What is Abstraction 🤔
Abstraction in Object-Oriented Programming (OOP) refers to the process of simplifying complex systems by breaking them down into smaller, more manageable components.
It involves hiding the implementation details of a system and exposing only the essential features to the user. Abstraction allows developers to focus on the core functionality of a component without being concerned with the underlying complexity, making code easier to understand, maintain, and extend.
Abstraction In TypeScript
In TypeScript, abstraction is achieved through classes and interfaces. Classes allow you to define blueprints for creating objects, while interfaces define the contract that a class must adhere to. Interfaces are used to enforce a certain structure on classes, ensuring that they have the required properties and methods.
Here's a basic example of abstraction using TypeScript:
// Define an interface for a shape
interface Shape {
area(): number;
perimeter(): number;
}
// Implement the Shape interface with a Circle class
class Circle implements Shape {
constructor(private radius: number) {}
area(): number {
return Math.PI * this.radius * this.radius;
}
perimeter(): number {
return 2 * Math.PI * this.radius;
}
}
// Implement the Shape interface with a Rectangle class
class Rectangle implements Shape {
constructor(private width: number, private height: number) {}
area(): number {
return this.width * this.height;
}
perimeter(): number {
return 2 * (this.width + this.height);
}
}
// Function to calculate the total area of an array of shapes
function calculateTotalArea(shapes: Shape[]): number {
let totalArea = 0;
for (const shape of shapes) {
totalArea += shape.area();
}
return totalArea;
}
// Create instances of Circle and Rectangle
const circle = new Circle(5);
const rectangle = new Rectangle(4, 6);
// Calculate the total area using the array of shapes
const shapes: Shape[] = [circle, rectangle];
console.log("Total area:", calculateTotalArea(shapes)); // Output: Total area: 103.53981633974483
In this example, we define a Shape
interface with two methods: area()
and perimeter()
. We then create two classes, Circle
and Rectangle
, that
implement the Shape
interface. This enforces that both classes have
the area()
and perimeter()
methods, providing a level of abstraction.
The calculateTotalArea()
function accepts an array of Shape
objects and
calculates the total area, demonstrating the benefits of abstraction by working
with different shape types in a consistent and simplified manner.
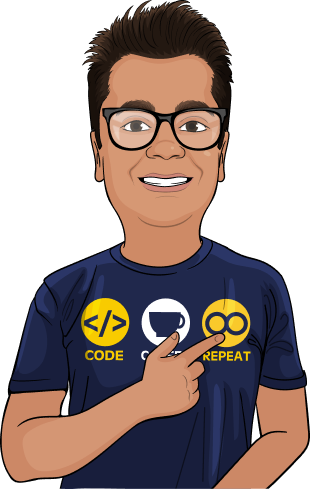
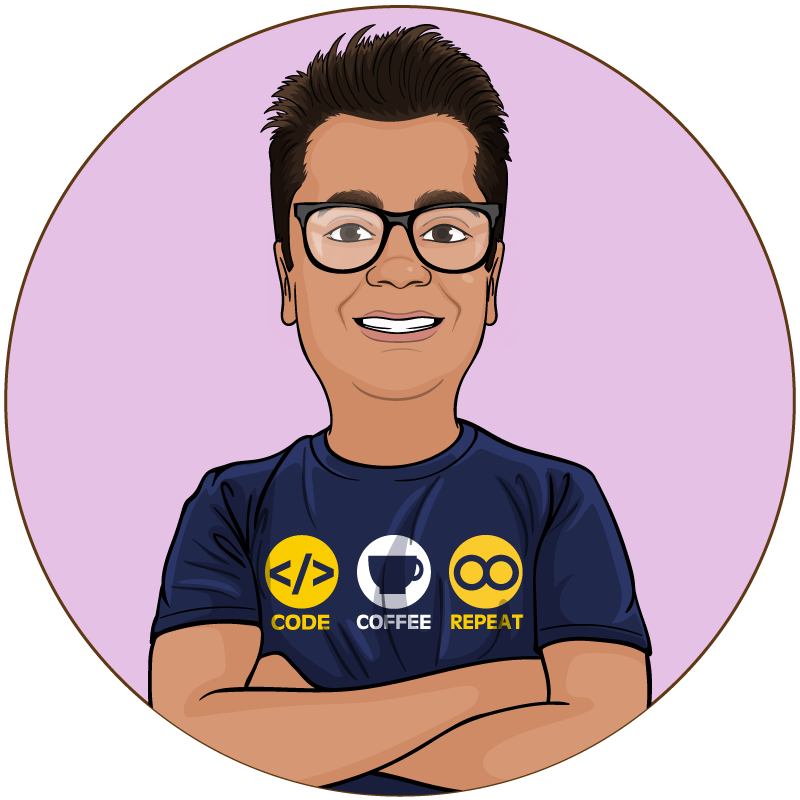
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Example
The JavaScript Date class provides an abstraction for working with dates and times. It encapsulates the complexity of date and time calculations, while providing a simple and intuitive API for developers to manipulate and retrieve date and time information.
The Date class hides details like leap years, time zones, daylight saving time, and the actual internal representation of date and time values. Developers can use the provided methods to create, parse, compare, and manipulate dates and times without having to worry about these complexities.
Here's a real-world example demonstrating how the Date class uses abstraction:
// Get the current date and time
const now = new Date();
// Get the current year, month, and date
const currentYear = now.getFullYear();
const currentMonth = now.getMonth() + 1; // Month is zero-based, so we add 1
const currentDate = now.getDate();
console.log(`Today is ${currentYear}-${currentMonth}-${currentDate}`);
// Calculate the number of days until a specific date (e.g., Christmas)
const christmas = new Date(currentYear, 11, 25); // Month is zero-based, so December is 11
const millisecondsPerDay = 24 * 60 * 60 * 1000;
const daysUntilChristmas = Math.ceil((christmas - now) / millisecondsPerDay);
console.log(`There are ${daysUntilChristmas} days until Christmas`);
// Format the current date using the built-in toLocaleDateString method
const formattedDate = now.toLocaleDateString("en-US", {
weekday: "long",
year: "numeric",
month: "long",
day: "numeric",
});
console.log(`Today is ${formattedDate}`); // Output: Today is Thursday, April 27, 2023
In this example, we use the Date class to obtain the current date and time, extract the year, month, and date, calculate the number of days until Christmas, and format the current date according to a specific locale. The Date class provides a simple API to perform these tasks, while abstracting away the underlying complexity of date and time calculations.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.