Where And When To Use Singleton
The Singleton pattern can be very useful in specific scenarios where there needs to be a single instance of a class throughout the lifetime of an application.
What To Consider
As a programmer, you should consider using the Singleton pattern when you have a situation where:
- There needs to be exactly one instance of a class, and it must be accessible to clients from a well-known access point.
- The single instance should be extensible by subclassing, and clients should be able to use an extended instance without modifying their code.
- Control of simultaneous access to the shared resource must be ensured.
Singleton Applications
Here are a few scenarios where the Singleton pattern is typically used: Absolutely, I'll provide more detail on each scenario:
Logger
Consider a large web application where different components, modules, or services are generating log data - like user actions, system events, or error messages. It's important to have a consistent and centralized way of logging this information. A singleton Logger class could provide this. It can ensure that all parts of the application are writing to the same log file in a consistent format. This can greatly simplify log analysis and debugging. If there were multiple instances of a logger, you could end up with logs scattered across different files or in different formats, which would make analysis much harder.
Configuration Data
In a complex application, you might have configuration settings that control various aspects of the application's behavior. These settings might be stored in a configuration file, a database, or a remote server. To avoid the overhead of fetching these settings every time they're needed, you can use a singleton Configuration class to load the settings when the application starts, and then provide access to them for the rest of the application. This can significantly improve performance, and it also ensures that all parts of the application are using the same settings.
Access to Shared Resources
Some resources are naturally singletons. For example, a database connection is a resource that can be shared among various parts of an application. Opening and maintaining multiple database connections can be resource-intensive and can lead to errors due to conflicting updates. A singleton DatabaseConnection class can ensure that all parts of the application are using the same connection, which can improve efficiency and consistency.
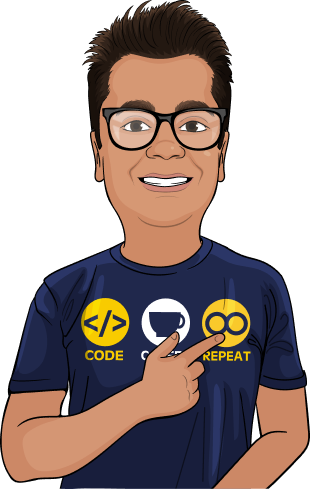
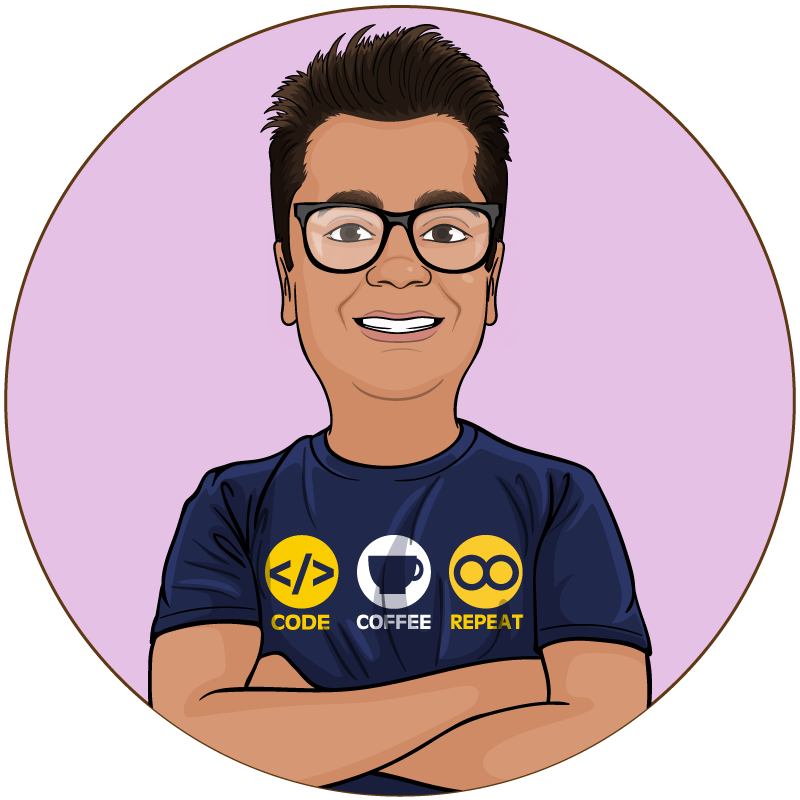
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSService Proxies
If your application communicates with a remote server - for example, to fetch data, submit updates, or perform other operations - it's often more efficient to have a single ServiceProxy class manage all the communication. This can avoid the overhead of establishing a new network connection for each operation, and it can also handle things like queuing operations if the server is busy, retrying operations if the server is unavailable, and so forth. If there were multiple service proxies, they might end up duplicating these efforts and causing unnecessary network traffic.
Caching
Caching is a common technique to improve performance by storing the results of expensive operations, so that if the same operation is requested again, the result can be returned from the cache instead of repeating the operation. A singleton Cache class can ensure that all parts of the application are using the same cache, so that they can share results and avoid duplicating effort. If there were multiple caches, an operation that had been cached by one part of the application might be needlessly repeated by another part of the application because it didn't have access to the same cache.
These are all simplifications of real-world scenarios, but they should give you a good idea of when and why you might want to use a singleton.
Remember, even though the Singleton pattern can be very useful, it should be used sparingly as it introduces a global state into the application and can lead to problems if not used carefully.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.