String Manipulation Utility Types in TypeScript With Examples
String Manipulation Utility Types
In this article, we will explore a
collection of utility types specifically designed to manipulate string
literals: Uppercase<StringType>
, Lowercase<StringType>
, Capitalize<StringType>
,
and Uncapitalize<StringType>
.
These utility types allow developers to transform string literal types in different ways, ultimately improving the type safety and expressiveness of your code. By using these utilities, you can ensure that your code adheres to specific casing requirements, making it more robust and less prone to bugs caused by incorrect string capitalization.
Here's a brief overview of each utility type:
Uppercase<StringType>
: This utility type transforms a given string literal type to its uppercase equivalent. It is particularly useful when working with constants or enumerated values that require uppercase representation.Lowercase<StringType>
: Similar to Uppercase, the Lowercase utility type converts a string literal type to its lowercase counterpart. This can be helpful when dealing with case-insensitive comparisons or user input validation.Capitalize<StringType>
: This utility type capitalizes the first letter of a string literal type while keeping the remaining characters unchanged. Capitalize can be handy when dealing with titles, proper nouns, or any situation where you want to ensure the correct capitalization of a string.Uncapitalize<StringType>
: As the inverse of Capitalize, this utility type uncapitalizes the first letter of a given string literal type, leaving the rest of the string intact. This can be useful when standardizing data or formatting output.
In the following sections, we will dive deeper into the practical usage of these string manipulation utility types, providing code examples and demonstrating their benefits in TypeScript applications. By leveraging these utilities, you can make your code resistant to potential errors caused by improper string casing.
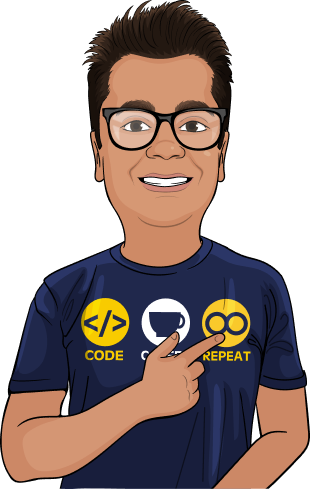
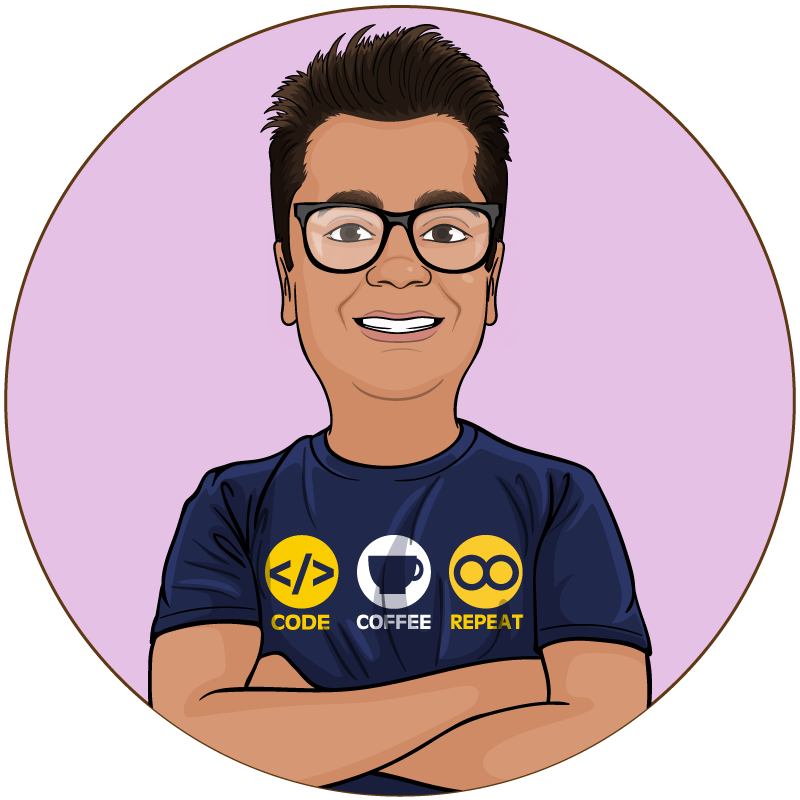
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Use Cases
While the examples provided in the article showcase the use of TypeScript's string manipulation utility types with a limited number of string literals, their true power becomes evident when dealing with large lists of string values. In real-world applications, developers often face the challenge of managing and type-checking extensive sets of string constants, such as long lists of country or city names, product codes, or user roles.
By employing these utility types, you can effectively transform, standardize, and enforce casing rules on extensive lists of string literals at compile time. Consequently, you can catch potential errors and inconsistencies early in the development process and significantly enhance the type safety and robustness of your code. This increased type safety allows you to maintain high-quality code and improve overall application performance, particularly when managing large-scale projects.
Uppercase<StringType>
Imagine you are building a weather application that uses an API that accepts city names in uppercase format. To ensure proper input, you can use the Uppercase utility type to convert user input to uppercase before making an API request.
type City = "new york" | "london" | "tokyo";
type UppercaseCity = Uppercase<City>;
// Now UppercaseCity will have the
// type "NEW YORK" | "LONDON" | "TOKYO"
Lowercase<StringType>
Suppose you are creating an application that manages a list of email addresses.
Since email addresses are case-insensitive, you can use the Lowercase
utility
type to standardize all stored email addresses in lowercase format.
type Email = "JohnDoe@example.com" | "JaneDoe@example.COM";
type LowercaseEmail = Lowercase<Email>;
// Now LowercaseEmail will have the
// type "johndoe@example.com" | "janedoe@example.com"
Capitalize<StringType>
Imagine you are developing a blogging platform where the titles of the blog
posts need to be capitalized. You can use the Capitalize
utility type to
ensure
that the first letter of each title is capitalized.
type BlogTitle = "a day in the life" | "my trip to london";
type CapitalizedTitle = Capitalize<BlogTitle>;
// Now CapitalizedTitle will have the
// type "A day in the life" | "My trip to london"
Uncapitalize<StringType>
Consider a situation where you are building an application to manage a database of book titles. Some titles may have been entered with inconsistent capitalization. You can use the Uncapitalize utility type to standardize the first letter of each title to lowercase.
type BookTitle = "The Catcher In The Rye" | "To Kill A Mockingbird";
type UncapitalizedTitle = Uncapitalize<BookTitle>;
// Now UncapitalizedTitle will have the
// type "the Catcher In The Rye" | "to Kill A Mockingbird"
Conclusion
In conclusion, TypeScript's string manipulation utility types offer developers powerful tools for working with string literals. As a result, the utility types play a vital role in enhancing the already impressive type system of TypeScript, further solidifying its position as a go-to language for building large-scale applications.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.