What is Encapsulation 🤔
Encapsulation is a fundamental concept in object-oriented programming (OOP) that refers to the practice of bundling together data (attributes) and the methods ( functions) that operate on that data within a single unit, typically a class.
Encapsulation In TypeScript
Encapsulation promotes the separation of concerns and data hiding, making it easier to reason about, maintain, and reuse code.
In encapsulation, an object's internal state is protected from direct manipulation or access by external code. Instead, access to the object's state is provided through a well-defined interface, usually in the form of getter and setter methods. This allows for better control and validation of data, and it ensures that the object's internal state remains consistent.
Here's an example of encapsulation using TypeScript:
class BankAccount {
private _balance: number;
constructor(initialBalance: number) {
this._balance = initialBalance;
}
// Getter method for balance
public get balance(): number {
return this._balance;
}
// Method to deposit money
public deposit(amount: number): void {
if (amount < 0) {
console.log("Invalid deposit amount");
return;
}
this._balance += amount;
}
// Method to withdraw money
public withdraw(amount: number): void {
if (amount < 0) {
console.log("Invalid withdrawal amount");
return;
}
if (this._balance - amount < 0) {
console.log("Insufficient funds");
return;
}
this._balance -= amount;
}
}
const myAccount = new BankAccount(1000);
myAccount.deposit(500);
myAccount.withdraw(200);
console.log("Current balance:", myAccount.balance);
In this example, the BankAccount
class encapsulates the data
attribute _balance
and provides methods to interact with
it (deposit
, withdraw
, and a getter for balance
). The _balance
attribute
is marked as private
, ensuring that it cannot be accessed or modified directly
from outside the class. Instead, the class provides a well-defined interface
through its public methods, which can be used to interact with the object's
state.
The deposit
and withdraw
methods also enforce some basic validation to
ensure that the internal state of the object remains consistent (e.g., not
allowing negative amounts or withdrawals that exceed the current balance). This
is an example of encapsulation in action, as it helps maintain data integrity
and makes the code more robust.
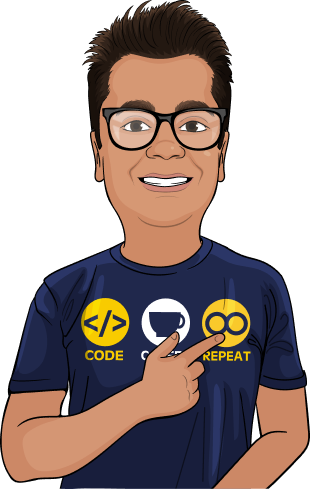
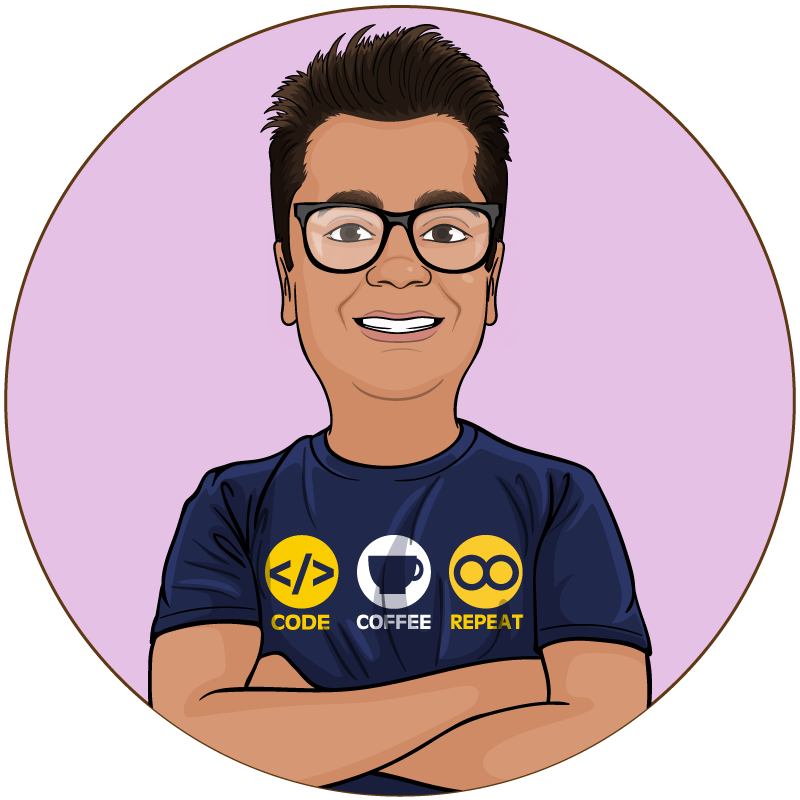
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Example
The JavaScript Date
class is a built-in object that represents a single moment
in time, providing various methods to create, manipulate, and retrieve date and
time information. The Date
class encapsulates the underlying timestamp (i.e.,
the number of milliseconds elapsed since the Unix epoch: January 1, 1970, 00:00:
00 UTC) and exposes a set of public methods for interacting with it.
Here's an example of how you can use the Date
class in JavaScript:
const now = new Date();
console.log("Current date and time:", now.toString());
const specificDate = new Date("2023-05-01T00:00:00");
console.log("Specific date:", specificDate.toString());
console.log("Current year:", now.getFullYear());
console.log("Current month (0-based):", now.getMonth());
console.log("Current date:", now.getDate());
now.setFullYear(2024);
console.log("Modified date:", now.toString());
In this example, we create two Date
objects: now
representing the current
date and time and specificDate
representing a specific date and time.
The Date
class provides several getter and setter methods to access and modify
its internal state, like getFullYear
, getMonth
, getDate
,
and setFullYear
. These methods serve as an interface to interact with the
encapsulated timestamp.
Behind the scenes, the Date
class encapsulates the timestamp, which is stored
as a private property. This property isn't directly accessible or modifiable
from outside the class. Instead, the class provides getter and setter methods to
manipulate the encapsulated data. This is an example of encapsulation, as it
hides the internal implementation details and provides a well-defined interface
for working with date and time information.
It's worth noting that JavaScript doesn't have explicit access modifiers
like private
or public
. The encapsulation is achieved through convention and
closures, rather than strict enforcement by the language. However, the Date
class still adheres to the principles of encapsulation, ensuring that the
internal state is protected and manipulated through a well-defined set of
methods.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.