Introduction To Object Oriented Programming Paradigm
Object Oriented Programming (OOP) is a programming paradigm that uses the concept of objects as a way to structure and organize code.
What is Object Oriented Programming (OOP) 🤔
Object Oriented Programming (OOP) is a programming paradigm that uses the concept of objects as a way to structure and organize code. It is based on the principles of abstraction, encapsulation, inheritance, and polymorphism, which enable developers to build more flexible, reusable, and maintainable software.
Here is a graph illustrating OOP and its four main concepts as branches. Each branch has a brief explanation of the corresponding concept to help visualize their relationships and their importance within the OOP paradigm.
Abstraction
This principle allows programmers to represent real-world entities as objects in code, hiding their complexity and focusing on the essential features relevant to the problem being solved. By using abstraction, developers can create simplified models of complex systems.
Encapsulation
Encapsulation is the mechanism of bundling data (attributes) and operations (methods) together within a single unit, called a class. This principle helps to achieve separation of concerns, allowing each object to manage its own state and behavior, and restrict access to its internal data.
Inheritance
Inheritance enables the creation of new classes that inherit attributes and methods from existing classes. The new classes, called subclasses, can extend or override the behavior of the parent class (also known as the base or superclass). This promotes code reusability and modularity, as common functionalities can be shared among related classes.
Polymorphism
Polymorphism allows a single interface to represent different types of objects. This enables objects of different classes to be treated as objects of a common superclass, allowing the same code to work with different data types. Polymorphism simplifies code maintenance and enables developers to create more flexible and extensible systems.
Here's a simple UML diagram that illustrates the concepts of OOP:
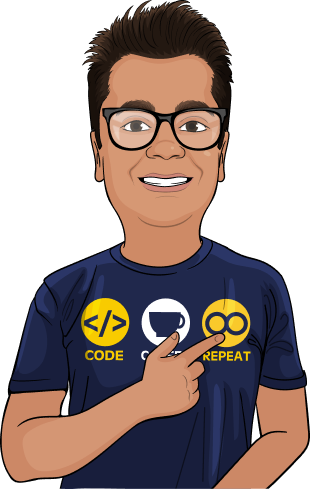
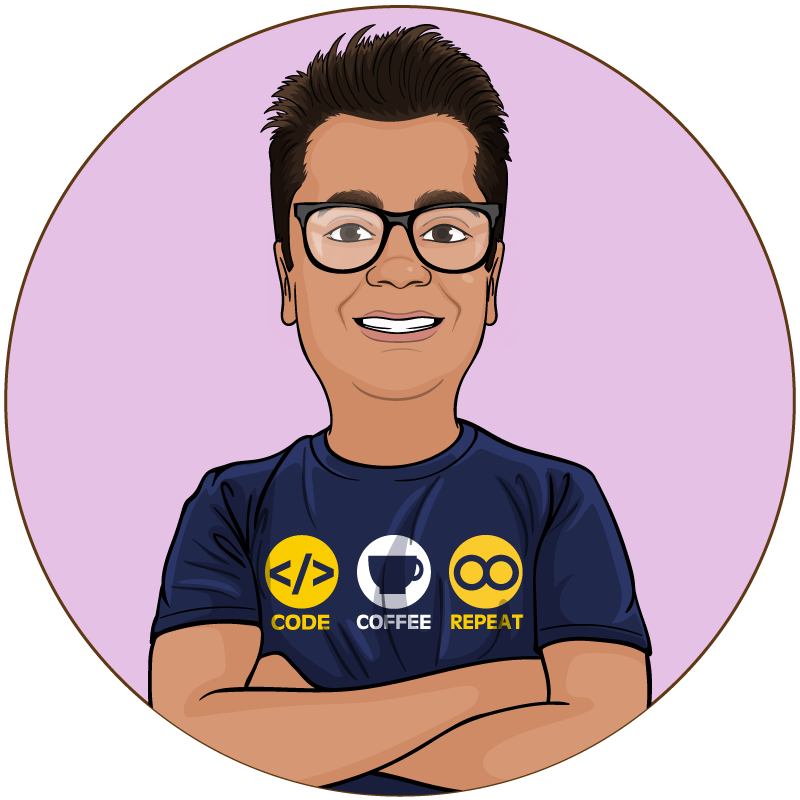
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSObject-oriented programming is popular in many modern programming languages, such as Java, Python, C++, and C#. By employing OOP principles, developers can build robust and scalable applications, making it an important paradigm in the software development world.
Other Programming Paradigms
Apart from Object-Oriented Programming (OOP), there are several other programming paradigms that developers use to design and structure their code. Here are five examples:
Procedural Programming
This paradigm is based on the concept of procedures (also known as routines, subroutines, or functions), which are a series of computational steps to be carried out. Procedural programming focuses on the explicit sequence of actions required to solve a problem, using constructs such as loops, conditionals, and variables. Examples of procedural programming languages include C, Pascal, and Fortran.
Functional Programming
Functional programming treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. It emphasizes immutability, first-class functions, and the use of higher-order functions, leading to more predictable and easier-to-debug code. Examples of functional programming languages include Haskell, Lisp, Erlang, and Clojure.
Logic Programming
This paradigm is based on formal logic, where programs consist of a series of statements expressing facts and rules about a problem domain. The programming language's interpreter searches for proofs of queries by applying inference rules on the facts and rules defined in the program. Examples of logic programming languages include Prolog and Mercury.
Event-Driven Programming
In this paradigm, the flow of the program is determined by events, such as user actions, sensor outputs, or messages from other programs. The program defines event handlers, which are functions that are triggered when specific events occur. This paradigm is often used in graphical user interfaces, real-time systems, and serverless computing. Examples of event-driven programming languages or frameworks include JavaScript, Node.js, and Python's Twisted.
Concurrent Programming
This paradigm is designed to handle multiple tasks simultaneously, either by dividing a problem into smaller sub-tasks that can be executed concurrently or by running multiple independent tasks in parallel. Concurrent programming often relies on constructs like threads, processes, or coroutines, and requires developers to consider synchronization and communication between tasks. Examples of languages that support concurrent programming include Go, Rust, and Java.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.