Criticism Of Facade Pattern
While the Facade pattern can provide many benefits, like simplifying interfaces and reducing complexity, it's not without its drawbacks or criticisms.
Here are some points to consider when deciding whether to use the Facade pattern:
Over-abstraction
Facades can sometimes lead to an unnecessary level of abstraction. If the underlying subsystem is relatively simple and doesn't require a simplified interface, introducing a facade might add complexity rather than reducing it.
let's take a look at how the Facade pattern might lead to over-abstraction
using the HomeTheaterFacade
example.
In the given example, the HomeTheaterFacade
provides a simplified interface
for operating a home theater system. It bundles up the operations of turning on
the amplifier, setting the volume, turning on the DVD player, setting the input
for the projector, dimming the lights, and playing the movie into a single
method watchMovie
.
class HomeTheaterFacade {
/* ... */
watchMovie(movie: string) {
console.log("Get ready to watch a movie...");
this.lights.dim(10);
this.amplifier.turnOn();
this.amplifier.setVolume(5);
this.dvdPlayer.turnOn();
this.projector.setInput(this.dvdPlayer);
this.dvdPlayer.play(movie);
}
}
This simplifies the client's interaction with the home theater system, but it also abstracts away a lot of details about the subsystems and their operation. While this level of abstraction is beneficial when we simply want to watch a movie, it can be limiting in other scenarios.
For instance, what if you want to use the amplifier with a different input, like
a music player, rather than the DVD player? Or what if you want to use the
projector without dimming the lights? In these cases, the abstraction provided
by the HomeTheaterFacade
is not only unhelpful but potentially restrictive.
You would have to bypass the facade and interact directly with the subsystems,
which defeats the purpose of the facade.
This is what is meant by over-abstraction. By trying to simplify the interface, you may end up abstracting away functionality that clients might need. In some scenarios, the abstraction provided by the facade is not necessary and might make the system harder to use rather than easier. This is why it's important to carefully consider when to use the Facade pattern. It's most beneficial when the subsystem is complex and a simpler interface can help the client use the system.
Limited Flexibility
By using a Facade, you're limiting your access to the full functionality and flexibility of the subsystem. If clients need to perform more complex operations not covered by the facade, they will have to bypass the facade, which can lead to a mix of high-level and low-level calls in the client code.
The Facade pattern's primary goal is to provide a simplified interface to a complex subsystem, encapsulating the details and hiding the complexities of that subsystem. This approach can limit flexibility in some scenarios.
Let's again consider our HomeTheaterFacade
example. The watchMovie
function
is specifically designed to set up a movie-watching experience. It dims the
lights, turns on the amplifier, sets the volume, powers up the DVD player,
configures the projector to use the DVD player as its input, and then plays the
movie.
class HomeTheaterFacade {
/* ... */
watchMovie(movie: string) {
console.log("Get ready to watch a movie...");
this.lights.dim(10);
this.amplifier.turnOn();
this.amplifier.setVolume(5);
this.dvdPlayer.turnOn();
this.projector.setInput(this.dvdPlayer);
this.dvdPlayer.play(movie);
}
}
This method provides a simplified way to perform all these steps, but it offers
no flexibility. Suppose you wanted to play a video game on the projector instead
of watching a movie, or you wanted to listen to an audio CD on the amplifier
without turning on the projector and dimming the lights. The watchMovie
method
can't handle these use cases.
In order to accomplish these tasks, you would need to bypass the facade and
directly interact with the subsystem
classes (Amplifier
, DvdPlayer
, Projector
, Lights
), which defeats the
purpose of having the facade in the first place.
So while the Facade pattern simplifies interaction with complex subsystems by providing a high-level interface, it may limit the flexibility to perform more specialized or complex tasks that require direct interaction with the subsystem components. Therefore, when using the Facade pattern, one should carefully consider the trade-off between simplicity and flexibility.
Hiding Useful Information
While encapsulating the details of complex subsystems is often useful, in some cases, it might hide information that could be beneficial for the client code. Developers using the facade may be unaware of the specifics of subsystem behavior which might be important in certain scenarios.
