Real World Application Of Bridge Pattern
Sure, I can provide several real-world scenarios where the Bridge pattern might be appropriate:
Graphic Libraries
Here's a class diagram created using Mermaid markdown for the Graphic Libraries example. I'll assume two types of shapes (Circle, Rectangle) and two types of renderers (OpenGL, DirectX).
In this diagram:
Shape
is the abstraction that client code interacts with.Circle
andRectangle
are concrete abstractions.Renderer
is the implementor interface.OpenGL
andDirectX
are concrete implementors.Shape
uses aRenderer
to carry out its operations.
This would allow you to add more shapes or rendering APIs in the future without affecting existing code.
Suppose you're creating a graphics library that should work with multiple rendering APIs (like OpenGL, DirectX, etc.). These APIs have their own way of drawing lines, curves, and other shapes. Now, a client wants to draw different shapes using your library but doesn't want to worry about the specifics of the rendering APIs.
Here, you can use the Bridge pattern to create an abstraction of the
shape (Shape
) and its implementation (Renderer
). The Shape
abstraction
can represent different types of shapes (like a circle, square, etc.), and
the Renderer
can represent different rendering APIs. This way, the client
code can work with the Shape
abstraction, and the specifics of rendering
are hidden in the Renderer
implementation.
Cross-platform Apps
Here's a class diagram created using Mermaid markdown for the Cross-platform Apps example. I'll assume two types of operations (CreateWindow, HandleEvent) and two types of platforms (Windows, Linux).
In this diagram:
Operation
is the abstraction that client code interacts with.CreateWindow
andHandleEvent
are concrete abstractions.Platform
is the implementor interface.Windows
andLinux
are concrete implementors.Operation
uses aPlatform
to carry out its operations.
This would allow you to add more operations or platforms in the future without affecting existing code.
If you're developing an application that needs to run on multiple platforms ( Windows, Linux, macOS, etc.), the Bridge pattern can be very useful. Here, the abstraction could represent high-level operations like creating a window, handling events, etc., and the implementations could represent platform-specific details.
With this approach, you can develop the high-level code without worrying about the platform specifics. When you need to support a new platform, you just need to add a new implementation.
Different Database Systems
Here's a class diagram created using Mermaid markdown for the Different Database Systems example. I'll assume two types of services (UserDataService, OrderDataService) and two types of databases (PostgreSQL, MongoDB).
In this diagram:
DataService
is the abstraction that client code interacts with.UserDataService
andOrderDataService
are concrete abstractions.Database
is the implementor interface.PostgreSQL
andMongoDB
are concrete implementors.DataService
uses aDatabase
to carry out its operations.
This would allow you to add more services or databases in the future without affecting existing code.
As we discussed in the previous examples, if your application needs to interact with different types of databases (like PostgreSQL, MongoDB, etc.), each having its own way of connecting, executing queries, and closing the connection, the Bridge pattern can be used to create a uniform interface for the client to interact with these databases while hiding the specifics.
In each of these scenarios, the Bridge pattern is beneficial because it allows the abstraction and implementation to vary independently. This makes the software more flexible to future changes and additions. It also improves the code's readability and maintainability by separating high-level logic from low-level details.
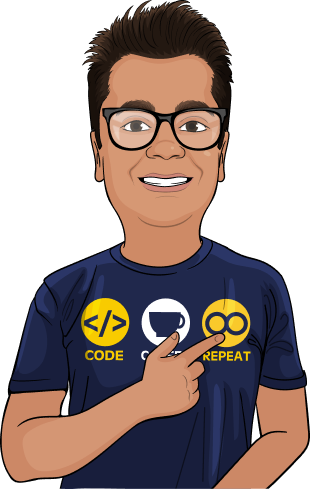
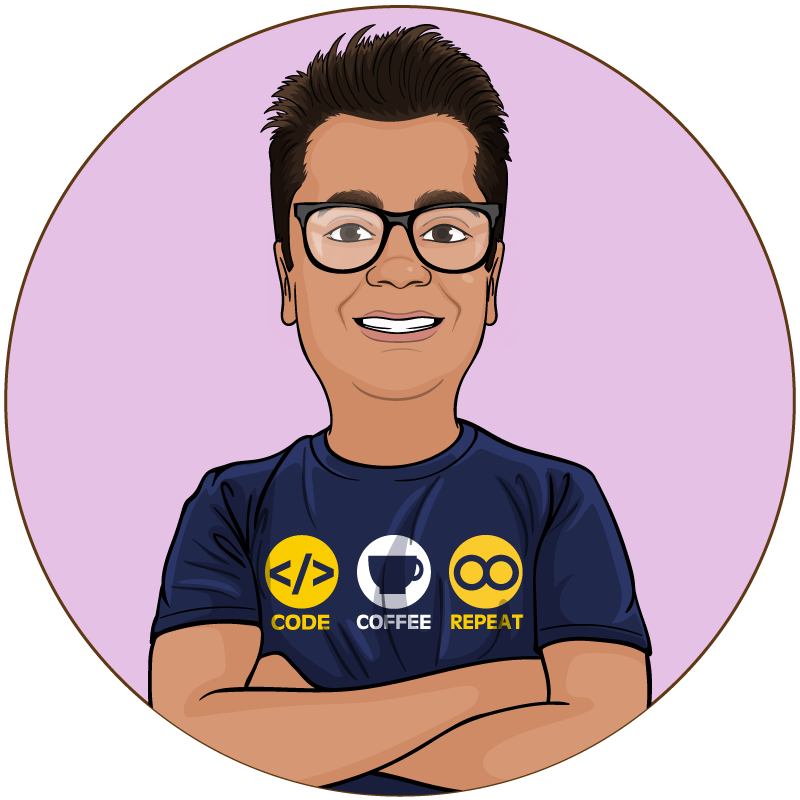
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.