Where To Use The Builder Pattern
What To Consider When Using The Builder Pattern
Deciding whether to use the Builder pattern often involves assessing your application's specific needs and characteristics. However, there are certain scenarios or indicators that can suggest the Builder pattern might be beneficial:
Complex Objects: If you're dealing with objects that require numerous parameters to be set at the time of their creation, especially if many of these parameters are optional, the Builder pattern can help manage this complexity.
Constructors with Many Parameters: If you have a class with a constructor that requires a large number of parameters, this is often a code smell indicating that the Builder pattern may be appropriate.
Immutable Objects: If you're creating immutable objects that must be fully populated at the time of their creation, a Builder can be useful in constructing these objects.
Nested Objects: If the object being constructed contains nested objects which themselves contain multiple parameters, the Builder pattern can make the code easier to write and much easier to read.
Step-by-Step Object Creation: If an object should be created in a specific sequence of steps, the Builder pattern is a good choice.
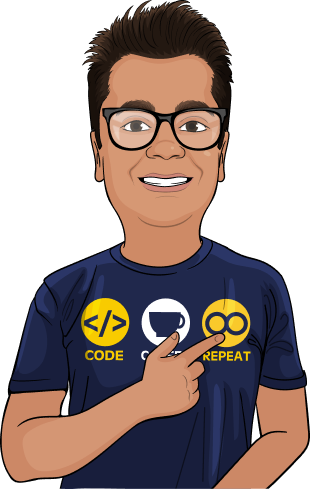
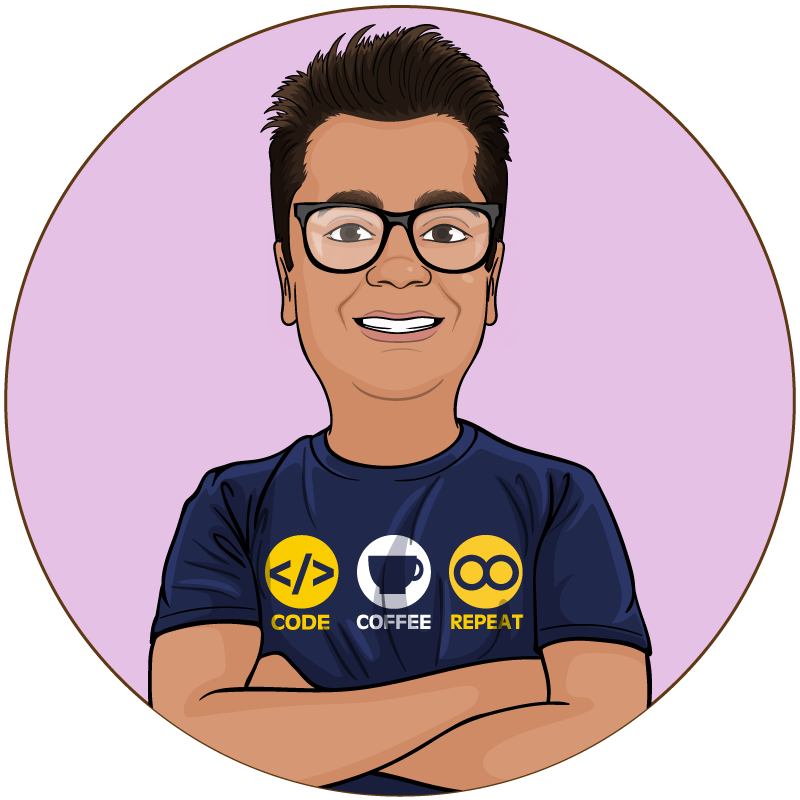
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSBuilder Pattern Application Examples
Here are a few real-world use cases where the builder pattern can be beneficial:
Meal Ordering System
Let's consider a meal ordering system in a restaurant. There are many different types of meals, each having different items (burger, drink, dessert, etc.) and each item can have different variants. For example, a burger can be vegetarian or non-vegetarian, with different types of bread, toppings, and sauces. Using a builder pattern, we could construct each meal step-by-step, allowing for great flexibility and readability.
