Criticism or Caveats Of Decorator Pattern
Caveats and Criticism of Decorator Pattern
While the Decorator pattern has numerous benefits, there are also certain caveats and criticisms to be aware of. Here are a few:
Can result in many small objects that complicate the design and over-complicate the debugging
Decorators can lead to situations where functionality is scattered among many small objects. In a complex system with a lot of decorators, it can become difficult to see at a glance what the code is doing.
let server: ServerRequest = new AuthMiddleware(
new LoggingMiddleware(new ValidationMiddleware(new BaseServer()))
);
In this case, determining how a request is handled requires understanding all of these classes.
Interface compatibility
In statically typed languages, the Decorator pattern typically requires the decorator and the object it decorates to share a common interface. This can become an issue when the interface of the base object or component changes. When this happens, all the decorator classes would need to be updated to reflect the changes in the interface.
However, this issue of interface compatibility isn't as much of a concern in dynamically typed languages like JavaScript or TypeScript. TypeScript does have static types, but it also employs structural (or "duck") typing. In TypeScript, if an object has all the required properties with the correct types, it can be used wherever an object of a certain type is required, regardless of its actual class or interface.
Considering our server-side application example, here's how a problem could arise:
Suppose we decide to add a new method cancel()
to the ServerRequest
interface:
interface ServerRequest {
handle(request: any): void;
cancel(request: any): void;
}
Our BaseServer
class would be updated accordingly:
class BaseServer implements ServerRequest {
handle(request: any): void {
console.log("Handling request:", request);
}
cancel(request: any): void {
console.log("Cancelling request:", request);
}
}
The issue now is that our ServerRequestDecorator
class and its
subclasses (LoggingMiddleware
and AuthMiddleware
) do not yet have
the cancel()
method. As a result, TypeScript will throw an error saying
that ServerRequestDecorator
, LoggingMiddleware
, and AuthMiddleware
do not
correctly implement the ServerRequest
interface.
To fix this, we'd have to add the cancel()
method to ServerRequestDecorator
and all of its subclasses:
abstract class ServerRequestDecorator implements ServerRequest {
protected serverRequest: ServerRequest;
constructor(serverRequest: ServerRequest) {
this.serverRequest = serverRequest;
}
abstract handle(request: any): void;
// Added cancel method
abstract cancel(request: any): void;
}
In large systems with many decorators, it can be quite a hassle to ensure that all decorators stay in sync with the base interface. This is the interface compatibility problem you may face with the Decorator pattern. However, keep in mind that this problem tends to be more severe in languages with stricter type systems than TypeScript.
Adding new methods
The Decorator pattern is intended to add, remove, or change behavior dynamically to an object by using existing methods from an interface or abstract class. Adding new methods that do not exist in the base object's interface doesn't align with the core principle of the Decorator pattern.
The main problem with adding new methods in a decorator is that those methods are not part of the interface that the decorators and the decorated objects share. This can lead to two primary issues:
Type Compatibility: Since decorators and their decorated objects share an interface, adding new methods to a decorator would mean it no longer adheres to the original interface. This could lead to issues where the object's type is expected to conform to the original interface, and hence the newly added methods would not be accessible.
Object Identity: The Decorator pattern relies on object identity, meaning a decorated object should still be recognizable as an instance of its original type. If you add new methods in the decorator, you are effectively changing the identity of the decorated object. Code that relies on the identity of the object will fail.
To illustrate, consider the following:
interface ServerRequest {
handle(request: any): void;
}
class BaseServer implements ServerRequest {
handle(request: any): void {
console.log("Handling request:", request);
}
}
abstract class ServerRequestDecorator implements ServerRequest {
protected serverRequest: ServerRequest;
constructor(serverRequest: ServerRequest) {
this.serverRequest = serverRequest;
}
abstract handle(request: any): void;
}
class AuthMiddleware extends ServerRequestDecorator {
handle(request: any): void {
if (request.isAuthenticated) {
console.log("Request is authenticated");
this.serverRequest.handle(request);
} else {
console.log("Unauthorized request");
}
}
// New method
authenticate(request: any): void {
console.log("Authenticating request:", request);
}
}
Now, if you have an AuthMiddleware
object, you can call authenticate()
on
it:
let server: ServerRequest = new BaseServer();
server = new AuthMiddleware(server);
(server as AuthMiddleware).authenticate({}); // This works
But if you're treating server
as an instance of ServerRequest
, you can't
call authenticate()
, because ServerRequest
doesn't have that method:
let server: ServerRequest = new AuthMiddleware(new BaseServer());
server.authenticate({}); // Type error: Property 'authenticate' does not exist on type 'ServerRequest'.
This defeats the purpose of the Decorator pattern, which is to treat decorated objects just like undecorated ones. If you need to add new methods, you may want to consider other patterns such as the Composite pattern.
Can be overkill for simple additions
If the functionality you're adding is very simple, it might be easier to just add it directly to the class rather than creating a new decorator. The Decorator pattern is most valuable when you need to add complex or variable functionality.
Unexpected behavior with other code
One of the potential downsides of the Decorator pattern is that it can cause unexpected behavior with other parts of your code that rely on the identity of objects. This means that when the identity of an object changes (as it does when it is wrapped with a decorator), it may not behave as expected in other parts of your system.
