Awaited<Type> Utility Types in TypeScript With Examples
Awaited<Type>
is a utility type used to obtain the return type of a promise.
It unwraps the type inside a promise and returns the underlying type.
Syntax
The syntax consists of a single part:
type NewType = Awaited<Type>;
Awaited<Type>
: This is the TypeScript utility type itself. It takes one type parameter, which is theType
representing thePromise
from which you want to extract the resolved type.
Understanding Awaited<Type>
To understand this lets define a function that returns a promise.
const promise: Promise<string> = new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Resolving");
resolve("done!");
}, 1000);
});
We know that the above function will always return a promise and that
promise will always resolve to a string. We can use the Awaited<Type>
utility to create a type that will be the return type of the promise.
type AwaitedType = Awaited<typeof promise>;
Now if we check the type of AwaitedType
we will see that it is a string.
Nested Promises
Awaited<Type>
can also be used to unwrap nested promises. Lets convert our
promise function to return a promise that resolves to a promise that return
a number. So lets first decalre another fucntion called nestedPromise
that
returns a promise which eventually resolves to a number.
const nestedPromise: Promise<number> = new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Resolving");
resolve(5200);
}, 1000);
});
Now lets decalre another function that will act an an outer promise for the
nestedPromise
function.
const outerPromise: Promise<unknown> = new Promise((resolve, reject) => {
resolve(nestedPromise);
});
Now using the Awaited<Type>
utility we can get the return type of the
nestedPromise
function. So we can create another type called NestedType
that can be used to get the return type of the nestedPromise
function
using the Awaited<Type>
utility.
type NestedType = Awaited<Promise<typeof nestedPromise>>;
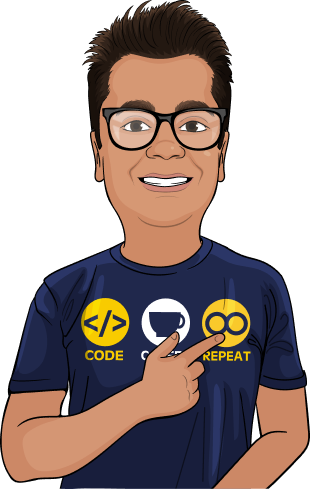
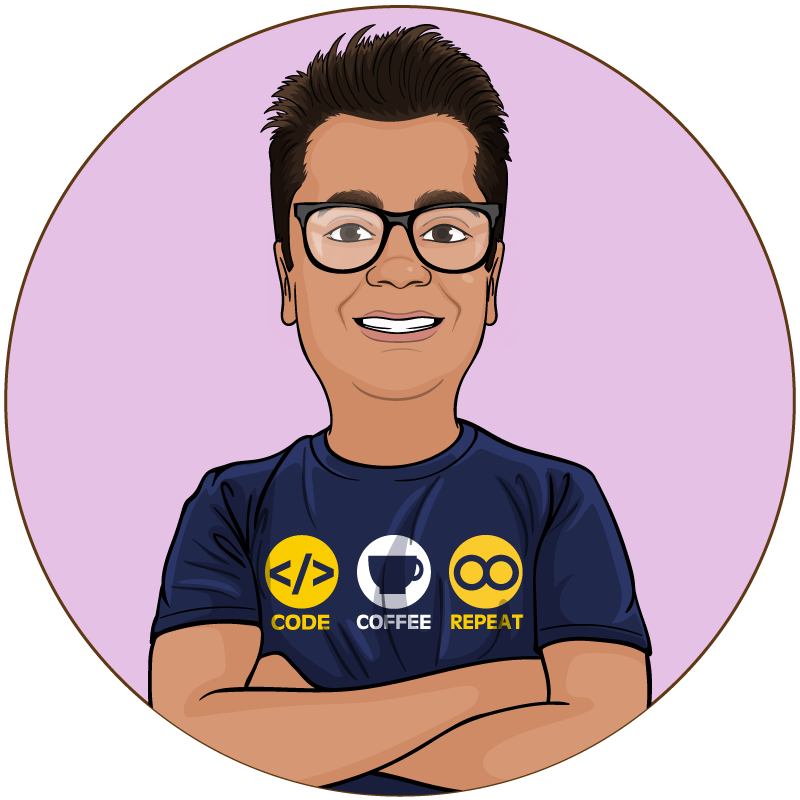
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhy is Awaited<Type>
Useful?
Lets look at a real work application scenario and assume that are working on a web application that uses a REST API to fetch data from a server and we are fetching userData from the sever.
async function fetchData(): Promise<{ name: string; age: number }> {
const response = await fetch("/api/user");
const data = await response.json();
return data;
}
Now whenever we need to use the fetchData
function we would not need to
redeclare the type of data returned by fetchData
function. We can use the
Awaited<Type>
utility to infer the return type of the data returned
by fetchData
function.
const userData: Awaited<typeof fetchUser> = fetchUser("string");
If we check the type of userData
we will see that it is of type
{ name: string; age: number }
. Awaited<Type>
utility is very useful for
asynchronous programming in TypeScript and working with promises.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.