Use Cases For Abstract Factory Pattern
What To Consider
While the decision to use the Abstract Factory pattern (or any design pattern for that matter) is highly context-dependent, here are a few situations where the Abstract Factory pattern might be a good fit:
Managing Related Product Families
The Abstract Factory pattern is ideal when you have families of products that are closely related, and you want to ensure that clients use products from only one family at a time. For instance, in our GUI example, we have a "Mobile" family and a "Desktop" family of products. It wouldn't make sense to mix a "MobileButton" with a " DesktopMenu".
Ensuring Compatibility Within Product Families
If you need to ensure that all products in a family are compatible with each other and that clients are prevented from using incompatible products together, the Abstract Factory can be useful.
Abstraction of Product Creation
Abstract Factory pattern abstracts away the creation details of complex objects from the client code. This makes the client code more flexible and easier to maintain.
You Want to Change Product Sets Dynamically
If your application needs to support multiple families of products that can be swapped in and out easily (like changing from a Mobile GUI to a Desktop GUI), the Abstract Factory pattern can help.
You Want to Isolate Concrete Classes from Clients
Abstract Factory pattern can be a good fit when your system should be independent of how its products are created, composed and represented. The client code only interacts with the abstract interfaces, promoting decoupling and making the code more extendable and maintainable.
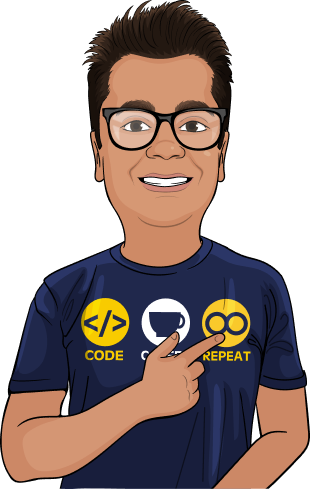
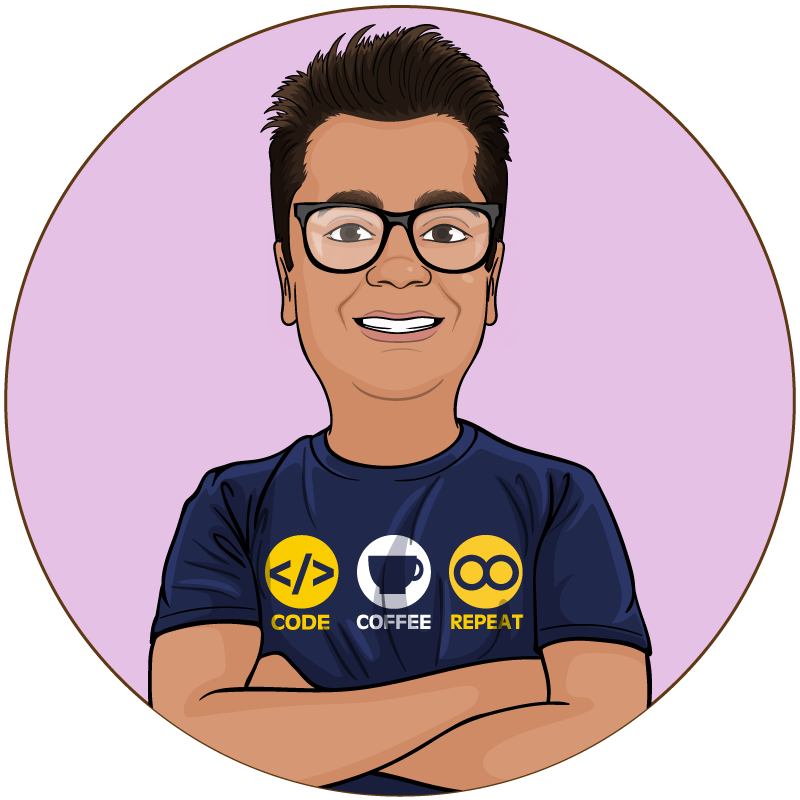
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSUse Cases
The Abstract Factory pattern is widely used in software development, especially in cases where a system must be independent from the way the products it works with are created. Here are some real-world use cases:
GUI Libraries
As we've seen in previous examples, GUI libraries often use the Abstract Factory pattern to provide support for different look-and-feels or windowing systems. For example, an application can display its UI as a native Windows application, a web application, or a mobile application, and the Abstract Factory pattern makes it easy to switch between these at runtime.
Databases
The Abstract Factory pattern can be used to handle the creation of database connections and queries for different types of databases. For instance, you might have an abstract factory for creating database connections, with different concrete factories for MySQL, PostgreSQL, Oracle, etc. The abstract factory would create the appropriate connection and query objects based on the database type.
Cross-Platform Development
The Abstract Factory pattern is often used in applications that need to run on multiple platforms. For example, a game might use different sets of objects (sounds, graphics, input handlers, etc.) depending on whether it's running on Windows, Mac, or a console. The Abstract Factory pattern makes it easy to swap out these object sets based on the current platform.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.