Criticism or Caveats Prototype Pattern
While these are some of the caveats, the Prototype pattern can still be very useful when used appropriately, considering the specific requirements and constraints of your project.
Prototype pattern has many benefits, it's not without its caveats. Here are a few important ones that need to be considered:
Shallow vs Deep Copying
By default, object assignment in JavaScript and TypeScript creates a shallow copy, meaning that nested objects are copied by reference rather than by value. If you're cloning an object with nested objects and you want those to be distinct copies (not references), you'll need to implement a deep copy. This can be complex and potentially performance-intensive.
Absolutely, let's consider this in the context of a simple JavaScript object.
Consider this object:
let original = {
name: "John",
address: {
street: "123 Main St",
city: "New York",
},
};
If you were to create a shallow copy of this object like this:
let shallowCopy = { ...original };
And then change a property of the nested object:
shallowCopy.address.city = "Los Angeles";
You'll see that the original object is also modified:
console.log(original.address.city);
// Outputs 'Los Angeles'
This is because a shallow copy only creates a new object at the top level. Nested objects are copied as references, so changes to the copy's nested objects affect the original object's nested objects.
Now, let's consider a deep copy. A simple way to make a deep copy in JavaScript
is to use JSON.parse
and JSON.stringify
:
let deepCopy = JSON.parse(JSON.stringify(original));
If you now change a property of the nested object:
deepCopy.address.city = "San Francisco";
The original object remains unchanged:
console.log(original.address.city);
// Outputs 'Los Angeles'
This is because a deep copy creates new objects for nested objects as well. So changes to the copy's nested objects don't affect the original object's nested objects.
Problems With JSON.parse and JSON.stringify
Again, using JSON.parse
and JSON.stringify
for deep copying is a
simple but limited approach, as it only works with JSON-compatible data. It
won't correctly copy complex objects such as those with function properties,
Date objects, or circular references. For complex cases, you might need a more
sophisticated approach or a library specifically designed for deep cloning.
The typical ways to deep clone objects in JavaScript were:
- Using
JSON.parse(JSON.stringify(obj))
for JSON-safe objects. - Using recursion to manually copy each property for more complex objects.
- Using a library like lodash's
_.cloneDeep()
method, which handled a wide range of edge cases.
However, with the introduction of the structured cloning algorithm
in
JavaScript, we can now use the structuredClone()
method to deep clone.
let deepCopy = structuredClone(original);
deepCopy.address.city = "Los Angeles";
console.log(original.address.city);
// Outputs 'New York'
console.log(deepCopy.address.city);
// Outputs 'Los Angeles'
Resource-intensive operations
If an object requires a lot of resources for cloning, using the Prototype pattern might not be efficient. Cloning an object might involve constructors, which could be a costly operation depending upon what's happening in the constructor.
Let's say we have a DatabaseRecord
class that queries a database when it's
instantiated.
Cloning such an object could potentially be very resource-intensive.
interface IDatabaseRecordPrototype {
clone(): IDatabaseRecordPrototype;
getDataFromDatabase(id: number): any;
}
class DatabaseRecord implements IDatabaseRecordPrototype {
data: any;
constructor(id: number) {
this.data = this.getDataFromDatabase(id);
}
clone(): IDatabaseRecordPrototype {
let clone = new DatabaseRecord(this.data.id);
return clone;
}
getDataFromDatabase(id: number): any {
console.log(`Querying database for record with id ${id}`);
return { id: id, value: "Some data" };
}
}
let original = new DatabaseRecord(1);
let clone: DatabaseRecord = original.clone() as DatabaseRecord;
In this example, creating a DatabaseRecord
object involves a (simulated)
database query, which is a costly operation. When we clone a DatabaseRecord
,
it also performs this operation because the clone()
method calls the
constructor.
In this case, if you frequently clone DatabaseRecord
objects, you'd end up
making many unnecessary database queries, which could significantly slow down
your application and consume a lot of resources.
This is a contrived example, but it shows why you would want to be careful about what happens during object creation and cloning when using the Prototype pattern. In some cases, it might be more efficient to not fully clone an object, but to share some common data between instances, or to implement lazy loading of expensive data. As always, the best approach depends on the specific requirements and constraints of your project.
Solving the resource-intensive operations problem
Yes, there are several strategies you can use to avoid resource-intensive operations during object cloning with the Prototype pattern.
Lazy Copy: This strategy involves deferring the copying of internal objects until they're needed. If an internal object is never modified, you don't need to create a separate copy for the cloned object, and both can share the original object.
Copy-on-write: This strategy is a variation of the lazy copy. It doesn't actually copy internal objects until they're modified. When a cloned object tries to modify an internal object, it first checks whether it's shared with the original object. If it is, it makes a copy before modifying it. This avoids unnecessary copying if the internal objects aren't modified.
