Parameters<Type> Utility Types in TypeScript With Examples
What Is Parameters<Type>
The Parameters<Type>
utility type in TypeScript helps you extract the types of
the parameters of a function. This is useful when you want to reuse the
parameter types of a function in another part of your code, without having to
manually write the types again.
Parameters<Type>
extracts the parameter types of a
function
type Type
and represents them as a tuple
type. This is useful
when you
want to reference the parameter types of a function in other parts of your code
without explicitly defining them.
Syntax
Here's the basic syntax for using Parameters<Type>
type NewType = Parameters<Type>;
Here's a breakdown of the syntax:
type
: A TypeScript keyword used to create a new type alias.NewType
: The name of the new type alias you are creating.=
: The assignment operator, used to assign the extracted parameter types to the new type alias.Parameters<Type>
: The built-in utility type Parameters that takes a function typeType
as an argument and extracts its parameter types as atuple
.
Here's an example to illustrate this concept:
// Define a function type
type MyFunction = (x: number, y: string) => void;
// Use Parameters to create a new type
// alias representing the parameters of MyFunction as a tuple
type MyFunctionParameters = Parameters<MyFunction>;
// MyFunctionParameters is now equivalent
// to the tuple type [number, string]
In this example, MyFunctionParameters
will have the type [number, string]
,
which
is the parameter types of the function type MyFunction
as a tuple.
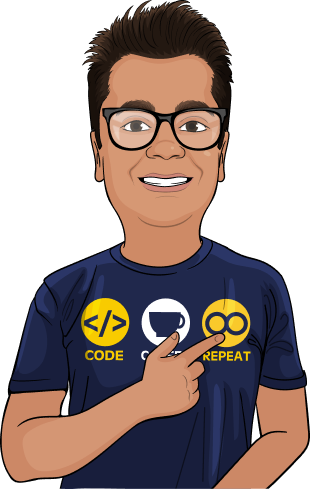
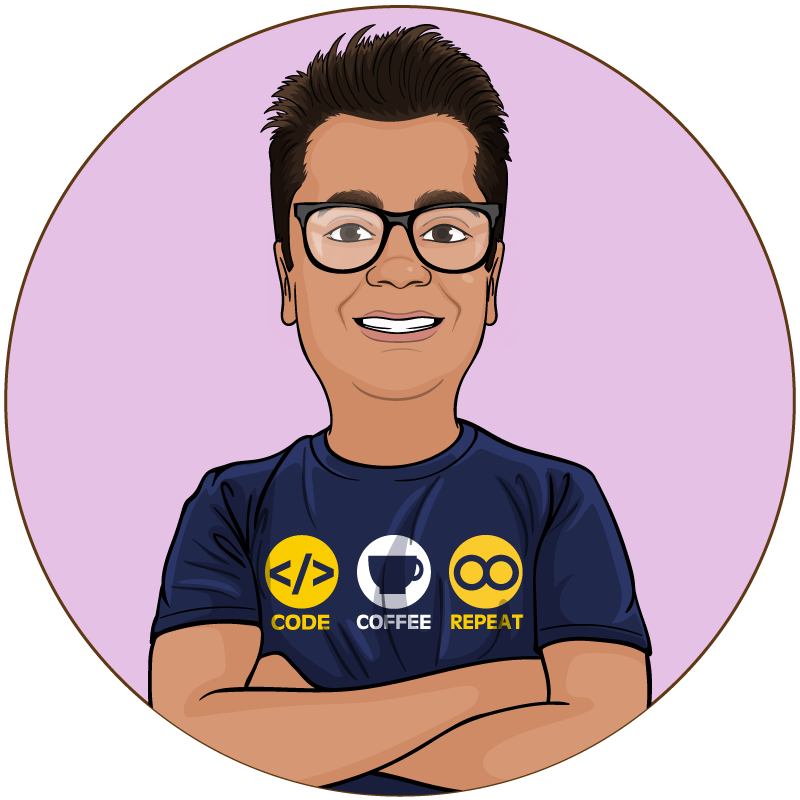
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal World Use Case
A real-life application use case of Parameters<Type>
in TypeScript is when you
want to create a higher-order function that wraps another function and adds
additional functionality while maintaining type safety. By using
Parameters<Type>
, you can ensure that your wrapper function accepts the same
parameters as the original function without explicitly defining them.
Let's say you're working on an application that logs the time taken for various operations. You have several functions that perform different tasks, and you want to create a generic function that wraps these tasks and logs their execution time.
// Dummy function to simulate fetching data from a URL
function fetchData(url: string): Promise<string> {
return new Promise((resolve) => {
setTimeout(() => {
// Simulate fetching data from the given URL
const data = `Data fetched from ${url}`;
resolve(data);
}, 1000); // Simulate a 1-second delay
});
}
In the fetchData
function, a new Promise
is created, which uses setTimeout
to
simulate a 1-second delay. After the delay, the promise resolves with a string
representing the fetched data. In a real-world scenario, you would replace this
mocked implementation with actual data-fetching logic using libraries like fetch
or axios.
function processFile(path: string, encoding: string): string {
// Implementation that processes a file and returns a string
// In a real-world scenario, you would replace this
// mocked implementation with actual file processing logic
// For the sake of example, we'll create a dummy string
// based on the provided path and encoding
const result = `Processed file at path "${path}" with encoding "${encoding}"`;
return result;
}
In this example, the processFile
function takes a path and an encoding as
arguments and returns a dummy string representing the processed file. In a
real-world scenario, you would use the fs
module in Node.js or other file
processing libraries to read and process the file based on the given parameters.
Nowe can create our higher order function that measures the execution time of the obove functions.
// Type-safe higher-order function to measure execution time
function measureExecutionTime<T extends (...args: any[]) => any>(
func: T,
...args: Parameters<T>
): ReturnType<T> {
const startTime = Date.now();
const result = func(...args);
const endTime = Date.now();
console.log(`Execution time for ${func.name}: ${endTime - startTime} ms`);
return result;
}
// Usage
const fetchDataResult = measureExecutionTime(
fetchData,
"https://example.com/data.json"
);
const processFileResult = measureExecutionTime(
processFile,
"./file.txt",
"utf-8"
);
In the provided example, measureExecutionTime
is a type-safe higher-order
function that measures the execution time of any function passed to it. By using
the Parameters<T>
utility type, we can ensure that the wrapper function accepts
the same parameters as the original function without explicitly defining
them as separate types.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.