The Facade pattern's primary role is to provide a simplified interface to complex subsystems. While this can make the subsystems easier to use, it can also result in the loss of potentially useful information or functionality that's available in the subsystems but not exposed through the facade.
Let's use our HomeTheaterFacade
example to explore this concept.
class HomeTheaterFacade {
/* ... */
watchMovie(movie: string) {
console.log("Get ready to watch a movie...");
this.lights.dim(10);
this.amplifier.turnOn();
this.amplifier.setVolume(5);
this.dvdPlayer.turnOn();
this.projector.setInput(this.dvdPlayer);
this.dvdPlayer.play(movie);
}
}
The watchMovie
method simplifies the process of watching a movie. But what if
one of the subsystems has additional features or settings that the client might
want to utilize?
For example, the Amplifier
class might have a method to adjust the audio
balance, the DvdPlayer
could have settings for language or subtitle
preferences, or the Projector
might have different aspect ratio settings.
These features are all hidden by the HomeTheaterFacade
, so clients can't
access them without bypassing the facade and directly interacting with the
subsystems.
In this way, while the facade makes the subsystem easier to use for the most common tasks, it can hide potentially useful information or functionality. When using the Facade pattern, it's important to carefully consider which functionalities to expose in the facade's interface to strike a balance between simplicity and functionality.
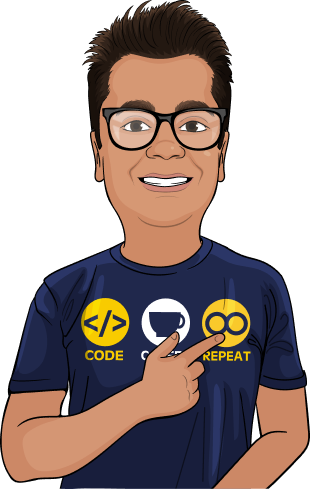
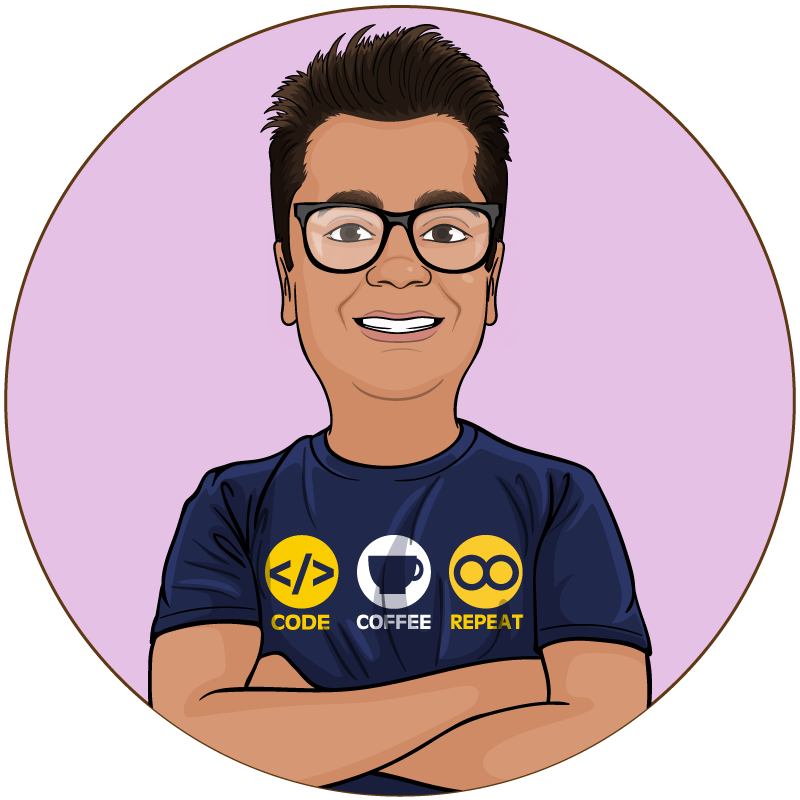
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.