Pick<Type, Keys> Utility Types in TypeScript With Examples
Pick<Type, Keys>
is a TypeScript utility type that creates a new type by
picking a subset of properties from an existing type. The Type
parameter
is the original type, and the Keys
parameter is an array of property names
to pick from the original type. The resulting type contains only the properties
specified by the Keys
parameter.
Syntax
Here's an example of how to use Pick<Type, Keys>
:
type NewType = Pick<Type, Keys>;
The syntax consists of two parts:
Pick<Type, Keys>
: This is the TypeScript utility type itself. It takes two type parameters: the first is the Type from which you want to pick properties, and the second is the Keys representing the properties you want to pick.Type
: This is the existing type from which you want to pick specific properties.Keys
: This is a union of string literal types representing the keys of the properties you want to pick from the Type. The keys are combined using the|
(pipe) symbol.
Understanding Pick<Type, Keys>
To understand this lets create an interface called Person
.
interface Person {
name: string;
age: number;
address: string;
}
type NameAndAge = Pick<Person, "name" | "age">;
const person: NameAndAge = {
name: "Alice",
age: 30,
};
In this example, we have an interface called Person
with three
properties: name
,
age
, and address
. We then create a new type called NameAndAge
by
using Pick<
Person, 'name' | 'age'>
. This creates a new type that contains only the name
and
age properties from the Person
interface. Finally, we create an object of type
NameAndAge
and assign it to a variable called person
.
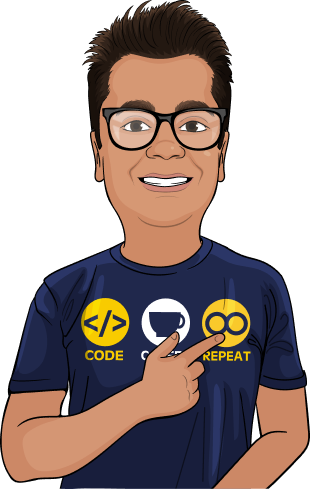
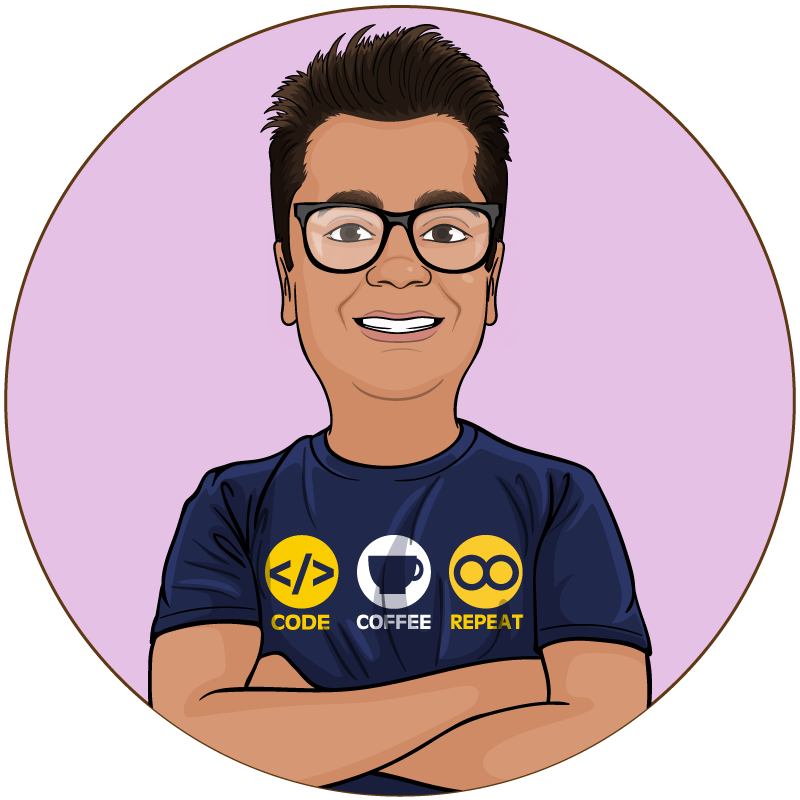
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-world use cases
Now that we understand what Pick<Type, Keys>
is and how it works, let's
look at some real-world use cases for this utility type.
Creating smaller, more specialized types
One common use case for Pick<Type, Keys>
is to create smaller, more
specialized types from larger, more general types. For example, suppose we have
an interface called Order
that represents an order for a product:
interface Order {
id: number;
product: string;
quantity: number;
price: number;
shippingAddress: string;
billingAddress: string;
}
This interface contains several properties,
including id
, product
, quantity
,
price
, shippingAddress
, and billingAddress
. However, suppose we only
need to work with the id
, product
, quantity
, and price
properties in our
application. We can create a new type that contains only these properties by
using Pick<Order, 'id' | 'product' | 'quantity' | 'price'>
:
type OrderSummary = Pick<Order, "id" | "product" | "quantity" | "price">;
This creates a new type called OrderSummary
that contains only
the id
, product
, quantity
, and price
properties from the Order
interface. We can now use this new type throughout our application whenever we
only need to work with these four properties.
Extracting Properties From Complex Types
Another use case for Pick<Type, Keys>
is to extract properties from
complex types. Suppose we have an interface called Car
that represents a
car:
interface Car {
make: string;
model: string;
year: number;
engine: {
displacement: number;
horsepower: number;
};
color: string;
}
This interface contains a property called engine
that is itself an object with
two properties: displacement
and horsepower
. Suppose we only need to work
with
the horsepower
property of the engine object. We can extract this property by
using Pick<Type, Keys>:
type CarEngine = Pick<Car["engine"], "horsepower">;
This creates a new type called CarEngine
that contains only the horsepower
property from the engine object of the Car
interface. We can now use this new
type whenever we need to work with the horsepower
property of a car's engine.
Creating read-only versions of types
Finally, another use case for Pick<Type, Keys>
is to create read-only
versions of types. Suppose we have an interface called User
that
represents a user:
interface User {
id: number;
name: string;
email: string;
password: string;
}
User
interface contains several properties, including id
, name
, email
,
and password
. However, suppose we want to create a read-only version of
User
interface that we can use in certain parts of our application where
we only need to read user data. We can create a read-only version of this
interface
by using Pick<Type, Keys>
in combination with the Readonly<Type>
utility
type:
type ReadOnlyUser = Readonly<Pick<User, "id" | "name" | "email">>;
This creates a new type called ReadOnlyUser
that is read-only and contains
only the id
, name
, and email
properties from the User
interface. We can
now use this new type throughout our application whenever we only need to read
user data.
Conclusion
In conclusion, the Pick<Type, Keys>
utility type in TypeScript is a
powerful tool for creating new types that are subsets of existing types. We have
seen how to use Pick<Type, Keys>
to create smaller, more specialized
types, extract properties from complex types, and create read-only versions of
types. By using Pick<Type, Keys>
in our TypeScript code, we can write more
concise and maintainable code that is easier to reason about and debug.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.