Abstract Factory Pattern Criticism and Caveats
Caveats or Criticism
The Abstract Factory pattern is a powerful tool when used correctly, but it does come with a few caveats:
Complexity
The Abstract Factory pattern introduces a fair amount of complexity and abstraction into your code. You're creating an entire additional layer of abstraction over your objects, and this may not be necessary for simpler applications or smaller codebases.
The complexity criticism of the Abstract Factory pattern arises from the fact that it requires creating a new concrete factory for every new "family" of products, and a corresponding interface for each product in that family. This can add a significant amount of extra classes and interfaces to your codebase, which might not be necessary for simpler applications.
In our GUIFactory
implementation, you can see this complexity in action:
interface Button {
click(): void;
}
interface Menu {
open(): void;
}
// The abstract factory interface
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
}
// Concrete implementations
class MobileFactory implements GUIFactory {
createButton(): Button {
return new MobileButton();
}
createMenu(): Menu {
return new MobileMenu();
}
}
class DesktopFactory implements GUIFactory {
createButton(): Button {
return new DesktopButton();
}
createMenu(): Menu {
return new DesktopMenu();
}
}
In this example, for every type of UI element (Button, Menu), we have an interface, and for every type of platform (Mobile, Desktop), we have a concrete factory class. Furthermore, for every UI element on every platform, we have a concrete class. That's a lot of classes and interfaces!
Imagine if we decided to add more UI elements (like a ControlStick) or more
platforms (like Console). For every new UI element, we'd have to add a new
method to the GUIFactory
interface and corresponding methods in each concrete
factory class. For every new platform, we'd have to add a whole new concrete
factory class.
Limited Flexibility In Modifying Product Families
The pattern can be restrictive in terms of flexibility. When new types of products need to be introduced, the core factory interface has to change, affecting all the factory implementations. This violates the Open/Closed Principle of SOLID design principles which states that "software entities ... should be open for extension, but closed for modification".
The Abstract Factory pattern can be inflexible when it comes to modifying product families or adding new types of products. The reason for this lies in the design of the pattern itself, which requires the definition of a fixed interface for each factory.
Let's illustrate this using our GUIFactory
implementation:
interface Button {
click(): void;
}
interface Menu {
open(): void;
}
// The abstract factory interface
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
}
In the above example, GUIFactory
defines methods for creating a Button
and
a Menu
. This works fine as long as these are the only two types of products we
want to create. But what happens if we want to add a new type of product, say
a ControlStick
?
We would need to modify the GUIFactory
interface to include
a createControlStick
method:
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
createControlStick(): ControlStick;
}
This is where we hit a roadblock. According to the Open/Closed Principle, one of
the SOLID principles of object-oriented design, software entities (classes,
modules, functions, etc.) should be open for extension, but closed for
modification. By changing the GUIFactory
interface, we've violated this
principle.
Moreover, we also need to update all classes that implement GUIFactory
to
include this new method. This cascading change could lead to significant
modification in existing codebase, which could in turn introduce new bugs.
class MobileFactory implements GUIFactory {
createButton(): Button {
return new MobileButton();
}
createMenu(): Menu {
return new MobileMenu();
}
createControlStick(): ControlStick {
return new MobileControlStick(); // New method implementation required
}
}
class DesktopFactory implements GUIFactory {
createButton(): Button {
return new DesktopButton();
}
createMenu(): Menu {
return new DesktopMenu();
}
createControlStick(): ControlStick {
return new DesktopControlStick(); // New method implementation required
}
}
So, while the Abstract Factory pattern can provide benefits in terms of encapsulation and consistency, it can also be inflexible when it comes to modifying product families or adding new types of products.
Code Maintenance
As the number of products or families of products increases, maintaining the code can become cumbersome. This is because for every new product, a new method has to be created in the Abstract Factory and implemented in all Concrete Factories.
Code Maintenance can indeed become more complex with the Abstract Factory
Pattern due to the increased number of classes and interfaces. Let's explore
this with our GUIFactory
implementation:
interface Button {
click(): void;
}
interface Menu {
open(): void;
}
// The abstract factory interface
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
}
// Concrete factories
class MobileFactory implements GUIFactory {
createButton(): Button {
return new MobileButton();
}
createMenu(): Menu {
return new MobileMenu();
}
}
class DesktopFactory implements GUIFactory {
createButton(): Button {
return new DesktopButton();
}
createMenu(): Menu {
return new DesktopMenu();
}
}
In this example, we have separate classes for each product (Button
, Menu
)
for each type of factory (MobileFactory
, DesktopFactory
). Now imagine if our
application grows, and we need to add more types of products (
like ControlStick
, Slider
, etc.), or more types of platforms (
like Console
, Tablet
, etc.).
For each new product, we need to:
- Add a new method in the
GUIFactory
interface. - Implement the new method in all existing concrete factory classes.
- Create new product interfaces and concrete classes for the new product.
