Applications Of Adapter Pattern
Sure, here are a few real-world scenarios where you might use the Adapter pattern:
Interfacing with external systems or libraries
In many applications, you need to interact with external systems or libraries that have their own interfaces. If these interfaces don't match the interfaces your application expects, you could use the Adapter pattern to create a compatible interface.
In this diagram:
Application
represents your main application.Adapter
is the adapter class which presents a unified interface to your application.ExternalLibrary1
andExternalLibrary2
represent the external libraries or systems with different interfaces that your application needs to interact with. These could represent different payment gateways, different database systems, different third-party APIs, etc.
The Application
interacts with the Adapter
using a unified interface (in
this case, unifiedMethod()
). The Adapter
then interacts with
the ExternalLibrary1
and ExternalLibrary2
using their own specific
interfaces (specificMethod1()
and specificMethod2()
).
This is a simplified example, and the real implementation may involve more complexity depending on the number of methods, the differences between the external interfaces, and the needs of your application.
For example, you might be developing a payment processing system that needs to work with various payment gateways (like PayPal, Stripe, etc.). Each gateway has its own API with different methods and parameters. You can use the Adapter pattern to create a unified interface for your application, while each adapter handles the specifics of interacting with its respective payment gateway.
Legacy code integration
If your application has a lot of legacy code, it's often impractical or even impossible to refactor all of it to match a new interface. The Adapter pattern can help by acting as a bridge between the new code and the old code, allowing them to work together without the need for significant refactoring.
In this diagram:
NewApplication
represents your main application, which uses modern methods and systems.Adapter
is the adapter class which presents a unified interface to your application.LegacySystem
represents the old, legacy code or system that your application needs to interact with.
The NewApplication
interacts with the Adapter
using the modern
method (request()
). The Adapter
then interacts with the LegacySystem
using
the older method (oldRequest()
).
The Adapter pattern provides a bridge between the new application and the old system, allowing them to work together without significant refactoring of the legacy code.
For instance, you might have a legacy system that uses a different method naming convention or different data structures. Instead of refactoring the entire legacy system, which could be time-consuming and risky, you can introduce an adapter to translate between the new code and the old code.
Plug-in architecture
If you're developing an application that supports plug-ins or extensions, the Adapter pattern can help manage the variety of interfaces that different plug-ins might provide.
In this diagram:
Application
represents your main application that uses the plug-in architecture.Adapter
is the adapter class which presents a unified interface to your application.Plugin1
andPlugin2
represent the different plugins that your application needs to interact with. Each plugin provides its own unique features with potentially different interfaces.
The Application
interacts with the Adapter
using a unified
method (requestFeature()
). The Adapter
then interacts with Plugin1
and Plugin2
using their own specific interfaces (specificFeature1()
and specificFeature2()
).
By using the Adapter pattern, the application can handle a variety of plugins without having to accommodate all the differing interfaces directly, leading to cleaner and more maintainable code.
For instance, a text editor that supports plug-ins for code formatting, spell checking, and so forth might use the Adapter pattern. Each plug-in might provide its own unique interface, but the text editor could use adapters to standardize the way it interacts with the plug-ins.
In all these scenarios, the Adapter pattern provides a way to handle differences in interfaces without having to alter the rest of your application or the external/legacy system you're working with. It provides a flexible and clean way to ensure different parts of a system can communicate effectively, regardless of interface mismatches.
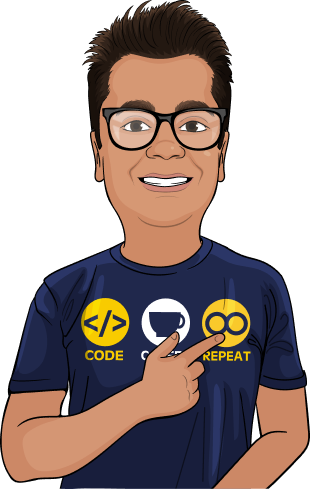
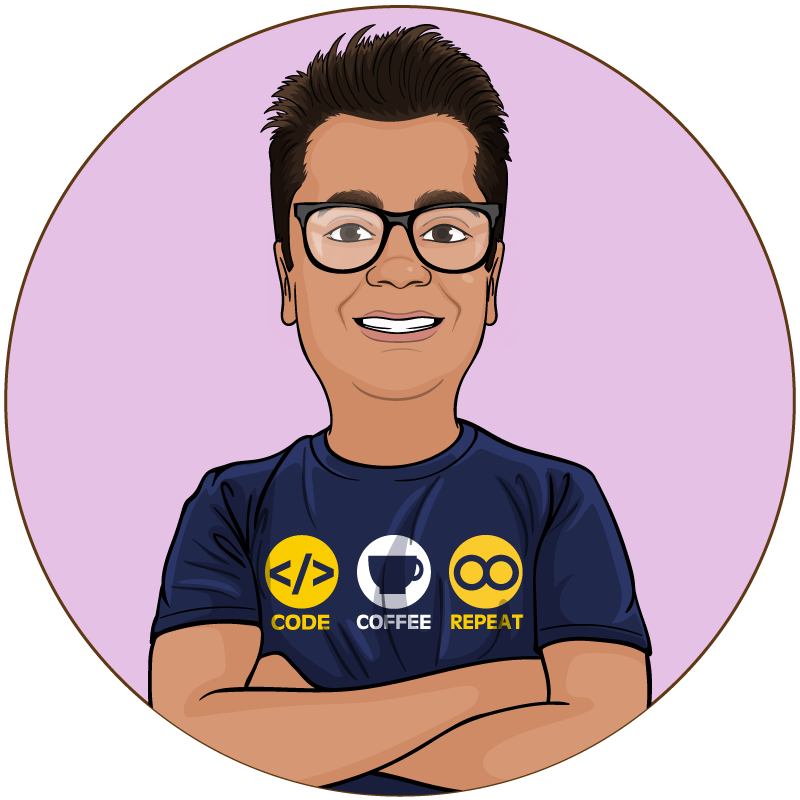
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.