Use Cases For The Chain of Responsibility Pattern
The Chain of Responsibility pattern can be quite useful in a variety of real-world scenarios
Event Propagation in Graphical User Interfaces (GUIs)
Many GUI frameworks use a form of the Chain of Responsibility pattern to propagate events. When a user interacts with a graphical component (like clicking a button), an event is generated. This event is then propagated up through the nested components (from the button to its parent panel, to the parent window, and so on) until it's handled. This allows individual components to either handle the event, or ignore it and allow a parent component to handle it. This use of the Chain of Responsibility pattern allows for flexible and dynamic event handling in GUIs.
Here is a simple example of how the Chain of Responsibility pattern can be represented in the context of event propagation in a Graphical User Interface ( GUI) application using a class diagram:
In this diagram:
GUIComponent
is an abstract base class that all GUI components inherit from. It declares thehandleEvent
method and thesetNext
method for setting the next handler in the chain.Button
,Panel
, andWindow
are concrete components that inherit fromGUIComponent
. Each component knows how to handle the event or pass it up to its parent in the chain.- The arrow from
GUIComponent
toButton
,Panel
, andWindow
signifies that these components inherit fromGUIComponent
.
This is a simplification and does not include every detail of how actual GUI systems work, but it gives a sense of how the Chain of Responsibility pattern might be used in a GUI system to propagate events.
Middleware in Web Development Frameworks
Middleware is a common feature in many web development frameworks (like Express.js for Node.js). Middleware functions are functions that have access to the request object, the response object, and the next function in the application's request-response cycle. This next function can be seen as the next link in the Chain of Responsibility. Each middleware function can either end the request-response cycle, or pass control to the next middleware function. This allows for modular and flexible handling of HTTP requests.
Here is a class diagram that represents how the Chain of Responsibility pattern might be used in middleware in a web development framework context:
In this diagram:
Middleware
is an abstract base class that all middleware inherit from. It declares thehandleRequest
method and thesetNext
method for setting the next handler in the chain.AuthenticationMiddleware
,LoggingMiddleware
, andRouteHandlerMiddleware
are concrete middleware that inherit fromMiddleware
. Each middleware knows how to handle the request or pass it to the next middleware in the chain.- The arrow from
Middleware
toAuthenticationMiddleware
,LoggingMiddleware
, andRouteHandlerMiddleware
signifies that these middleware inherit fromMiddleware
.
This is a simplification and does not include every detail of how actual web development frameworks work, but it gives a sense of how the Chain of Responsibility pattern might be used in a middleware context to handle HTTP requests.
Input/Event Handling in Game Development
In game development, the Chain of Responsibility pattern can be used to handle user input or game events. This allows different game objects or systems to handle the input or event as needed. For example, a game might have a chain of input handlers, where each handler checks if the input is relevant to it (like a specific keystroke or mouse movement). If a handler can't handle the input, it passes it along to the next handler.
In this diagram:
InputHandler
is an abstract base class that all input handlers inherit from. It declares thehandleInput
method and thesetNext
method for setting the next handler in the chain.PlayerInputHandler
,AIInputHandler
, andNetworkInputHandler
are concrete input handlers that inherit fromInputHandler
. Each input handler knows how to handle the input or pass it to the next handler in the chain.- The arrow from
InputHandler
toPlayerInputHandler
,AIInputHandler
, andNetworkInputHandler
signifies that these input handlers inherit fromInputHandler
.
This is a simplification and does not include every detail of how actual game development works, but it gives a sense of how the Chain of Responsibility pattern might be used in a game development context to handle user inputs or game events.
Logging Frameworks
Logging frameworks often use a form of the Chain of Responsibility pattern to propagate log messages. Loggers can be arranged in a hierarchy, and each logger can decide whether to handle a log message or to propagate it up the hierarchy. This allows for flexible and configurable logging, where each logger can be set to handle a certain level of log messages (like ERROR, WARNING, INFO, etc.) and to propagate other messages.
In this diagram:
Logger
is an abstract base class that all loggers inherit from. It declares thelogMessage
method and thesetNext
method for setting the next handler in the chain.ConsoleLogger
,FileLogger
, andDatabaseLogger
are concrete loggers that inherit fromLogger
. Each logger knows how to handle the log message or pass it to the next logger in the chain.- The arrow from
Logger
toConsoleLogger
,FileLogger
, andDatabaseLogger
signifies that these loggers inherit fromLogger
.
In all these scenarios, the Chain of Responsibility pattern is used because it provides a flexible and dynamic way to handle a request or event. The pattern allows you to decouple the sender of the request from its receivers, and to specify a chain of handlers at runtime. This can simplify your code and make it easier to modify and extend.
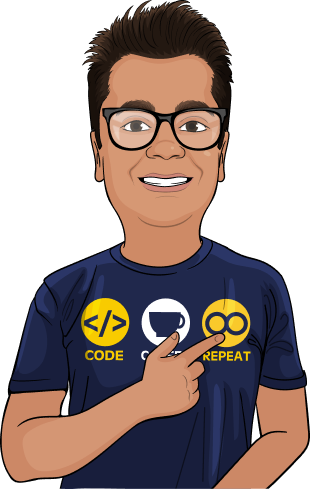
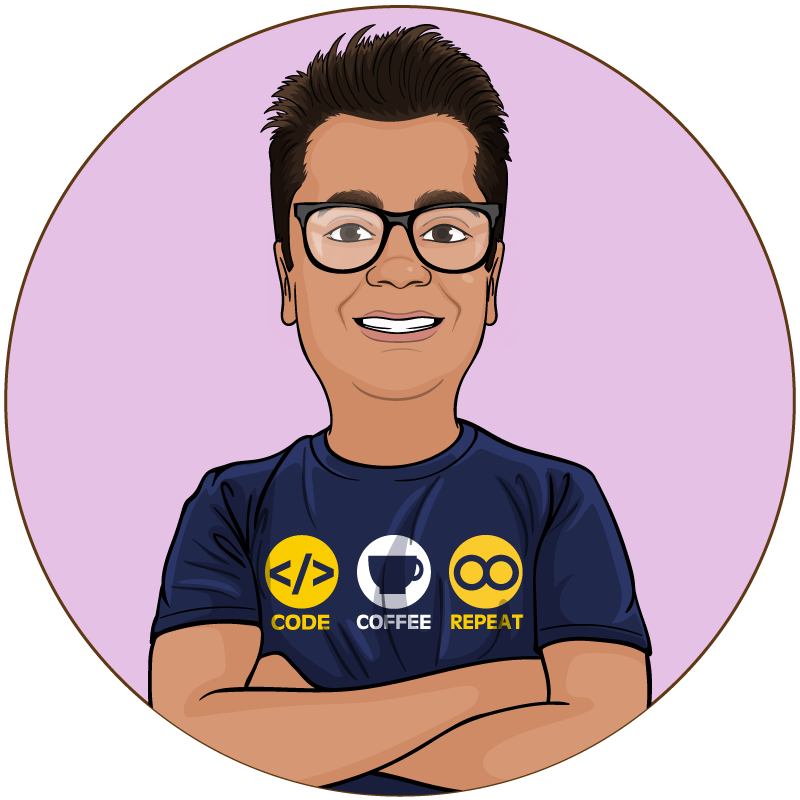
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.