Citicism and Caveats Of Strategy Design Pattern
The Strategy Pattern, like all design patterns, is a powerful tool in software engineering, especially in TypeScript. However, it's not always the best tool for every job, and it does come with its caveats and criticisms.
Here are some of those to consider when deciding whether to use the Strategy Pattern:
Complexity
Strategy Pattern adds complexity to the code, mainly due to the introduction of additional classes or interfaces. If the number of strategies is small and they are not likely to change in the future, then using Strategy Pattern might be an overkill.
Inconsistent Strategies
The "Inconsistent Strategies" caveat refers to the possibility that the different implementations of a strategy interface might not behave in a similar or consistent way. The way it would apply to the code you've posted would look something like this:
Let's say that your FilterStrategy
interface is meant to represent a strategy
for applying a filter to an image. Each class that
implements FilterStrategy
(GrayscaleStrategy
, SepiaStrategy
, NegativeStrategy
)
is supposed to apply a filter and then return the image. However, as per your
current implementation, the apply
method in each strategy does not really
apply any filter to the image, it just logs a message.
Now, suppose we have an inconsistent strategy implementation as follows:
class InconsistentStrategy implements FilterStrategy {
apply(image: string): void {
console.log(`Doing something completely different with ${image}`);
}
}
In the case of InconsistentStrategy
, instead of applying a filter to the
image, it does something completely different. This inconsistency can lead to
unexpected behavior or bugs in the program.
Another scenario could be inconsistent error handling across different strategy implementations. One strategy might throw an exception when an error occurs, while another might swallow the error and log a message or do nothing at all. These inconsistencies can make the strategies difficult to swap in and out, defeating the purpose of the strategy pattern.
Dependency management
Every strategy implemented might come with its own set of dependencies, which can make managing them challenging.
Discoverability
It might be harder for new developers to understand the flow of the application as the logic is spread over different strategy classes.
The strategy pattern can be very beneficial when used in the right context. It supports open/closed principle, makes the code more maintainable, and is a good choice when you have several algorithms that are similar, and you want to choose between them at runtime. However, it's also important to weigh these benefits against the potential drawbacks listed above.
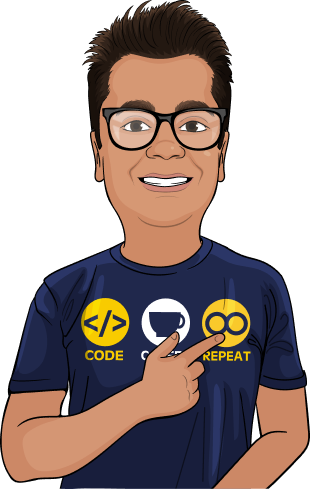
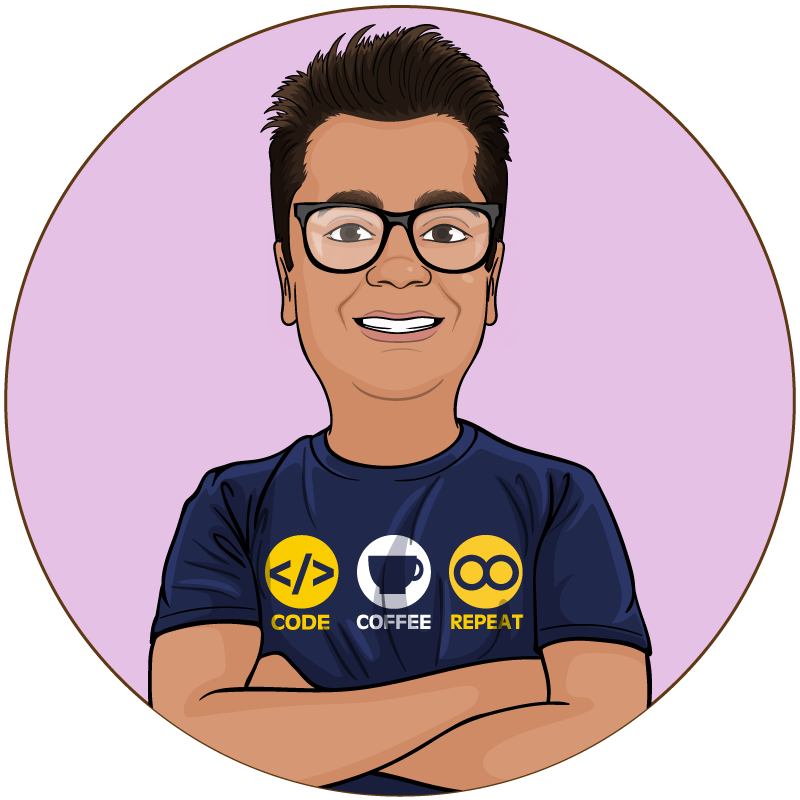
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.