Shared references: If your data doesn't change, or if it's okay for clones to share some data, you can keep a reference to the original data in your cloned objects. This allows you to save resources by sharing large, complex, or computation-intensive resources between clones.
Here's an example demonstrating the shared references strategy:
interface IPrototype {
clone(): IPrototype;
}
class DatabaseData implements IPrototype {
private data: any;
constructor(data: any) {
this.data = data;
}
clone(): IPrototype {
// Creating a new instance that shares the same data reference.
let clone = new DatabaseData(this.data);
return clone;
}
}
class DatabaseRecord {
data: DatabaseData;
constructor(id: number) {
// Assume that getDataFromDatabase is a costly operation, like a database query.
this.data = this.getDataFromDatabase(id) as DatabaseData;
}
getDataFromDatabase(id: number): IPrototype {
// Simulating a database operation...
console.log(`Querying database for record with id ${id}`);
return new DatabaseData({ id: id, value: "Some data" });
}
}
let original = new DatabaseRecord(1);
let cloneData: DatabaseData = original.data.clone() as DatabaseData;
console.log(cloneData); // Outputs { id: 1, value: 'Some data' }
In this example, the clone()
method creates a new ResourceIntensiveObject
that shares the same data
reference with the original object, instead of
creating a new copy of data
. This is much less resource-intensive than the
previous version.
But remember, these strategies aren't perfect solutions for every situation. They're suitable when certain conditions are met, such as when data doesn't change frequently, or when it's acceptable for cloned objects to share some or all of their data with the original object. The best strategy depends on the specific requirements and constraints of your project.
Tight Coupling
While this approach provides a way to manage resource-intensive data and its duplication, it's important to note that it introduces a high degree of coupling between the DatabaseRecord and DatabaseData classes. Such tight coupling could make the code less flexible and harder to maintain, especially in larger systems.
Decoupling these classes could involve introducing abstractions (like interfaces or abstract classes) or dependency injection. The optimal approach depends on the broader context of your application, such as its size, complexity, and the likelihood of changes in the future. Always consider these factors when designing your classes and their interactions.
It's also crucial to note that the Prototype pattern may not always be the best solution, and there might be alternative design patterns or strategies more suitable for specific scenarios. It's important to weigh the advantages and disadvantages, understand the problem domain thoroughly, and choose the most appropriate pattern or strategy.
Complexity with custom clone methods
"Complexity with custom clone methods," refers to situations where the cloning operation might not be as straightforward as duplicating all properties of an object.
This could happen if some of the properties should not be cloned, or if the object contains cyclical references, or when the object's properties are instances of classes that also need to be cloned. Implementing a custom clone method in these situations can add a layer of complexity to the Prototype pattern.
Here's an example to illustrate this caveat:
interface IPrototype {
clone(): IPrototype;
}
class ComplexObject implements IPrototype {
simpleProp: string;
complexProp: ComplexSubObject;
constructor(simpleProp: string, complexProp: ComplexSubObject) {
this.simpleProp = simpleProp;
this.complexProp = complexProp;
}
clone(): IPrototype {
// We can't simply clone all properties, as complexProp needs to be cloned as well.
let clone = new ComplexObject(
this.simpleProp,
this.complexProp.clone() as ComplexSubObject
);
return clone;
}
}
class ComplexSubObject implements IPrototype {
someProp: string;
constructor(someProp: string) {
this.someProp = someProp;
}
clone(): IPrototype {
let clone = new ComplexSubObject(this.someProp);
return clone;
}
}
In this example, the ComplexObject
class has a simple property (simpleProp
)
and a complex property (complexProp
). When cloning a ComplexObject
, we can't
simply duplicate all properties, because complexProp
is an instance
of ComplexSubObject
and needs to be cloned as well. Therefore, we need to
implement a custom clone method that handles this scenario.
As the complexity of the objects and their relationships increases, the complexity of the custom clone methods will increase as well. This can make the code harder to understand and maintain. It's also easy to make mistakes, like forgetting to clone a property, which can lead to bugs that are hard to track down.
It's important to be aware of these complexities and to carefully design your objects and their clone methods to manage them effectively. In some cases, alternative design patterns or strategies might be a better fit.
How Complex Object Can Look Like
Sure! Let's go through each of these situations:
- Some properties should not be cloned: In some scenarios, not all properties of the object need to be cloned. For example, let's say we have a unique id for each object which should not be duplicated:
class UniqueIdObject implements IPrototype {
id: number;
data: string;
constructor(id: number, data: string) {
this.id = id;
this.data = data;
}
clone(): IPrototype {
// The unique id should not be cloned.
let clone = new UniqueIdObject(Math.random(), this.data);
return clone;
}
}
In the above example, the UniqueIdObject
has a property id
which should not
be cloned. Thus, the clone
method creates a new id for the cloned object.
