TypeORM as an Example Of Abstraction 🤔
TypeORM is an abstraction layer over various databases, which allows developers to interact with different types of databases using a unified, high-level API. This abstraction layer reduces the need to write database-specific SQL queries and provides a more JavaScript or TypeScript-friendly way of dealing with data persistence.
Sure, let's dive into how TypeORM acts as a layer of abstraction, especially when it comes to defining a schema, querying the database, and inserting an entry into a table.
1. Defining a Schema
In TypeORM, you define a schema by creating entities. An entity is a class that maps to a database table. Each instance of the entity represents a row in the table. Here's an example:
import { Entity, PrimaryGeneratedColumn, Column } from "typeorm";
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
firstName: string;
@Column()
lastName: string;
@Column()
age: number;
}
In this example, a User
class maps to a User
table in the database. Each
property of the User
class maps to a column in the table.
The @Entity
, @PrimaryGeneratedColumn
, and @Column
decorators are used to
declare the mapping.
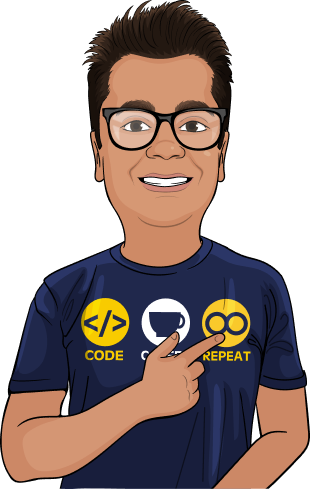
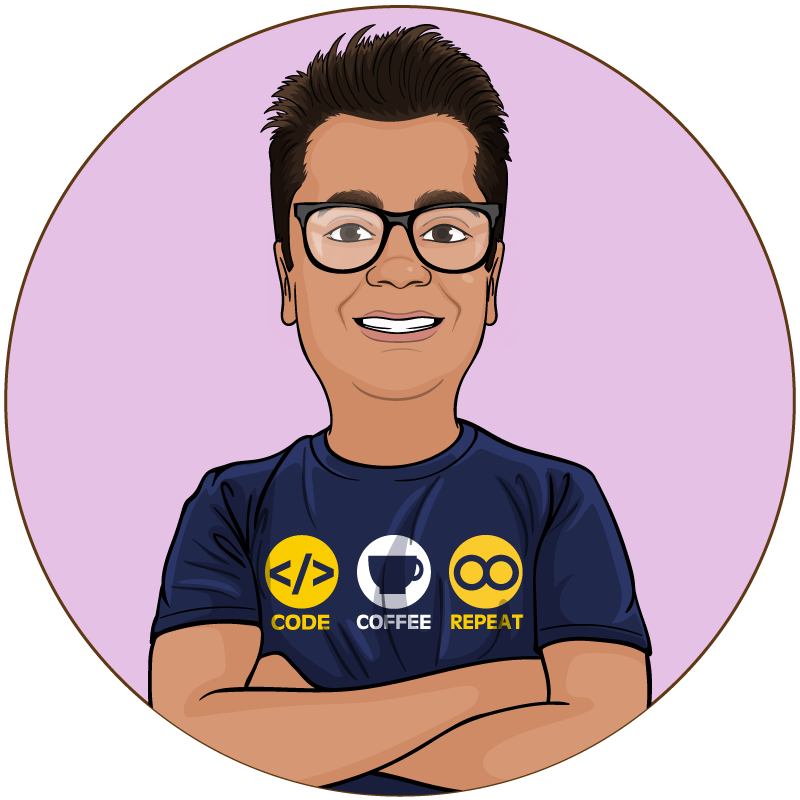
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILS2. Querying the Database
TypeORM provides a repository API to fetch data from the database. This allows you to write queries in a way that is closer to TypeScript and JavaScript. Here's an example:
import { getRepository } from "typeorm";
import { User } from "./User";
async function getUser() {
const userRepository = getRepository(User);
const user = await userRepository.findOne({ id: 1 });
console.log(user);
}
In this example, getRepository(User)
gets you a repository for the User
entity. The repository has methods like findOne
, find
, save
, etc. that
allow you to query the database. The findOne
method fetches a single user
with id
of 1.
3. Inserting an Entry into a Table
Inserting data into a table involves creating an instance of the entity and then saving it using the repository. Here's an example:
import { getRepository } from "typeorm";
import { User } from "./User";
async function createUser() {
let user = new User();
user.firstName = "John";
user.lastName = "Doe";
user.age = 25;
const userRepository = getRepository(User);
await userRepository.save(user);
console.log("User has been saved.");
}
In this example, we create a new User
instance and then save it using
the save
method of the User
repository. The save
method handles both
insert and update operations. If the User
instance doesn't exist in the
database, it will be inserted. If it does exist, it will be updated.
TypeORM provides an abstraction layer over the database, allowing you to work with the database in a more JavaScript/TypeScript-friendly way. It eliminates the need to write raw SQL queries for basic CRUD operations and provides a more object-oriented way of working with the database.
Why Use Abstraction 🤔
Simplicity: Abstraction allows developers to hide complex details and expose only the necessary parts of an object. This makes it easier to interact with objects without understanding the underlying complexity.
Maintainability: By hiding the internal workings of objects, any changes made inside an object do not affect the rest of the application. This makes maintaining and updating the software easier.
Reusability: With abstraction, developers can create generic classes or interfaces that can be reused across different parts of an application. This leads to less code duplication and enhances code reusability.
Modularity: Abstraction encourages a more modular design. Each object manages its own behavior based on its state, leading to well-structured and modular code.
Security: Abstraction also provides a level of security by hiding the internal states and implementation details of objects. Only methods exposed and intended to be accessed can be operated by other classes, preventing unauthorized access and misuse.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.