Use Cases Of Strategy Design Pattern
The Strategy Pattern is used in scenarios where a particular task can be performed in different ways and we want to decide how to perform that task at runtime. It helps in selecting an algorithm at runtime without the client knowing the specifics of that algorithm.
Here are some real-world scenarios where the Strategy Pattern is useful:
Sorting Algorithms
In an application where data is sorted in different
ways, the Strategy Pattern can be used to select the appropriate sorting
algorithm at runtime. For example, an e-commerce site might want to sort its
products in different ways - by price, by popularity, by ratings, or by
relevance to the search query. By creating a SortStrategy
interface and
implementing it for each of these sorting algorithms, the site can easily
switch between sorting methods at runtime.
Payment Processing
Here's a class diagram for the Strategy Pattern as applied to payment processing:
In this diagram:
PaymentStrategy
is an interface with aprocessPayment
method.CreditCardStrategy
,PayPalStrategy
, andBankTransferStrategy
are classes that implement thePaymentStrategy
interface. They each provide their ownprocessPayment
method.CheckoutSystem
is a class that has astrategy
property of typePaymentStrategy
, asetPaymentStrategy
method for setting the strategy, and acheckout
method that uses the current strategy to process a payment. The "uses" relationship fromCheckoutSystem
toPaymentStrategy
signifies thatCheckoutSystem
uses thePaymentStrategy
interface.
Online shopping applications often support multiple payment methods, like credit card, PayPal, bank transfer, etc. Each method has its own algorithms for processing payments. Using the Strategy Pattern, each payment method would be a different strategy, and the payment processing engine can select the appropriate one at runtime based on the user's selection.
Compression Algorithms
Here's a class diagram for the Strategy Pattern as applied to compression algorithms:
In this diagram:
CompressionStrategy
is an interface with acompress
method.ZipStrategy
,RarStrategy
, andTarStrategy
are classes that implement theCompressionStrategy
interface. They each provide their owncompress
method.FileCompressor
is a class that has astrategy
property of typeCompressionStrategy
, asetCompressionStrategy
method for setting the strategy, and acompressFile
method that uses the current strategy to compress a file. The "uses" relationship fromFileCompressor
toCompressionStrategy
signifies thatFileCompressor
uses theCompressionStrategy
interface.
In applications that require file compression, the Strategy Pattern can be useful. Different compression algorithms like ZIP, RAR, TAR, etc., can be encapsulated within their own strategy classes. Depending on the situation, different compression strategies can be chosen at runtime.
Image Rendering
Here's a class diagram for the Strategy Pattern as applied to image rendering:
In this diagram:
RenderingStrategy
is an interface with arender
method.SVGStrategy
,BitmapStrategy
, andWebGLStrategy
are classes that implement theRenderingStrategy
interface. They each provide their ownrender
method.ImageRenderer
is a class that has astrategy
property of typeRenderingStrategy
, asetRenderingStrategy
method for setting the strategy, and arenderImage
method that uses the current strategy to render an image. The "uses" relationship fromImageRenderer
toRenderingStrategy
signifies thatImageRenderer
uses theRenderingStrategy
interface.
In graphics rendering, different algorithms can be used to render an image, each with different trade-offs between quality and performance. By implementing each rendering algorithm as a strategy, the rendering engine can choose the best strategy at runtime based on factors like system performance and user settings.
In all of these examples, the benefit of the Strategy Pattern is that the client code that uses these strategies doesn't need to know anything about the specifics of each strategy. It simply calls a method on the strategy interface, and the appropriate strategy implementation takes care of the details. This makes the client code simpler, easier to read, and easier to maintain.
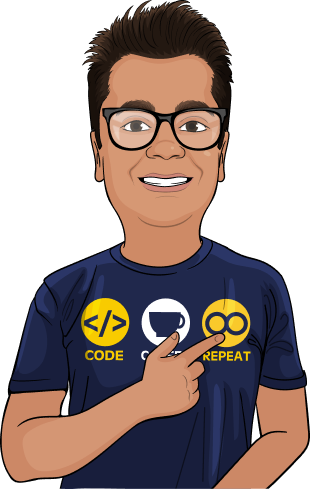
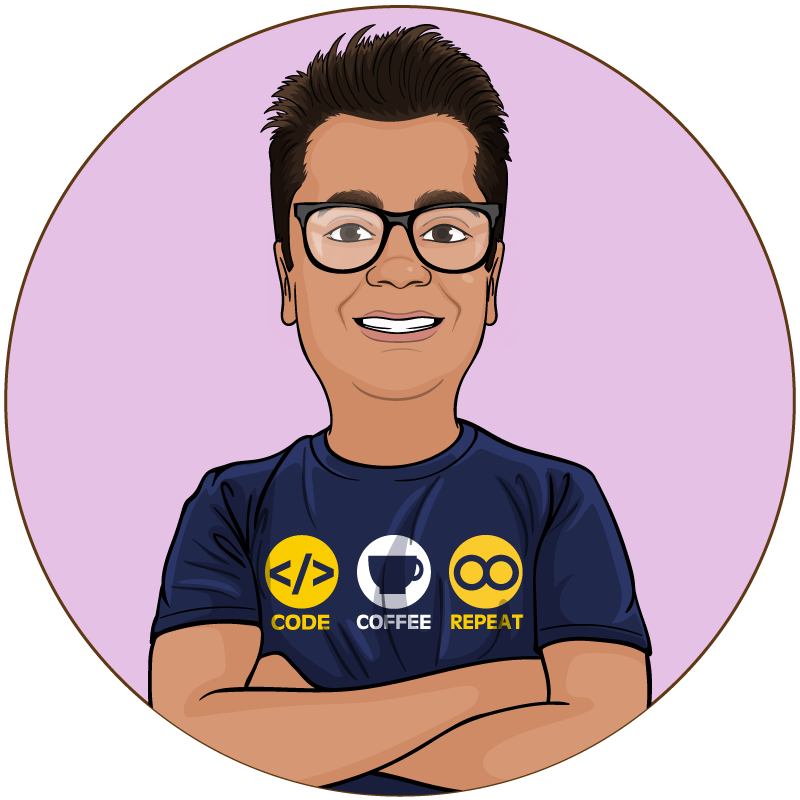
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.