Omit<OldType, Keys> Utility Types in TypeScript With Examples
We already looked
at Pick<Type, Keys>
is a TypeScript utility type that creates a new type by picking a subset of
properties from an existing type.
The Omit<OldType, Keys>
utility type is the opposite of Pick<Type, Keys>
.
It helps us create a new type by omitting a subset of properties from an
existing type.
Understanding Omit<OldType, Keys>
Here's an example of how to use Omit<OldType, Keys>
Syntax
The syntax for the Omit utility type is as follows:
type NewType = Omit<OldType, Keys>;
- In this syntax,
OldType
is the original type that has the properties you want to omit, andKeys
is a union of the property names you want to omit. - The resulting type,
NewType
, is a new type that has all the properties ofOldType
except the ones specified in Keys.
Example
To better understand how the Omit utility type works, let's use the Todo
type
from the previous example:
interface Todo {
title: string;
description: string;
completed: boolean;
}
Suppose you want to create a new type that omits the description
property. You
can use the Omit utility type as follows:
type TodoWithoutDescription = Omit<Todo, "description">;
The resulting type TodoWithoutDescription
is a new type that has all the
properties of Todo
, except for description
.
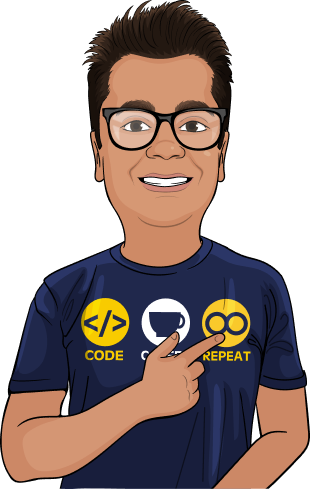
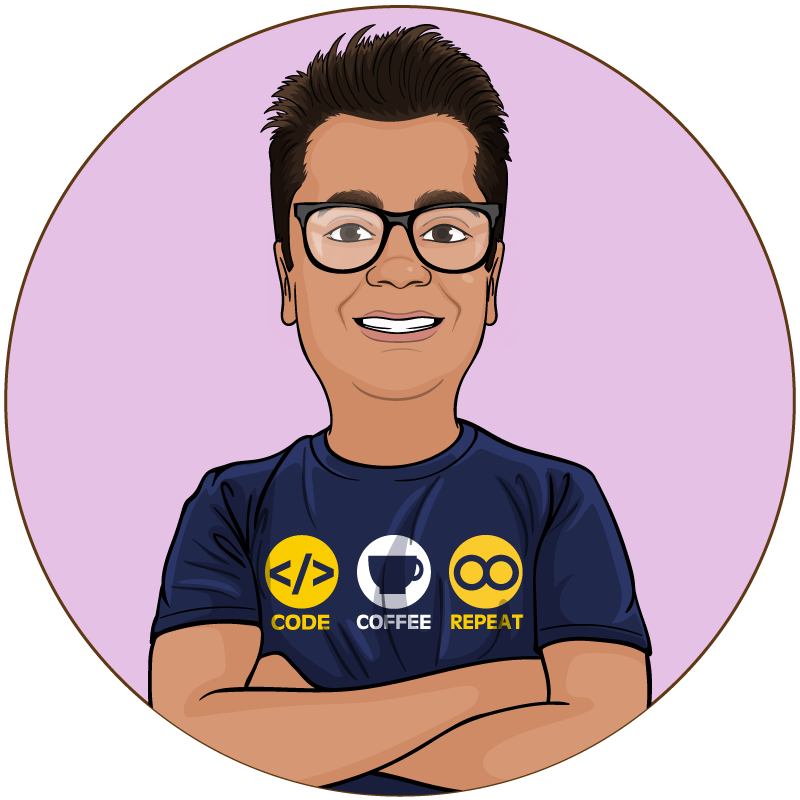
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Use Cases
The Omit utility type can be useful in many situations. Let's take a look at a few examples:
Forms
When building forms, you might want to create a type that omits certain
properties from an existing type. For example, suppose you have a User
type
with many properties, but you only want to use a few of them in a form. You can
use the Omit utility type to create a new type that omits the properties you
don't need:
interface User {
name: string;
age: number;
email: string;
phone: string;
address: string;
}
type UserForm = Omit<User, "phone" | "address">;
In this example, we create a new type UserForm
that omits the phone
and address
properties from the User
type. This makes it easier to define the properties
that are needed for the form, and in turn, makes it easier to manage the form
data.
Removing sensitive data from objects
In many applications, we may have objects that contain sensitive data, such as passwords or credit card numbers. We don't want this data to be sent to the client, so we need to remove it from the object before sending it. We can use the Omit utility type to create a new object that omits the sensitive data.
For example, let's say we have a user object with a password property:
interface User {
name: string;
email: string;
password: string;
}
We want to remove the password property from the user object before sending it to the client. We can do this using the Omit utility type:
type SafeUser = Omit<User, "password">;
Now we can create a new object that only contains the name and email properties of the user object:
Component Props
When building React components, you might want to create a type that omits
certain props from an existing type. For example, suppose you have a Button
component that takes many props, but you only want to use a few of them in a
specific context. You can use the Omit
utility type to create a new type that
omits the props you don't need:
interface ButtonProps {
text: string;
color: string;
size: string;
onClick: () => void;
disabled: boolean;
}
type PrimaryButtonProps = Omit<ButtonProps, "color" | "size">;
In this example, we create a new type PrimaryButtonProps that omits the color and size props from the ButtonProps type. This makes it easier to create a button that has a specific style or behavior.
Conclusion
The Omit utility type is a powerful feature of TypeScript that lets you create new types by omitting specific properties from an existing type. This can be useful in many situations, such as when building forms, React components, or when filtering data.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.