Here's a simplistic representation of how the builder pattern could be applied in a Meal Ordering System using the mermaid markdown syntax:
In this flowchart:
MealBuilder
is the director class that usesBurgerBuilder
,DrinkBuilder
, andDessertBuilder
to create parts of the meal.- Each specific builder like
BurgerBuilder
,DrinkBuilder
, andDessertBuilder
is responsible for creating specific meal components -Burger
,Drink
, andDessert
. - The
MealOrder
class represents a customer's order and uses theMealBuilder
to create a meal.
This chart shows the separation of construction logic from the actual objects,
as MealOrder
doesn't create meal components directly but relies on
the MealBuilder
and the specific builders.
Construction Industry
Consider a house construction scenario where a construction firm builds different types of houses - such as villas, apartments, bungalows, etc. Each type has different building steps and different components like rooms, garage, swimming pool, garden, etc. A builder pattern would be a good way to encapsulate the complex construction process, allowing different types of buildings to be created in a uniform way.
Here's how the builder pattern could be applied in a Construction Industry scenario using the mermaid markdown syntax:
In this flowchart:
HouseBuilder
is the director class that usesRoomBuilder
,GarageBuilder
, andGardenBuilder
to create parts of the house.- Each specific builder like
RoomBuilder
,GarageBuilder
, andGardenBuilder
is responsible for creating specific house components -Room
,Garage
, andGarden
. - The
ConstructionOrder
class represents a customer's order and uses theHouseBuilder
to create a house.
This chart shows how construction logic is separated from the actual objects,
as ConstructionOrder
doesn't create house components directly but relies on
the HouseBuilder
and the specific builders.
Game Development
In game development, especially in complex strategy or RPG games, characters can have a wide range of attributes and behaviors. A builder pattern can be used to create different characters with different abilities, equipment, skills, etc., in a very readable and manageable way.
Here's a simplified representation of how the builder pattern could be applied in Game Development using the mermaid markdown syntax:
In this flowchart:
CharacterBuilder
is the director class that usesAbilityBuilder
,EquipmentBuilder
, andSkillBuilder
to create parts of the game character.- Each specific builder like
AbilityBuilder
,EquipmentBuilder
, andSkillBuilder
is responsible for creating specific character components -Ability
,Equipment
, andSkill
. - The
GameCharacter
class represents a character in the game and uses theCharacterBuilder
to create a game character.
This chart shows how construction logic is separated from the actual objects,
as GameCharacter
doesn't create character components directly but relies on
the CharacterBuilder
and the specific builders.
Document Creation
Imagine a document conversion system where a document can be represented in various formats like HTML, PDF, or Markdown. Each document consists of parts like headers, paragraphs, footers, etc. The construction process of each document type can be encapsulated using a builder for each format. This way, a uniform interface can be maintained for the document creation, while supporting a wide range of document formats.
Absolutely, here's a simplistic representation of how the builder pattern could be applied in Document Creation using the mermaid markdown syntax:
In this flowchart:
DocumentBuilder
is the director class that usesHeaderBuilder
,ParagraphBuilder
, andFooterBuilder
to create parts of the document.- Each specific builder like
HeaderBuilder
,ParagraphBuilder
, andFooterBuilder
is responsible for creating specific document components -Header
,Paragraph
, andFooter
. - The
Document
class represents a document and uses theDocumentBuilder
to create a document.
This chart shows how construction logic is separated from the actual objects,
as Document
doesn't create document components directly but relies on
the DocumentBuilder
and the specific builders.
E-commerce System
In an e-commerce system, creating a product with various characteristics like size, color, material, manufacturer, price, etc., can be complex. Using a builder pattern, the product creation process can be made more manageable and scalable.
Here's a representation of how the builder pattern could be applied in an E-commerce System using the mermaid markdown syntax:
In this flowchart:
ProductBuilder
is the director class that usesDescriptionBuilder
,PriceBuilder
, andImageBuilder
to create parts of the product.- Each specific builder like
DescriptionBuilder
,PriceBuilder
, andImageBuilder
is responsible for creating specific product components -Description
,Price
, andImage
. - The
Product
class represents a product on the e-commerce platform and uses theProductBuilder
to create a product.
This chart shows how construction logic is separated from the actual objects,
as Product
doesn't create product components directly but relies on
the ProductBuilder
and the specific builders.
In each of these cases, the Builder pattern helps manage the complexity of creating diverse objects, while maintaining a consistent and readable interface. It helps to keep the code flexible and maintainable, which is especially beneficial in large, evolving projects.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.