Applications Of Decorator Pattern
Applications Of The Decorator Pattern
The Decorator pattern is particularly useful when you need to dynamically add or modify behavior in an object without affecting other objects of the same class. This often arises in scenarios where extending functionality through inheritance is not ideal because it results in an explosion of subclasses to support every possible combination of behaviors. Here are a few real-world examples:
GUI Toolkits
Here's a simplified class diagram of how a GUI toolkit might use the Decorator pattern using Mermaid markdown:
In the above diagram:
Component
is an abstract component defining the interface for objects that can have responsibilities added to them dynamically.ConcreteComponent
is the actual object to which additional responsibilities can be added.Decorator
maintains a reference to a Component object and defines an interface that conforms to Component's interface. It acts as the base decorator.ConcreteDecoratorA
andConcreteDecoratorB
are the decorators that add responsibilities to the component. These are specific decorators with added behaviors. For a GUI toolkit, they might represent individual features like a scrollbar (ConcreteDecoratorA
) or a border (ConcreteDecoratorB
). Each of these decorators can alter the behavior of theConcreteComponent
, either before and/or after forwarding theoperation()
call.
This diagram is generic and not specific to GUI Toolkits. However, it helps in
understanding the implementation of the Decorator pattern which can then be
contextualized to GUI Toolkits. For example, ConcreteComponent
could be
a Window
object, and ConcreteDecoratorA
and ConcreteDecoratorB
could
be ScrollbarDecorator
and BorderDecorator
, respectively, that add a
scrollbar or border to the window.
GUI (Graphical User Interface) toolkits often use the
Decorator pattern to add features to visual components. For instance, you
might have a base Window
object, and you want to be able to add features
like borders, scrollbars, or menus. You could create a separate subclass for
each combination (
e.g., WindowWithScrollbar
, WindowWithMenu
, WindowWithScrollbarAndMenu
,
etc.), but the number of subclasses would quickly become unmanageable.
Instead, you can use decorators to add each feature individually to
a Window
object. This gives you the flexibility to add any combination of
features to any Window
without needing to create a huge number of
subclasses.
Java I/O Classes
Here's a simplified class diagram of how Java I/O classes use the Decorator pattern, depicted using Mermaid markdown:
In the above diagram:
InputStream
is the abstract component, providing an interface for input streams of bytes.FileInputStream
is a concrete component, getting input bytes from a file in a file system.FilterInputStream
is the base decorator, containing anotherInputStream
, which it uses to perform its operations.BufferedInputStream
andDataInputStream
are concrete decorators, providing additional functionality like buffered reading and reading Java primitive data types.
In this scenario, the FilterInputStream
maintains a reference to
an InputStream
and defines an interface that conforms to InputStream's
interface. Concrete decorators like BufferedInputStream
and DataInputStream
add specific input stream manipulations like buffered reading or reading
primitive data types. They modify the behavior of InputStream
(
specifically, FileInputStream
), either before and/or after forwarding
the read()
call.
This diagram is a very high-level abstraction. Real Java I/O classes may have additional complexities and functionalities.
The Java I/O classes use the Decorator pattern to
provide a flexible way of adding functionality to reading and writing streams
of data. The base classes InputStream
and OutputStream
provide basic data
read/write capabilities. Decorators such
as BufferedInputStream
, DataInputStream
, LineNumberInputStream
, etc.
add additional features like buffering data for efficient reading, reading
primitive data types, and keeping track of line numbers. By using decorators,
you can choose exactly which features you need and apply them in any
combination, without cluttering your code with a large number of subclasses.
Middleware in Web Development
Here's a simplified class diagram of how Middleware in web development could use the Decorator pattern, depicted using Mermaid markdown:
In the above diagram:
Middleware
is the abstract component defining the interface for objects that can have responsibilities added to them dynamically.BasicHandler
is the concrete component that handles requests.MiddlewareDecorator
is the base decorator that maintains a reference to aMiddleware
object and defines an interface that conforms toMiddleware's
interface.AuthMiddleware
,LoggingMiddleware
, andValidationMiddleware
are concrete decorators that add responsibilities to theMiddleware
. They represent middleware that perform authentication, logging, and validation, respectively. Each of these decorators can modify the behavior of thehandleRequest
operation, either before and/or after forwarding the call.
Middleware in web development is often implemented using the Decorator pattern. Middleware is software that provides services to applications outside of what's offered by the operating system. Features like caching, logging, validation, authentication, etc., can all be implemented as middleware and added to a web application as needed. Using the Decorator pattern allows these features to be added in any combination and order, giving developers the flexibility to customize how requests are processed.
In all these cases, the Decorator pattern allows developers to add or change behavior dynamically and flexibly, without needing to create a large number of subclasses or change the base class. It gives developers the ability to choose exactly what functionality they need and apply it in the way that best suits their specific use case.
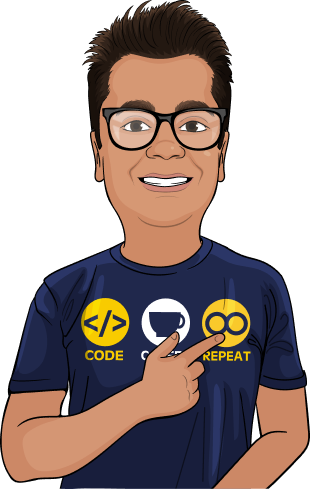
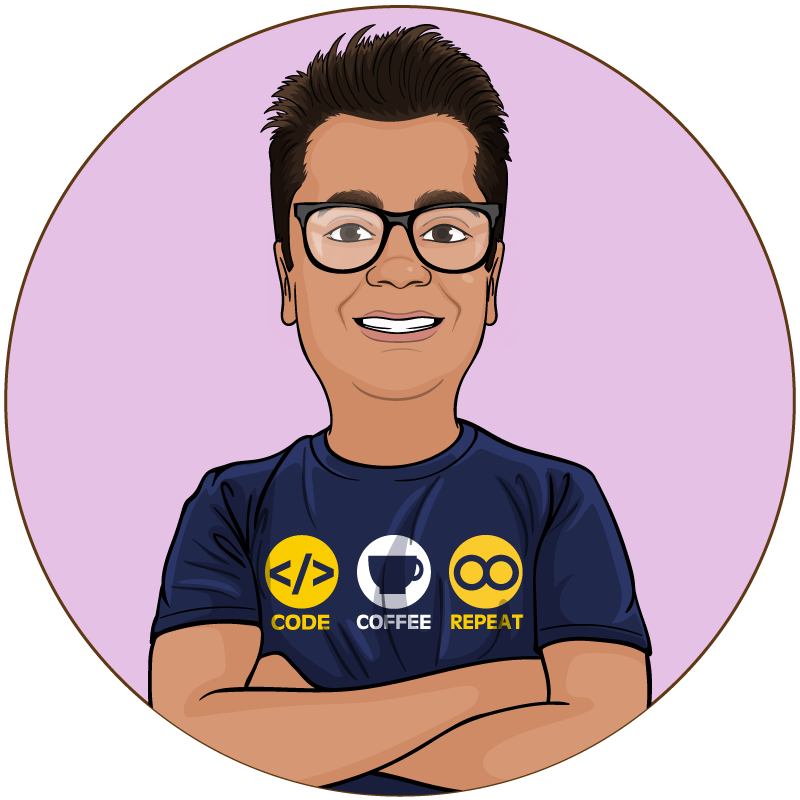
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.