Introduction To Creational Design Patterns
What Are Creational Design Patterns?
Creational design patterns in object-oriented programming deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. The basic form of object creation could result in design problems or add complexity to the design. Creational design patterns solve this problem by somehow controlling this object creation process.
Here are the five types of creational design patterns:
Singleton
This pattern restricts the instantiation of a class and ensures that only one instance of the class exists in the java virtual machine. It provides a global point of access to the object. This is useful when exactly one object is needed to coordinate actions across the system.
Factory Method
This pattern provides a way to delegate the instantiation logic to child classes. The factory method design pattern defers the case of object creation to the subclasses implementing the factory method.
Abstract Factory
This pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. It encapsulates a group of factories with a common theme without specifying their concrete classes.
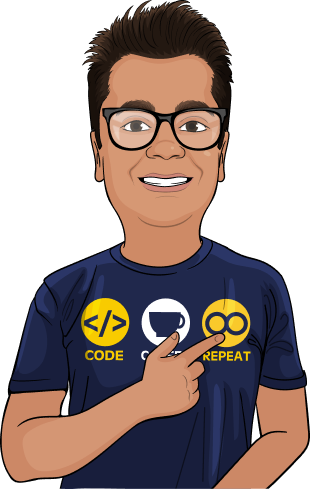
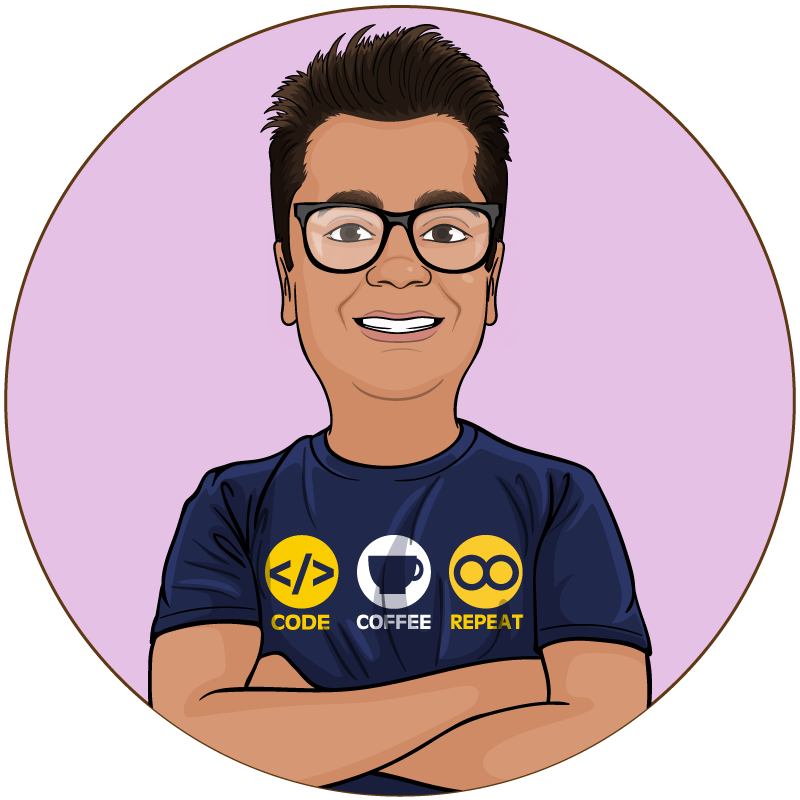
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSBuilder
This pattern builds a complex object using simple objects and a step by step approach. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object. It provides clear separation and a unique layer for the construction and representation of a complex object.
Prototype
This pattern is used when the type of objects to create is determined by a prototypical instance, which is cloned to produce new objects. This pattern involves implementing a prototype interface which tells to create a clone of the current object.
In all these cases, the system is more flexible, adaptable, and reusable because it is not hard-wired to the application's classes but can work with any classes that meet the required interface. The creational patterns all provide some way to improve system modularity by encapsulating these responsibilities.
Why Do We Need Creational Design Patterns?
Creational design patterns are needed because they abstract the process of object creation and help make a system independent of how its objects are created, composed, and represented. In simpler terms, they provide a way to encapsulate knowledge about which classes the system uses.
There are several reasons why we might want to use creational design patterns:
Flexibility and Reusability
Creational patterns make the system more flexible in terms of the what, who, and when of object creation. They allow systems to be composed of interchangeable parts to which you can add new parts with little or no modification. This makes it easier to upgrade the system or to modify it to provide new functionality.
Abstraction and Decoupling
Creational patterns encapsulate the knowledge about which classes the system uses and how objects of these classes are created and put together. They provide a way to decouple a client and the concrete classes it instantiates. Decoupling is beneficial because it leads to a more resilient system that's less likely to break when changes are made.
Control over Object Creation Process
Some creational design patterns allow finer control over the object creation process, allowing you to put steps in place to handle variations or specific cases of object creation, which can't be achieved with straightforward constructors.
Handling Complexity
Object creation can often become complex when it involves a lot of configuration or composition of other objects. Creational patterns like Builder or Factory Method can help manage this complexity by organizing and simplifying the creation process.
Overall, creational design patterns help in structuring code to manage and control how objects are created, which is essential for building robust and flexible software systems.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.