Various Real World Uses Cases Of The Iterator Pattern
The Iterator pattern is broadly applicable in many scenarios where a collection of items needs to be traversed, especially when the underlying data structure of the collection shouldn't be exposed.
Here are a few real-world examples:
File System Traversal
Consider the task of traversing a file system, where you want to apply an operation (like search, delete, or modify) to each file.
Sure, here's a class diagram that represents an implementation of the Iterator Pattern for File System Traversal:
This class diagram represents an application of the Iterator pattern to the file system traversal problem. In this hypothetical design:
FileSystem
is the collection that containsFileNode
objects.FileNode
represents a file or a directory in the file system. A file node can have children if it's a directory.Iterator<T>
is the iterator interface, which is implemented byFileNodeIterator
.FileNodeIterator
is the iterator forFileNode
objects in aFileSystem
. It maintains its current position in the file system and implementsnext
andhasNext
methods.
Here, the Iterator pattern can be applied to abstract the details of the filesystem structure (e.g., directories, subdirectories, etc.) and provide a uniform way to traverse each file.
Why Iterator pattern? The filesystem could be organized as a tree structure or some other complex format. Using an iterator, you can hide this complexity and provide a simple interface to go through each file or directory, one at a time. This makes the client code simpler and decouples it from the specific structure of the filesystem.
Database Query Result Processing
In database operations, you often have to iterate over a set of records returned by a query. An iterator can be used to traverse these records without exposing the details of database access.
Here is a class diagram for "Database Query Result Processing".
In this diagram:
Database
is a class representing the database. It has aquery()
method that returns aResultSet
.ResultSet
is a class representing the result set of a database query. It contains an array ofRow
instances.Row
is a class representing a single row in the result set. It containsdata
, which can be of any type.Iterator<T>
is an interface that defines the contract for an iterator. It includesnext()
that returnsIteratorResult<T>
andhasNext()
methods.IteratorResult<T>
is an interface representing the result of an iteration. It includes avalue
of typeT
and adone
flag to indicate if the iteration is finished.ResultSetIterator
is a class that implements theIterator
interface for aResultSet
. It maintains a reference to theResultSet
it is iterating over and the currentposition
within the result set. It providesnext()
andhasNext()
methods as required by theIterator
interface.
Why Iterator pattern? A database query result could be a large set of records that may not fit in memory. An iterator could help in this scenario by loading records in small chunks (pagination) as the client code traverses the result. This way, it helps manage resources effectively and makes the client code independent of the pagination logic.
Graph Algorithms
Graph algorithms often require traversal, either in depth-first or breadth-first manner. An iterator can be designed to perform these operations seamlessly, without the client needing to understand the internal details of these algorithms. Sure, here's an example of how you can represent a graph data structure using Mermaid. Please note that this is a simple, directed graph.
In this graph:
- Each
Node
represents a vertex in the graph. - The arrows (
-->
) represent directed edges between vertices. For instance,A --> B
represents an edge from Node A to Node B.
Please keep in mind that Mermaid's ability to express complex graph data structures is limited. It is more suited for high-level overviews and diagrams. For detailed manipulations and traversals of graph data structures, you would typically use a programming language with support for complex data structures.
In this diagram:
Graph
is a class representing the graph. It has an array ofVertex
instances.Vertex
is a class representing a vertex in the graph. It contains avalue
, which can be of any type, and an array ofEdge
instances.Edge
is a class representing an edge in the graph. It contains avertex
, which is the vertex the edge connects to.Iterator<T>
is an interface that defines the contract for an iterator. It includesnext()
that returnsIteratorResult<T>
andhasNext()
methods.IteratorResult<T>
is an interface representing the result of an iteration. It includes avalue
of typeT
and adone
flag to indicate if the iteration is finished.VertexIterator
is a class that implements theIterator
interface for a list of vertices. It maintains a reference to the list of vertices it is iterating over and the currentposition
within the list. It providesnext()
andhasNext()
methods as required by theIterator
interface.