Consider the following scenario:
You have code elsewhere in your system that relies on object identity, such as an equality check (
===
). Let's imagine you have a "registry" ofServerRequest
objects:const registry = new Set<ServerRequest>();
let server: ServerRequest = new BaseServer();
registry.add(server); // Register this serverNow you decide to decorate your
ServerRequest
withLoggingMiddleware
:server = new LoggingMiddleware(server);
If you now perform an equality check against the registry, the decorated server is not recognized as being the same object:
if (registry.has(server)) {
// This will be false
console.log("Server found in registry.");
} else {
console.log("Server not found in registry."); // This will be printed
}Even though you've only added behavior to
server
, the fact that you've wrapped it in a decorator means it's now a different object as far as the===
operator (and methods likeSet.prototype.has
) is concerned.
This can be a particularly insidious problem because it's not immediately obvious why the code is not working as expected. When using the Decorator pattern, it's important to keep in mind that the identity of the decorated object is not preserved, and this can cause unexpected issues with equality checks and other code that relies on object identity.
If you need to preserve identity, you may need to find a different solution, such as modifying the object directly or using a different pattern.
Ordering of decorators is important
The order in which decorators are applied is important because it determines the order in which the decorators' behaviors are applied, which can significantly impact the behavior of the decorated object.
In our server-side application example, let's say we
have AuthMiddleware
, LoggingMiddleware
, and ValidationMiddleware
. The
order of applying these decorators will directly impact how requests are
handled:
let server: ServerRequest = new BaseServer();
server = new AuthMiddleware(server);
server = new LoggingMiddleware(server);
server = new ValidationMiddleware(server);
In this case, when a request is passed to server.handle(request)
, the
following happens:
ValidationMiddleware
checks whether the request data is valid.- If the request is valid, it's passed to
LoggingMiddleware
, which logs the request. - The request is then passed to
AuthMiddleware
, which checks whether the request is authenticated. - If the request is authenticated, it's finally passed to
BaseServer
to be handled.
Now, if we change the order in which we apply these decorators:
let server: ServerRequest = new BaseServer();
server = new ValidationMiddleware(server);
server = new AuthMiddleware(server);
server = new LoggingMiddleware(server);
The following happens when server.handle(request)
is called:
LoggingMiddleware
logs the request.- The request is passed to
AuthMiddleware
, which checks whether the request is authenticated. - If the request is authenticated, it's passed to
ValidationMiddleware
, which checks whether the request data is valid. - If the request is valid, it's finally passed to
BaseServer
to be handled.
As you can see, changing the order of decorators changes the order in which operations are performed on the request. In the first example, we log the request only after it has been validated, but before authentication. In the second example, we log the request before it has been authenticated or validated.
Hence, it's crucial to understand the business logic and the consequences of the order in which decorators are applied, as it directly affects the system behavior.
Before deciding to use the Decorator pattern, developers should consider these potential downsides and ensure they won't cause issues for their specific use case. The Decorator pattern is a powerful tool, but like any tool, it's not always the best choice for every situation.
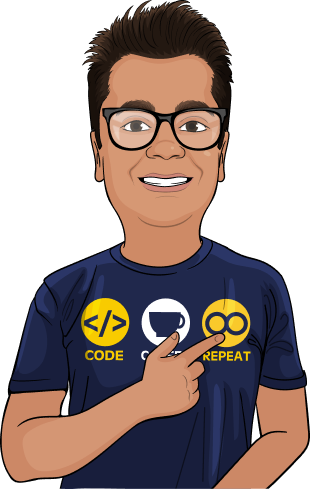
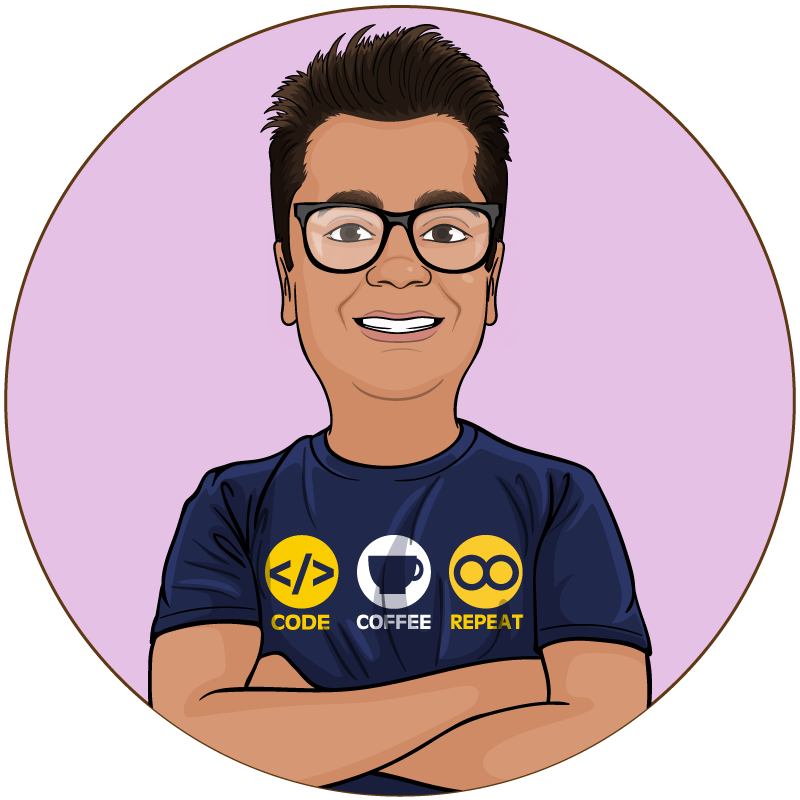
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.