Required<Type> Utility Types in TypeScript With Examples
What is Required<Type>
The Required<Type>
utility type is used to create a new type that makes all
properties of the original type required. This means that any optional
properties become required properties.
Syntax
Here is the what the basic sytax looks like:
type NewType = Required<Type>;
Required<Type>
: This is the TypeScript utility type itself. It takes one type parameter, which is theType
for which you want to set all properties as required.
Developers can use the Required<Type>
utility type in their TypeScript
applications to ensure that certain properties of an object are always defined.
Here are some real-life code examples:
Example Use Case In an eCommerce Application
Suppose you are building an application that tracks orders and you have the following interface for an order:
interface Order {
orderId?: number;
customerName?: string;
orderDate?: Date;
orderTotal?: number;
}
In this case, all properties of the Order
interface are optional. However, you
want to ensure that all the properties Order
type are always defined. You can
use theRequired<Type>
s utility type to create a new type that makes these
properties required as follows:
type RequiredOrder = Required<Order>;
let order: RequiredOrder = {
orderId: 1,
customerName: "John Doe",
orderDate: new Date(),
orderTotal: 100,
};
In this example, the RequiredOrder type makes all properties of the Order interface required, so you can create an order object with all required properties.
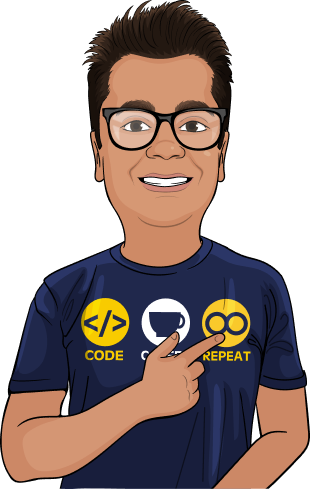
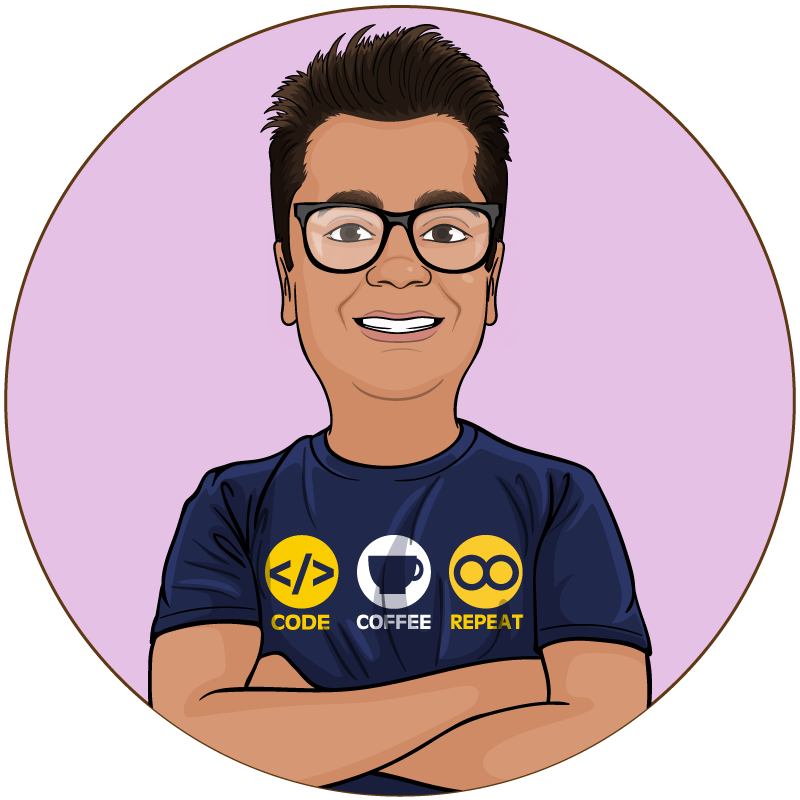
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSCombining Required<Type>
with Pick<Type,Keys>
Suppose you are building an application that manages user profiles and you have the following interface for a user:
interface User {
name?: string;
age?: number;
email?: string;
address?: string;
}
In this case, all properties of the User
interface are optional. However, you
want to ensure that the name
and email
properties are always defined. You
can use the Required<Type>
utility type to create a new type that makes these
properties required as follows:
type RequiredUser = Required<Pick<User, "name" | "email">>;
let user: RequiredUser = {
name: "John Doe",
email: "johndoe@example.com",
};
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.