Partial<Type> Utility Types in TypeScript With Examples
What is Partial<Type>
The Partial<Type>
type is a built-in utility type in TypeScript that allows
you
to create a new type based on an existing type, but with all its properties set
to optional. This means that when you use the Partial<Type>
type, you can
create
a new type that has all the same properties as the original type, but you can
omit any of those properties if you need to.
Syntax
The Partial<Type>
type is a utility type that takes an existing type as its
parameter. This is how the basic syntax looks like:
type NewType = Partial<Type>;
Partial<Type>
: This is the TypeScript utility type itself. It takes one type parameter, which is the Type for which you want to set all properties as optional.
For example, if you have an interface called Person
with three
properties, you can create a new type called PartialPerson
that has all the
same
properties, but with each property set to optional.
interface Person {
name: string;
age: number;
email: string;
}
type PartialPerson = Partial<Person>;
const person: PartialPerson = {
name: "John",
age: 30,
};
In this example, we defined an interface called Person
with three properties:
name
, age
, and email
. We then created a new type called PartialPerson
using the
Partial<Person>
type. This means that PartialPerson
has all the same
properties
as Person
, but they are all optional.
We then created an object called person
of type PartialPerson
. Notice that
we
only set the name and age properties but not the email property. Since
PartialPerson
has all its properties set to optional, this is a perfectly
valid
TypeScript code.
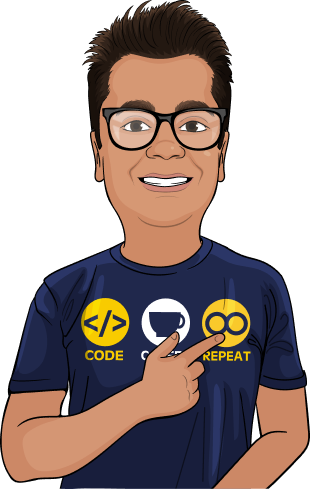
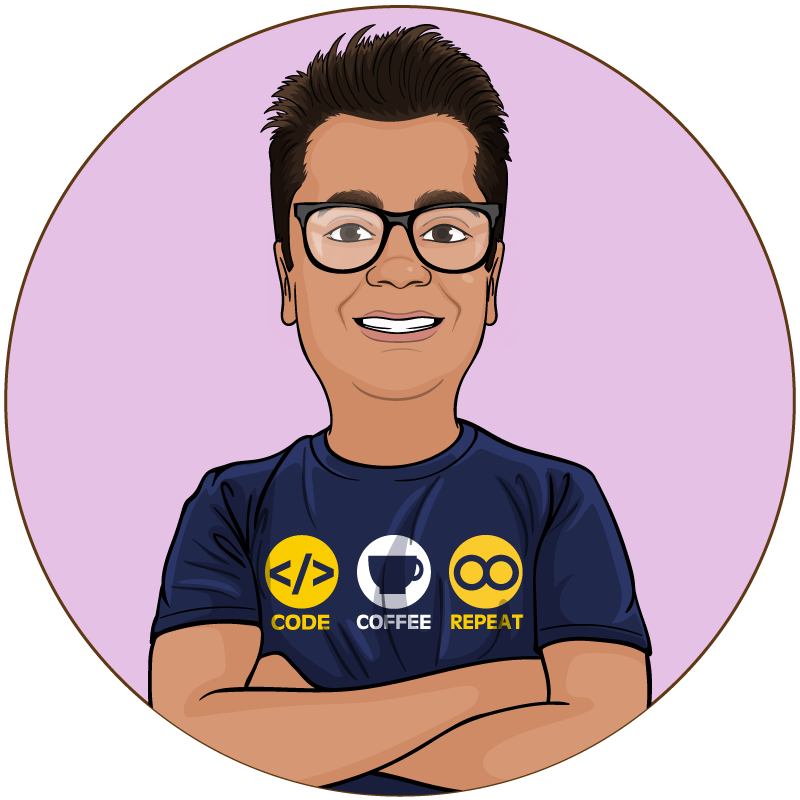
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Use Cases
So, how can we use the Partial<Type>
type in real-world applications? Here are
a few examples:
Updating an Object
Often, you may need to update an object in your application, but you don't want
to replace the entire object. Instead, you just want to update some of its
properties. In this case, you can use the Partial<Type>
type to create a new
object that has all the same properties as the original object, but with some of
them updated.
interface User {
name: string;
email: string;
password: string;
}
function updateUser(user: User, updates: Partial<User>): User {
return { ...user, ...updates };
}
const user: User = {
name: "John",
email: "john@example.com",
password: "123456",
};
const updatedUser = updateUser(user, { email: "new-email@example.com" });
In this example, we defined an interface called User
with three
properties: name
, email
, and password
. We then defined a function
called updateUser
that takes a User
object and a Partial<User>
object as
parameters. The Partial<User>
object represents the updates that we want to
apply to the User
object.
Inside the updateUser
function, we use the spread operator (...
) to create a
new object that has all the properties of the original User
object, but with
the properties of the Partial<User>
object overriding the original properties.
We then created a User
object called user
, and called updateUser
to update
its email address. The result is a new User
object called updatedUser
with
the same name and password, but with the email address updated.
Conclusion
The Partial<Type>
type is a powerful utility type in TypeScript that allows
you to create new types with optional properties. It has a variety of use cases,
from updating objects to defining optional props in React components. By
understanding the Partial<Type>
type, you can write more flexible and
maintainable code in your TypeScript applications.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.