Meaning Of Commonly Used Statments By Developers 🤔
Who Is The User Of The Class?
In the context of object-oriented programming (OOP), the "user" of a class is any entity that interacts with or utilizes that class. This can be another class, a function, or even a human developer.
Another Class or Object: In OOP, classes often interact with each other. One class may use another class by creating instances of it, invoking its methods, or accessing its properties. In this case, the class doing these actions is considered the "user" of the other class.
Functions or Methods: Functions or methods within a class or in the wider program may use a class. They may create objects of that class, call its methods, or manipulate its properties. These functions or methods are users of the class.
Developers: Developers write code, including classes, methods, and properties. When a developer uses a class in code they are writing, they are also considered a "user" of the class. This is a more meta perspective, but it's important to note because developers benefit from many features of OOP like encapsulation, inheritance, and polymorphism, which can make the class easier and safer to use.
In OOP, the idea of a "user" of a class is significant because one of the goals of OOP is to create code that is easy to understand, maintain, and reuse. By thinking about how a class will be used, developers can design their classes to be more user-friendly, whether the user is another part of the code or another developer.
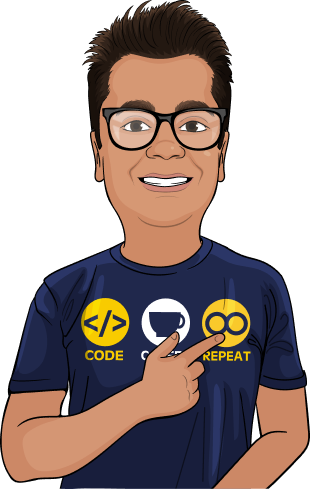
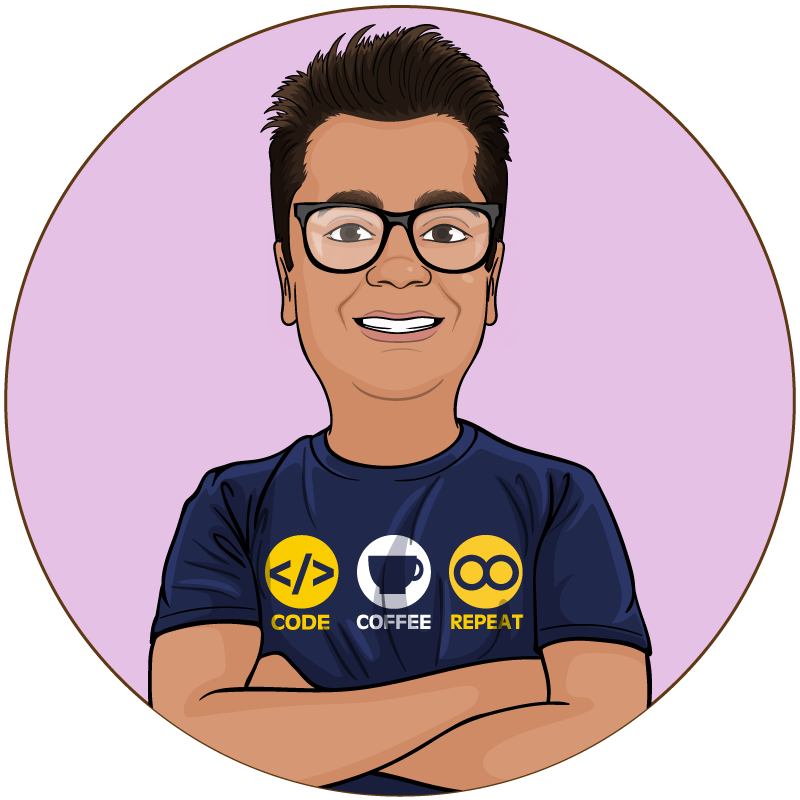
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSAn Interface
In the broad field of computer science, an interface often refers to the medium through which an individual interacts with a software or hardware system. It serves as a bridge between the user and the system, facilitating communication and interaction.
There are various types of interfaces in computing:
User Interface (UI): This is probably the most commonly thought-of type of interface. It's the space where interactions between humans and machines occur. The goal of this interaction is effective operation and control of the machine from the human end, while the machine simultaneously feeds back information that aids the operators' decision-making process. User interfaces can include graphical user interfaces (GUI), command-line interfaces (CLI), touch-based interfaces, voice-controlled interfaces, and many more.
Hardware Interface: These are the physical and logical arrangements that enable communication between devices and systems in a computer. For instance, the USB port on your computer is a type of hardware interface that allows it to communicate with USB devices.
Software Interface: This term is usually used in the context of software components (like libraries or modules) communicating with each other. A software interface defines the methods or functions that a component exposes to other components, defining how they should interact.
Network Interface: This refers to the software and hardware options that enable communication between networks. For example, a Network Interface Card (NIC) in a computer allows it to connect to a network.
Each of these interfaces serves as a contract or agreement, defining how interaction should occur and what form the exchange of information should take. The specifics of how this communication is carried out are usually hidden, allowing users (whether they're humans, software, or hardware devices) to focus on their interaction without worrying about the underlying complexities.
Relating Interfaces to Object-Oriented Programming
In software development, an interface is a shared boundary across which two separate components exchange information. It defines the communication between different parts of a software system and how they interact with each other.
In TypeScript and many other object-oriented programming languages, an interface is a syntax contract that an entity should conform to. Interfaces define properties, methods, and events, which are the members of the interface. Interfaces contain only the declaration of the members. It is the responsibility of the deriving class to define the members. They make it easy to ensure that a class adheres to a certain contract, helping with code organization and offering a level of abstraction.
Let's take a simple example where we define an interface Animal
and have two
classes Dog
and Cat
that implement this interface.
interface Animal {
name: string;
makeSound(): void;
}
class Dog implements Animal {
name: string;
constructor(name: string) {
this.name = name;
}
makeSound() {
console.log("Woof! Woof!");
}
}
class Cat implements Animal {
name: string;
constructor(name: string) {
this.name = name;
}
makeSound() {
console.log("Meow! Meow!");
}
}
let dog: Animal = new Dog("Spike");
dog.makeSound(); // Output: 'Woof! Woof!'
let cat: Animal = new Cat("Tom");
cat.makeSound(); // Output: 'Meow! Meow!'
In the above example, Animal
is the interface and Dog
and Cat
are the
classes that implement the Animal
interface. Both classes are obligated to
implement the name
property and the makeSound
method because they have been
specified in the interface.
In the main part of the code, you can see that Dog
and Cat
are treated
as Animal
. This is possible because of the interface. You can add more classes
that implement the Animal
interface and still treat them as Animal
, which
makes your code more flexible and extendable.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.