For each new platform, we need to:
- Create a new concrete factory class.
- Implement all the methods defined in the
GUIFactory
interface in this new class.
This could result in a significant increase in the number of classes and interfaces, making the code more difficult to manage and maintain. It may also lead to code duplication, as similar products for different platforms might have similar implementations.
Tight Coupling And Dependency
The client code becomes dependent on the Abstract Factory interface, which means if you change the interface, you might need to change the client code as well. This may not be a major concern, but it's a dependency that you need to manage.
One criticism of the Abstract Factory pattern is that it can create a certain level of dependency, or tight coupling, between the client code and the abstract factory.
This dependency arises from the fact that the client code must be aware of the abstract factory and its interfaces in order to use the objects that the factory creates. In other words, any changes to the abstract factory interfaces may impact the client code.
Let's illustrate this using the GUIFactory
implementation:
interface Button {
click(): void;
}
interface Menu {
open(): void;
}
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
}
// Using the factory in the client code:
function renderUI(factory: GUIFactory) {
const button = factory.createButton();
button.click();
const menu = factory.createMenu();
menu.open();
}
In the above example, the renderUI
function depends on the GUIFactory
interface. If we decided to add a new UI element, like a ControlStick
, we
would need to modify the GUIFactory
interface to include a method for creating
a ControlStick
:
interface GUIFactory {
createButton(): Button;
createMenu(): Menu;
createControlStick(): ControlStick; // New method
}
Because of this change, we would also need to update the renderUI
function in
the client code to handle the new ControlStick
object:
function renderUI(factory: GUIFactory) {
const button = factory.createButton();
button.click();
const menu = factory.createMenu();
menu.open();
const controlStick = factory.createControlStick(); // Handle the new object
controlStick.move();
}
So, even though the Abstract Factory pattern can help to separate the details of object creation from the client code, it can also introduce a level of dependency between the client code and the factory interfaces, which can lead to tighter coupling and the potential for impact when changes are made. This must be managed carefully when using this pattern.
Remember, like all design patterns, Abstract Factory is a tool that's beneficial when used in the right place. If you find the pattern adds unnecessary complexity or isn't providing enough benefits, it might be a good idea to consider other design patterns or approaches.
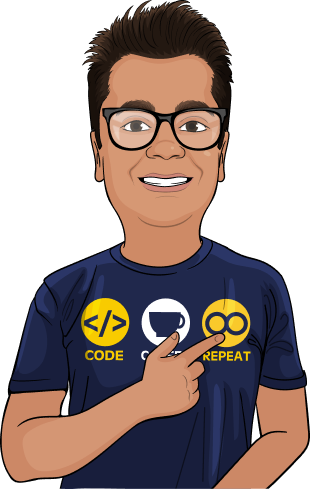
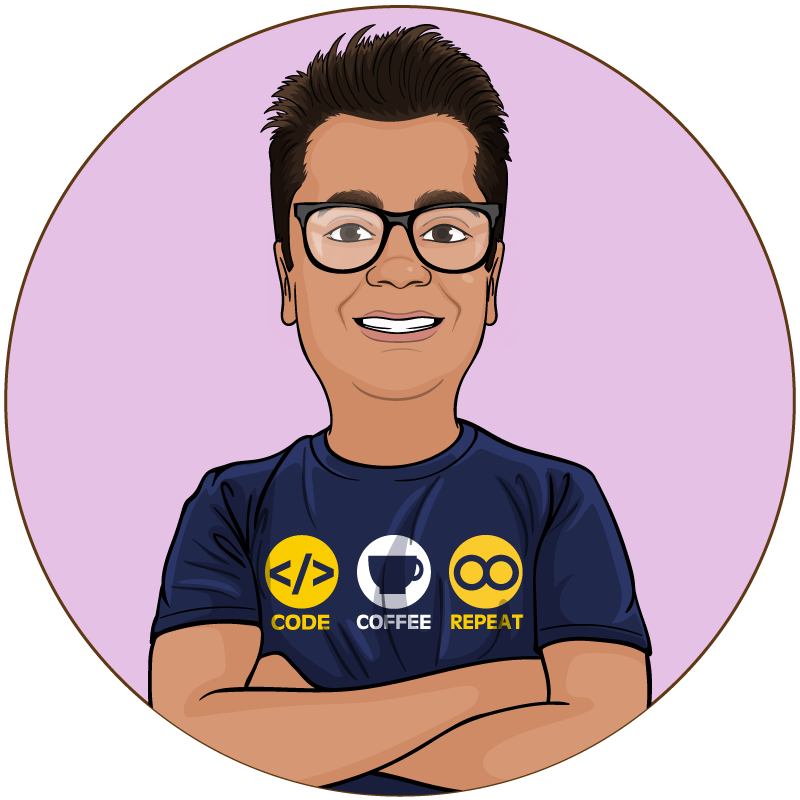
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.