What is Inheritance 🤔
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows one class to inherit properties (attributes) and methods (functions) from another class, thereby promoting code reusability and modularity.
Inheritance In TypeScript
Inheritance
helps in creating a new class (derived class) from an existing class (base
class) while maintaining the existing class's behavior and extending or
overriding it to add new functionalities. In TypeScript, inheritance is
implemented using the extends
keyword.
Here's an example to illustrate inheritance in TypeScript:
// Base class: Animal
class Animal {
protected name: string;
constructor(name: string) {
this.name = name;
}
move(distance: number = 0) {
console.log(`${this.name} moved ${distance} meters.`);
}
}
// Derived class: Dog, which inherits from Animal
class Dog extends Animal {
constructor(name: string) {
super(name);
// Calls the constructor of the base class
}
bark() {
console.log("Woof! Woof!");
}
// Overriding the move method from the Animal class
move(distance: number = 5) {
console.log("The dog is running...");
super.move(distance);
// Calls the move method of the base class
}
}
const myDog = new Dog("Max");
myDog.bark();
// Output: Woof! Woof!
myDog.move();
// Output: The dog is running...
// Max moved 5 meters.
In this example, we have a base class Animal
with a name
attribute, a
constructor, and a move
method. We then create a derived class Dog
that
inherits from Animal
using the extends
keyword. The Dog
class has its
own bark
method and overrides the move
method from the Animal
class. When
creating a new Dog
instance, we can use both the bark
method from the Dog
class and the move
method from the Animal
class (with the overridden
behavior).
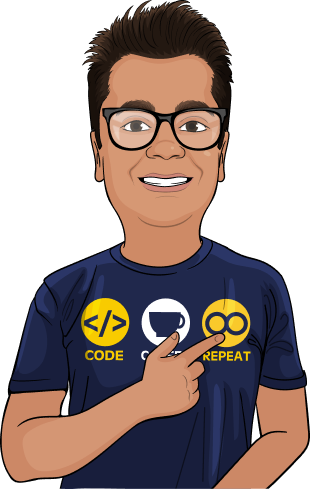
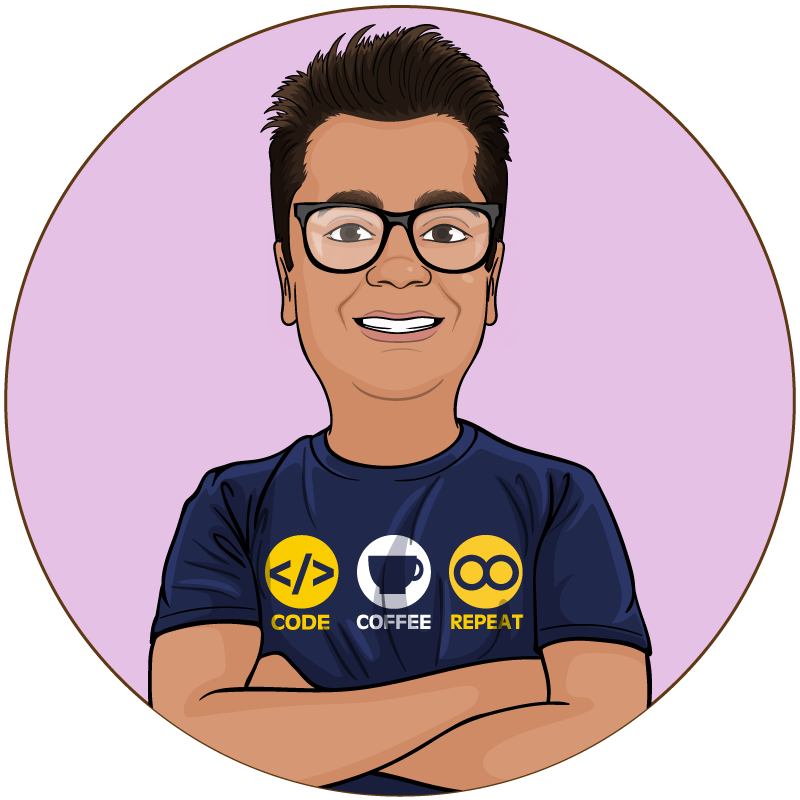
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Application Example
Let's take a look at a more real-world scenario involving an e-commerce application where TypeScript inheritance might be used:
In this diagram, we have three classes: Product
, Book
, and Electronic
.
Both Book
and Electronic
classes are shown as inheriting from the Product
class with the --|>
symbol. The class fields and methods are also outlined.
The +
symbol before the field names and method names indicates that they are
public.
// The base class Product which defines common properties for all products
class Product {
constructor(
public id: string,
public price: number,
public description: string
) {}
display(): void {
console.log(
`ID: ${this.id}, Price: ${this.price}, Description: ${this.description}`
);
}
}
// A derived class Book which extends the Product base class
class Book extends Product {
constructor(
id: string,
price: number,
description: string,
public author: string,
public title: string
) {
super(id, price, description);
// Calls the constructor of the base class
}
display(): void {
super.display();
// Calls the display method from the base class
console.log(`Author: ${this.author}, Title: ${this.title}`);
}
}
// A derived class Electronic which extends the Product base class
class Electronic extends Product {
constructor(
id: string,
price: number,
description: string,
public brand: string,
public model: string
) {
super(id, price, description);
// Calls the constructor of the base class
}
display(): void {
super.display(); // Calls the display method from the base class
console.log(`Brand: ${this.brand}, Model: ${this.model}`);
}
}
let book = new Book("1", 19.99, "A great book", "John Doe", "My Great Book");
book.display();
let electronic = new Electronic(
"2",
299.99,
"A great laptop",
"Dell",
"XPS 15"
);
electronic.display();
In this example, we have an e-commerce application where we sell various types
of products. We start with a base Product
class that includes properties and
methods common to all products, such as an ID, a price, a description, and a
method to display this information.
We then define a Book
class and an Electronic
class that both inherit from
the Product
class. Each of these classes has additional properties specific to
its type (like author
and title
for Book
, and brand
and model
for Electronic
), and they override the display
method to include their
specific properties.
This example shows how TypeScript inheritance can be used to create a more organized and maintainable codebase by defining common properties and methods in a base class and extending this base class for each specific type of product.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.