Understanding Utility Types in TypeScript
Utility types are predefined generic types in TypeScript that can be used to manipulate other types and crate new types from other types.
What are Utility Types?
Utility types are predefined generic types that can perform common type transformations in TypeScript. These types are built into TypeScript and are used to manipulate other types and create new types. Utility types can create new types by applying transformations to existing types. They are a powerful feature of TypeScript and help avoid code duplication and make it easier to write generic code.
Some Examples of Utility Types
Here are some examples of utility types in TypeScript:
Partial<T>
- This type creates a new type that is a partial version of an existing type T. This means that all properties of T become optional. This is useful when working with interfaces that have many properties, but only some of them are required.Readonly<T>
- This type creates a new type that is a read-only version of an existing type T. This means that all properties of T become read-only. This is useful when working with interfaces that should not be modified after creation.Pick<T, K>
- This type creates a new type by picking only the specified properties K from an existing type T. This is useful when working with interfaces that have many properties, but only some of them are needed.Exclude<T, U>
- This type creates a new type by excluding all properties of U from an existing type T. This is useful when working with interfaces that have overlapping properties.Omit<T, K>
- This type creates a new type by omitting the specified properties K from an existing type T. This is useful when working with interfaces that have many properties, but some of them are not needed.Required<T>
- This type creates a new type that is a required version of an existing type T. This means that all properties of T become required. This is useful when working with interfaces that require all properties to be defined.
There are many more utility types offered by TypeScript, and we will be talking about each of these utility types in our series of articles on utility types in TypeScript.
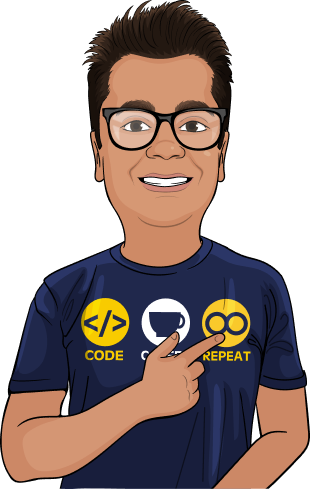
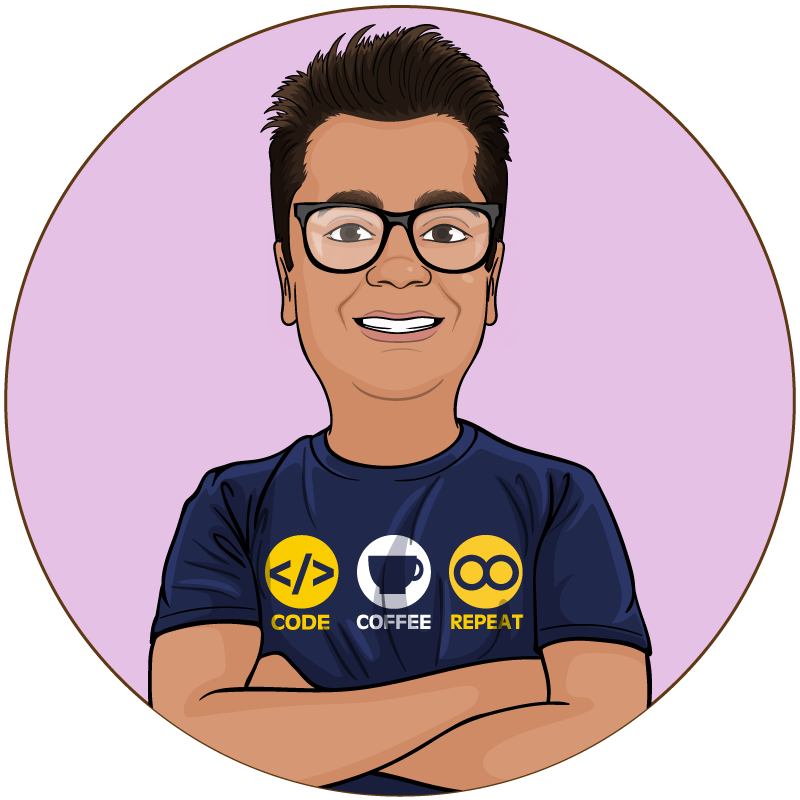
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSBenefits Utility Types
Here are a few benefits of working with utility types in TypeScript, ranging from ease of writing code to allowing developers to write more generic and readable code. Let's have a look at the advantages one by one.
- Utility types help create new types by transforming existing types, which helps avoid code duplication.
- It helps developers in writing generic code that works with many different types.
- Improve code readability and maintainability by creating types that accurately describe the data used.
- Utility types can be considered a library of generic types available for developers to use out of the box. Utility types have been developed, keeping in mind the most common use cases a developer would encounter while working with types in TypesScript.
Since we now understand what utility types are, let's look at how utility types work behind the scenes and how they are coded or created using various features of TypeScript itself in the next article.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.