Criticism and Caveats Of Observer Pattern
The Observer pattern, while useful in many scenarios, also has its own set of potential downsides and challenges. Here are some considerations that should be taken into account
Criticism And Caveats Of The Observer Pattern
Unexpected Updates
Since the pattern relies on notifying all observers whenever a change happens, there can be situations where updates are triggered when they are not needed or expected. It's important to manage these notifications effectively to avoid unnecessary updates.
Debugging Difficulty
Debugging and troubleshooting can be challenging, because the Observer pattern can lead to complex chains of reactions that can be hard to follow. These complexities are often introduced by dynamic relationships and broad communication, where changes propagate through a network of loosely coupled objects.
Memory Leaks
You must remember to remove observers from the subject when they're no longer needed, otherwise, this can lead to memory leaks. This is particularly important in scenarios where observers are frequently added and removed.
class WeatherData implements Subject {
removeObserver(o: Observer): void {
const index = this.observers.indexOf(o);
if (index >= 0) {
this.observers.splice(index, 1);
}
}
//...
}
Ordering of Updates
The Observer pattern does not specify the order in which observers receive updates. If there's a requirement for certain observers to be updated before others, additional logic will need to be added to handle this.
Over-notification
In some cases, there might be a need to only update observers when certain properties of the subject change. With the simple implementation of the Observer pattern, any change to the subject results in all observers being notified, which could be inefficient.
In TypeScript specifically, you might face these additional challenges:
Strict Type Checking
TypeScript's strict type checking could lead to more complex code to ensure that objects adhere to the observer and subject interfaces correctly.
Compatibility Issues
While TypeScript brings many benefits, some JavaScript libraries or frameworks might not play well with it, and hence, implementing patterns could be a bit tricky.
While the Observer pattern offers many benefits, these potential downsides and challenges mean it's not the perfect solution for every scenario. It's essential to understand the specific requirements of your software system to decide if this pattern is suitable for your situation.
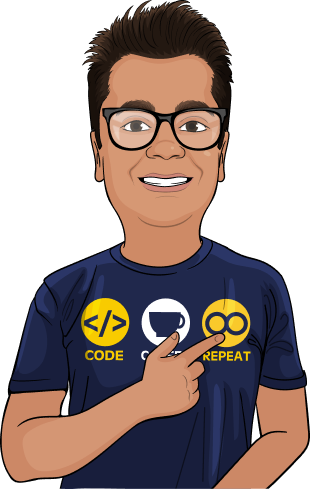
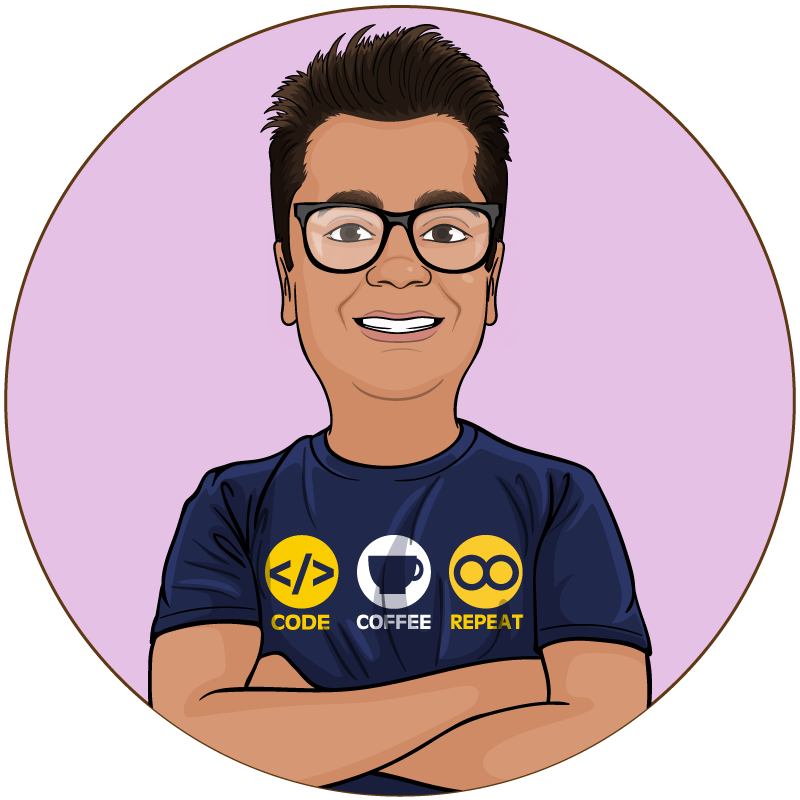
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.