Why Iterator pattern? Graphs are complex data structures with nodes and edges. Traversal methods like depth-first and breadth-first require maintaining states and knowing which nodes have been visited. The Iterator pattern can encapsulate this logic within the iterator itself, providing a straightforward way for the client to iterate over the nodes.
Social Media Feeds
Social media platforms like Facebook, Instagram, Twitter, etc., show feeds to the users which are essentially collections of posts, images, videos, etc. These feeds can be iterated using an Iterator pattern to load and display items one at a time or in chunks.
In this diagram:
- A
User
can have multiplePost
instances. - The
Feed
class aggregates posts from multiple users. - The
PostIterator
implements theIterator
interface, providing a unified way to iterate over posts in a feed. - This way, the client code (the code using these classes) does not need to be
concerned with the internal structure of the
Feed
orUser
classes.
Why Iterator pattern? Social media feeds often involve large amounts of data. Loading all this data at once may not be feasible or efficient. The Iterator pattern allows you to load and process data in manageable chunks, providing a smooth user experience.
Remember, the value of the Iterator pattern is in providing a way to access the elements of an aggregate object sequentially without exposing its underlying representation. The examples above are common scenarios where this attribute is particularly useful.
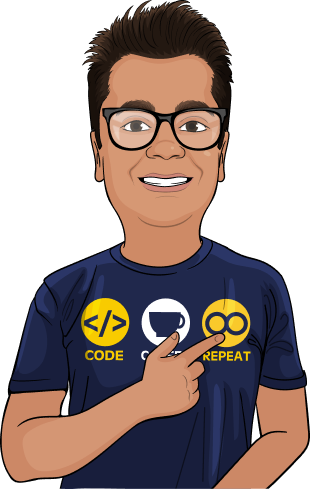
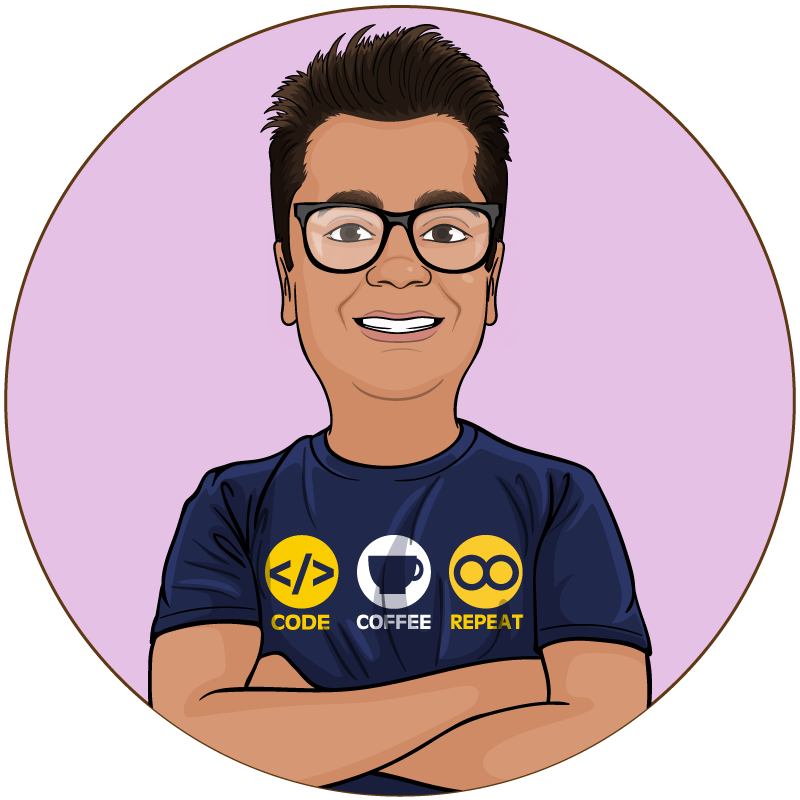
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.