ConstructorParameters<Type> Utility Types in TypeScript With Examples
What Is ConstructorParameters<Type>
In TypeScript, the ConstructorParameters<Type>
utility type is used to extract
the types of constructor arguments for a given class or constructor function. It
returns a tuple type containing the parameter types in the same order as they
are declared in the constructor. This utility type is particularly helpful when
you need to create a new instance of a class, pass constructor arguments
dynamically, or create higher-order functions or factories that work with
classes.
Syntax
The syntax for the ConstructorParameters<Type>
utility type is as follows:
type NewType = ConstructorParameters<T>;
- Here,
T
represents the class or constructor function whose constructor arguments types you want to extract. The utility type will return atuple
type containing the types of the constructor parameters in the order they are declared.
For example, consider the following class:
class Person {
constructor(public name: string, public age: number) {}
}
If you want to extract the constructor parameters types of the Person
class,
you
would use the ConstructorParameters
utility type like this:
type PersonConstructorArgs = ConstructorParameters<typeof Person>;
Now, PersonConstructorArgs
would be a tuple type equivalent
to [string, number]
.
This tuple type represents the types of the constructor arguments for
the Person
class in the order they are declared, which are a string for the name
and a
number for the age
.
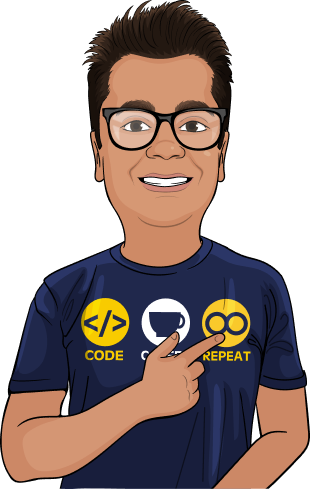
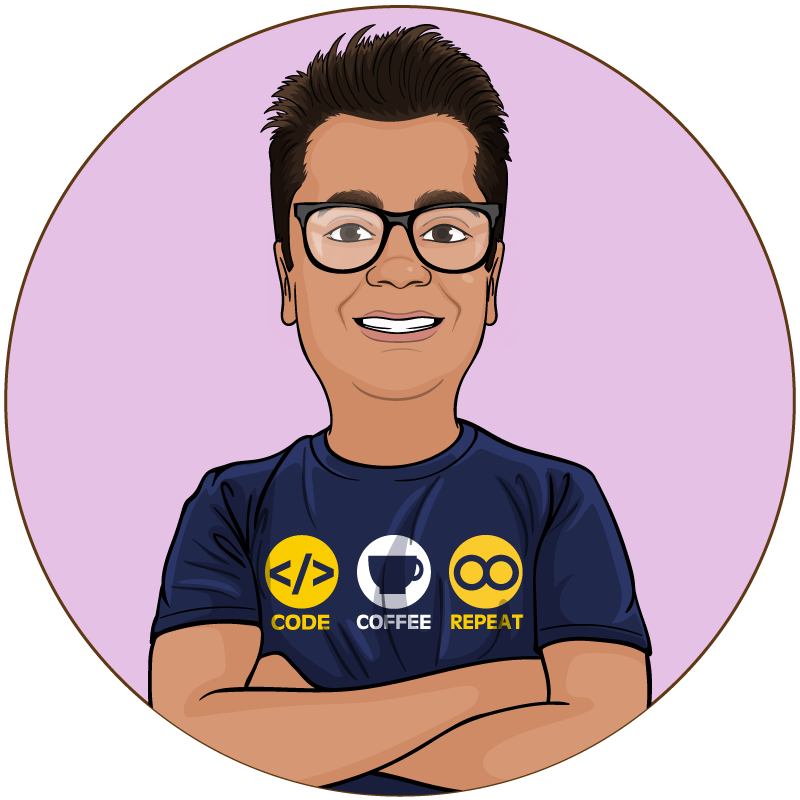
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Use Cases
In real-world applications, the ConstructorParameters<T>
utility type can be
particularly useful when you want to create a factory function that dynamically
generates instances of a class. Let's say we have a simple class hierarchy
representing shapes:
abstract class Shape {
constructor(public color: string) {}
abstract getArea(): number;
}
class Circle extends Shape {
constructor(color: string, public radius: number) {
super(color);
}
getArea(): number {
return Math.PI * this.radius * this.radius;
}
}
Now, we want to create a factory function that generates a new instance of a
given class with the provided constructor arguments. We can use the
ConstructorParameters<T>
utility type to achieve this:
function createInstance<T extends new (...args: any[]) => any>(
ClassConstructor: T,
...args: ConstructorParameters<T>
): InstanceType<T> {
return new ClassConstructor(...args);
}
Here, we define a generic factory function createInstance
that accepts a class
constructor ClassConstructor
and its constructor arguments ...args
. We use
the
ConstructorParameters<T>
utility type to specify that the args
parameter
should
be a tuple type containing the types of the constructor parameters of the class
represented by T
.
Now, we can use this factory function to create new instances of the Circle
class:
const circle = createInstance(Circle, "red", 5);
console.log(circle.getArea()); // 78.53981633974483
In this example, we call createInstance
with the Circle
class constructor, a
color
string, and a radius
number. The factory function creates a new Circle
instance with the provided arguments and returns it. The
ConstructorParameters<T>
utility type ensures that we pass the correct types of
constructor arguments for the given class.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.