well-defined interfaces. Smaller interfaces are easier to implement, leading to less complex code. They also make the system easier to understand, change, and maintain, since each interface has a clear and narrow responsibility.
The interfaces PostCreator
, CommentCreator
,
and PostSharer
are smaller, each with only a single method. This makes it
easier to understand what each interface is responsible for and how they are
implemented by different classes.
Reduced impact of changes
Since classes depend only on the interfaces they use, changes in one interface won't affect classes that don't depend on it. This reduces the impact of changes in the codebase, making it easier to modify or extend functionality with less risk of creating bugs in unrelated areas.
If we need to make changes to the way posts
are shared, we only need to adjust the PostSharer
interface and the classes
that implement it. This does not affect the other interfaces or the classes
that implement them, thus reducing the potential impact of the change.
Increased flexibility and reusability
Small, well-defined interfaces tend to be more focused around specific roles, which can be more easily combined in different ways to create new functionality. This makes components more reusable and the overall system more flexible.
The specific roles defined by
these interfaces can be mixed and matched as needed. For example, we might
create a GuestUser
class that implements PostSharer
but not PostCreator
or CommentCreator
. This flexibility makes it easier to adjust the system to
new requirements.
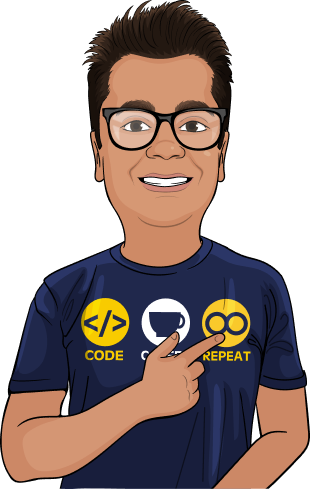
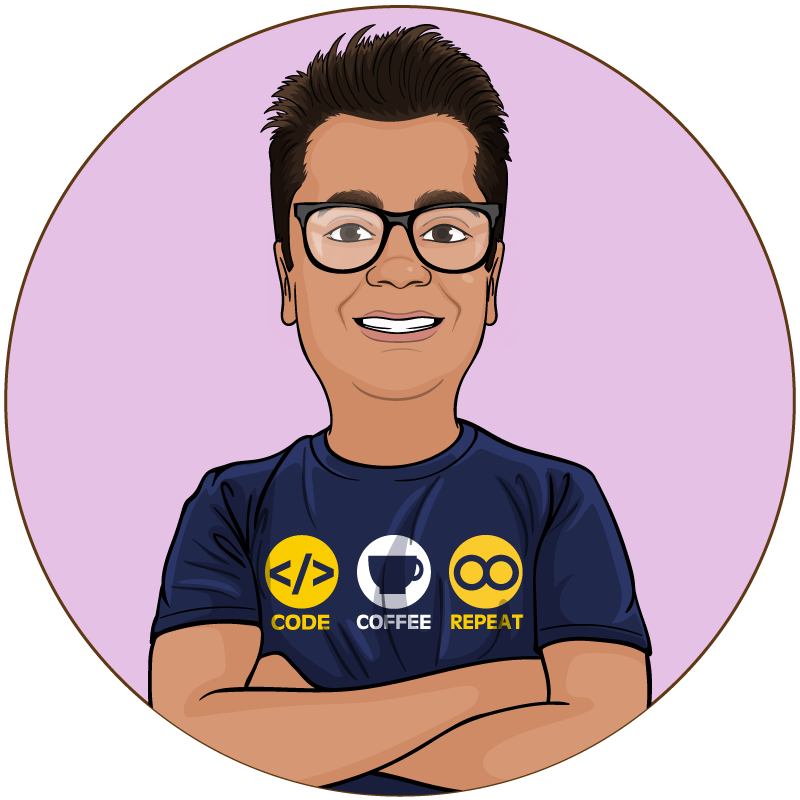
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSStronger encapsulation
By using interfaces to limit the exposure of classes to just the methods they need to know about, we strengthen the encapsulation of our system, leading to more robust and reliable code.
The Admin
, RegularUser
, and ReadOnlyUser
classes only expose the methods that they actually need to use. For instance,
the ReadOnlyUser
class doesn't need to implement any of the methods related
to creating or sharing content. This strengthens encapsulation by limiting
the exposure of these classes.
Better testing
Smaller interfaces with less responsibility can be easier to mock for unit tests. This can improve the quality of your testing and lead to less brittle tests.
The segregation of interfaces also simplifies testing.
For instance, when testing the post creation functionality, we only need to
focus on the PostCreator
interface. This makes it easier to write focused,
effective tests.
Better compliance with other principles
The ISP is related to other principles like the Single Responsibility Principle and the Dependency Inversion Principle. Following the ISP can often lead to better compliance with these other principles, leading to better designed, more modular code.
The segregated interfaces align
well with the Single Responsibility Principle (SRP) because each interface
has a single clear purpose. Additionally, they follow the Dependency
Inversion Principle (DIP) because higher-level modules (like
the Admin
, RegularUser
, and ReadOnlyUser
classes) depend on
abstractions (PostCreator
, CommentCreator
, PostSharer
interfaces) not
on concretions.
In summary, the Interface Segregation Principle leads to more maintainable and flexible code, which ultimately can reduce the cost and complexity of software projects.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.