Applications Of Facade Pattern
Applications Of Facade Pattern
Certainly, here are some real-world scenarios where the Facade Pattern could be beneficial:
E-commerce Systems
In this diagram:
OrderFacade
is the facade class. It provides a unified interface (placeOrder
) to the client.InventorySystem
,PaymentSystem
, andShippingSystem
are subsystem classes. Each subsystem might have a complex interface with various methods.The
OrderFacade
interacts with all these subsystems whenplaceOrder
method is called. The facade is responsible for calling methods likecheckStock
,reduceStock
,authorizePayment
,chargePayment
,scheduleDelivery
in the correct sequence.
Please note that this is a very simplified representation. In real-world applications, these subsystems would be much more complex and could have many more methods. Also, there could be more subsystems involved in the process.
An e-commerce system has multiple subsystems like
inventory management, user authentication, payment processing, shipping, etc.
Each of these subsystems can be complex in their own right. A Facade could
simplify client interactions with these subsystems. For example, when a
customer places an order, the facade could handle interactions with
inventory, payment, and shipping subsystems, providing a single placeOrder
method that abstracts away these complexities. This reduces the complexity
for clients (like a web interface or mobile app), simplifies code
maintenance, and provides flexibility to change underlying subsystems without
affecting clients.
Banking Systems
In a banking system, operations like fund transfers may
involve several steps like authentication, balance checks, transaction
initiation, transaction completion, and receipts generation. These operations
might be distributed across different subsystems. A FundTransfer
facade
could simplify this process by providing a single transferFunds
method,
abstracting away the complexities of interacting with multiple subsystems.
This would simplify the client code (which could be an online banking portal
or a banking app) and make it easier to maintain and adapt.
In this diagram:
FundTransferFacade
is the facade class. It provides a unified interface (transferFunds
) to the client.AuthenticationSystem
,BalanceSystem
,TransactionSystem
, andReceiptSystem
are subsystem classes. Each subsystem might have a complex interface with various methods.The
FundTransferFacade
interacts with all these subsystems when thetransferFunds
method is called. The facade is responsible for calling methods likeauthenticateUser
,checkBalance
,deductAmount
,initiateTransaction
,completeTransaction
,generateReceipt
in the correct sequence.
Game Engines
Game engines have to deal with multiple subsystems like rendering, physics, audio, AI, input handling, etc. A facade can be used to provide a simplified API for game developers, abstracting away the complexities of the underlying engine subsystems. The game developers can focus on the game logic and don't have to worry about the intricacies of the game engine subsystems.
Absolutely, let's construct a class diagram for a simplified Game Engine using the Facade pattern:
In this diagram:
GameEngineFacade
is the facade class. It provides a unified interface (initialize
,render
,update
) to the client, in this case, the game developers.RenderingSystem
,PhysicsSystem
,AudioSystem
,AISystem
, andInputSystem
are subsystem classes. Each subsystem might have a complex interface with various methods.The
GameEngineFacade
interacts with all these subsystems when theinitialize
,render
, andupdate
methods are called. The facade is responsible for calling methods likeprepareScene
,drawScene
,updatePhysics
,prepareAudio
,playAudio
,updateAI
,processInput
in the correct sequence.
Please note that this is a simplified representation. In real-world game engines, these subsystems would be far more complex and could have many more methods. Also, there could be more subsystems involved in the process.
In all these scenarios, the common factor is that the Facade Pattern is used to simplify interactions with complex subsystems. The facade provides a simple, unified interface to clients, hiding the complexities and details of the subsystems, making the system easier to use and maintain, and providing flexibility to evolve subsystems independently.
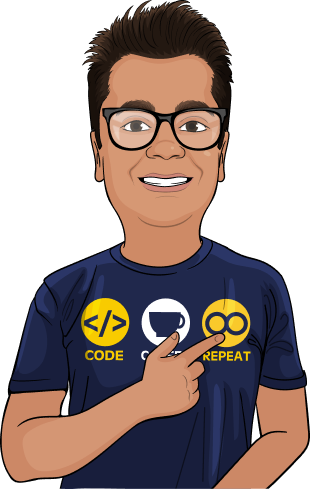
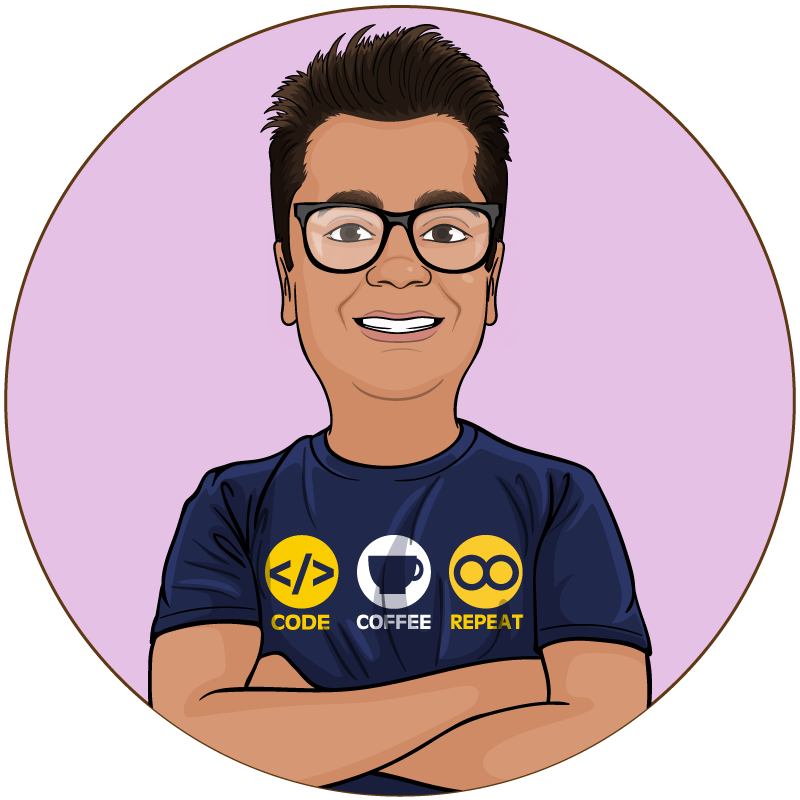
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.