- Cyclical references: Cyclical references occur when an object has a property that references itself. Naive cloning methods would result in an infinite loop in this situation:
class CyclicalReferenceObject implements IPrototype {
selfReference: CyclicalReferenceObject | null;
constructor() {
this.selfReference = null;
}
clone(): IPrototype {
let clone = new CyclicalReferenceObject();
// WARNING: This line would cause an infinite loop!
// clone.selfReference = this.selfReference.clone() as CyclicalReferenceObject;
return clone;
}
}
In this example, naive cloning would result in an infinite loop,
because CyclicalReferenceObject
has a selfReference
property that refers to
itself.
- Properties are instances of classes that also need to be cloned: I
actually covered this in the previous
ComplexObject
andComplexSubObject
example. In a complex object graph, each object might need to implement its ownclone
method:
class ComplexObject implements IPrototype {
subObject: ComplexSubObject;
constructor(subObject: ComplexSubObject) {
this.subObject = subObject;
}
clone(): IPrototype {
let clone = new ComplexObject(this.subObject.clone() as ComplexSubObject);
return clone;
}
}
class ComplexSubObject implements IPrototype {
data: string;
constructor(data: string) {
this.data = data;
}
clone(): IPrototype {
let clone = new ComplexSubObject(this.data);
return clone;
}
}
Here, ComplexObject
has a property subObject
that is an instance
of ComplexSubObject
, and ComplexSubObject
also implements a clone
method.
Therefore, ComplexObject
's clone
method needs to call subObject.clone()
to
correctly clone subObject
.
As you can see, each of these situations can add complexity to the clone
method. They require a deeper understanding of the object's properties and the
relationships between them. When using the Prototype pattern, it's crucial to
consider these factors to ensure that objects are cloned correctly.
While these are some of the caveats, the Prototype pattern can still be very useful when used appropriately, considering the specific requirements and constraints of your project.
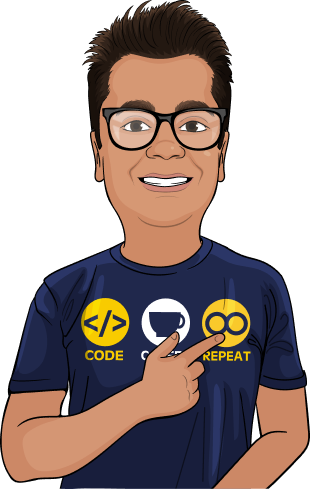
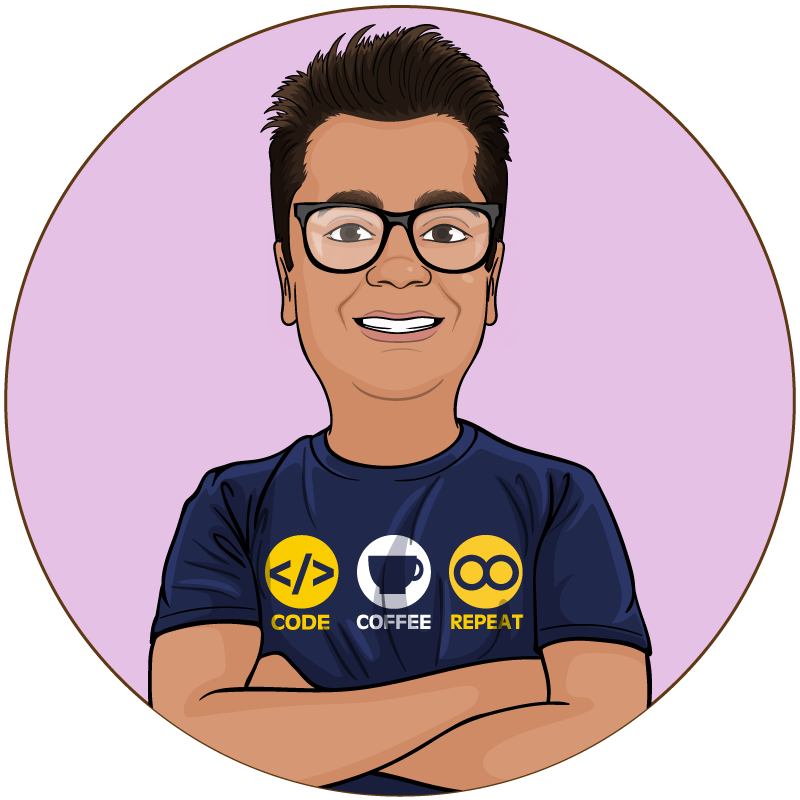
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.