ReturnType<Type> Utility Types in TypeScript With Examples
What Is ReturnType<Type>
ReturnType<Type>
is a utility type in TypeScript that extracts the return type
of a function type. It takes a function type as its input and produces the type
of the function's return value. This is useful when you want to use the return
type of a function in other parts of your code without explicitly defining it,
enabling better type inference and code maintainability.
Syntax
Here is the basic syntax for ussing the ReturnType<Type>
utility type
type NewType = ReturnType<Type>;
Here's a breakdown of the syntax:
type
: A keyword used to declare a type alias.NewType
: The name of the new type alias you want to create.=
: Assignment operator used to assign the type on the right-hand side to the new type alias.ReturnType<Type>
: The utility type that extracts the return type of the function type Type.
Using this syntax, you can create a new type that represents the return type of a function, which can be useful for better type inference and code maintainability.
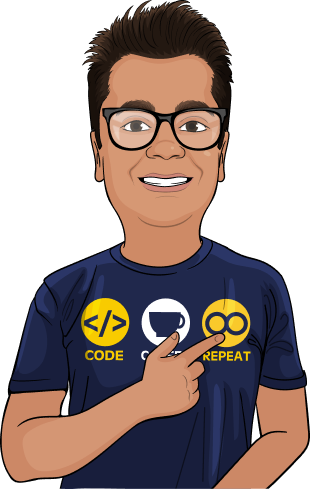
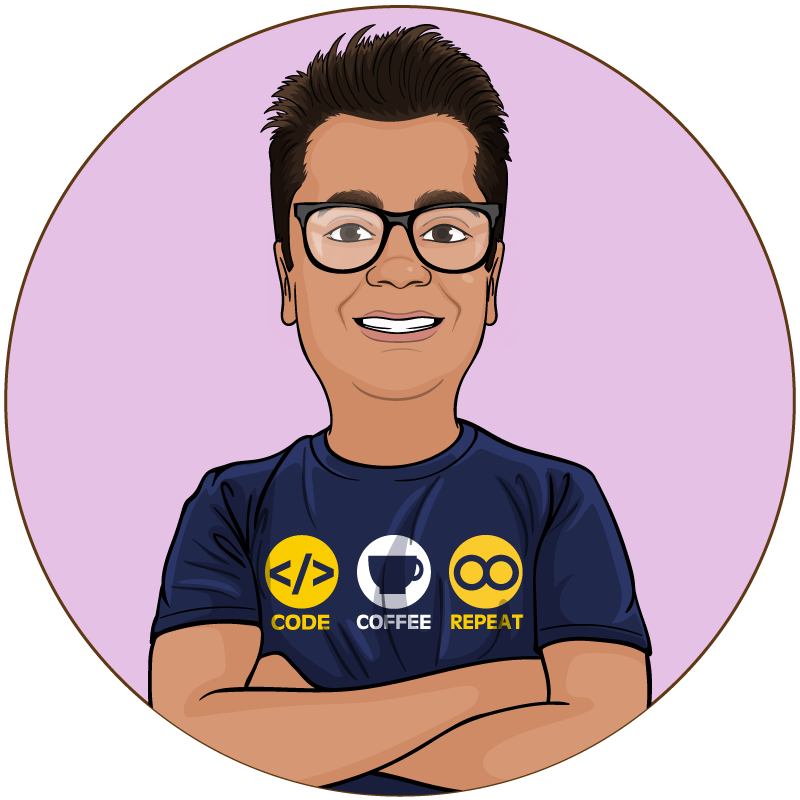
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSReal-World Use Cases
Let's consider a real-world example where the ReturnType<Type>
utility type
can
be useful in a TypeScript application. Imagine we have an e-commerce application
where we need to fetch product data from an API and perform some operations on
it.
First, we define a function fetchProduct
that fetches the product data from
the
API:
async function fetchProduct(id: number): Promise<{
id: number;
name: string;
price: number;
}> {
// Fetch product data from the API and return it as an object.
// For simplicity, we're returning a hardcoded object here.
return { id, name: "Sample Product", price: 100 };
}
Now, we want to create a function processProduct
that takes the product data
returned by fetchProduct
as an argument and performs some operations. Instead
of
explicitly defining the type for the argument, we can use ReturnType<Type>
to
infer it:
// Using the ReturnType utility type to create a
// type alias for the returntype of fetchProduct
type ProductData = ReturnType<typeof fetchProduct> extends Promise<infer T>
? T
: never;
async function processProduct(product: ProductData) {
// Perform some operations on the product data.
console.log(`Processing product: ${product.name}`);
}
// Usage
fetchProduct(1).then((product) => {
processProduct(product);
});
We use the ReturnType<typeof fetchProduct>
to create a new type alias
ProductData
. We also use a conditional type to unwrap the Promise
and get the
actual object type. Now, the processProduct
function takes an argument of
type ProductData
.
What Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.