Use Cases Of Observer Pattern
The Observer pattern is commonly used in many real-world applications, particularly when there's a need for multiple objects to stay updated with the state of another object.
Real World Application Of Observer Pattern
Here are some application scenarios where the Observer pattern is typically used:
GUI Interactions and Event Management
One of the most common uses of the Observer pattern is in Graphical User Interfaces (GUIs) of many desktop and web applications. User actions in the GUI (like button clicks, mouse movements, key presses, etc.) can be modeled as events, and various components of the UI can act as observers of these events. When an event occurs, all interested components (observers) get notified and update themselves accordingly.
For example, imagine a text box in a form that the user fills out. When the text in the box changes, various components like character count display, form validation component, 'submit' button's state (enabled/disabled), etc. need to be updated. These components can act as observers, and the text box can act as the subject. Whenever the text changes, all observers get notified and update their state.
Model-View-Controller (MVC) Architectural Pattern
The Observer pattern is a fundamental part of MVC architecture, often used in web and desktop application development. In MVC, the model contains the application's core data and logic, while views display the data, and controllers handle user input. Views act as observers of the model. Whenever the model's state changes (for example, data changes due to some business logic), it notifies all views, which then update themselves to reflect the new state.
Stock Market Applications
In a stock market application, the price of a stock (Subject) can have many observers like investment algorithms, trader dashboards, automated trading systems, etc. These observers need to react immediately whenever there's a change in the price. Using the Observer pattern, the stock object notifies all observers whenever its price changes.
Social Networks and Messaging Platforms
In a social network or messaging platform, let's consider a scenario where
a User
(the subject) has multiple Follower
s or Subscriber
s (observers).
When the user posts a new message, all followers or subscribers get notified.
Here is a class diagram for this scenario using the Mermaid markdown syntax:
In this diagram, User
is the subject that maintains a list of
observers (Follower
and Subscriber
) and notifies them whenever a new message
is posted. Both Follower
and Subscriber
implement the Observer
interface
and update themselves (perhaps displaying the new message somewhere) whenever
they receive a notification from User
.
Again, to render this diagram, make sure you use a markdown editor that supports Mermaid diagrams, or use an online Mermaid live editor to view the diagram.
When a user posts a new message or updates their status on a social network, their followers (observers) are notified about this change. Similarly, in a group chat, when someone posts a message, all members of the group receive this message.
In all these scenarios, the Observer pattern is used because it provides an effective way to keep multiple observers updated with the state of a subject, while keeping the subject and observers loosely coupled. The subject doesn't need to know anything specific about its observers, and new observers can be added or existing ones removed at any time without needing to change the subject's code.
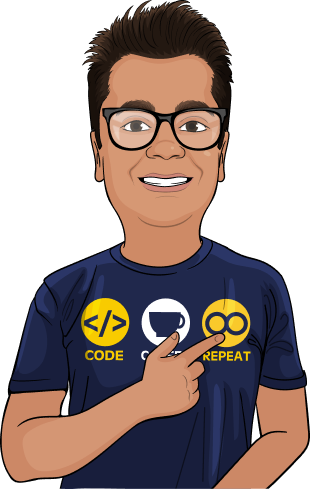
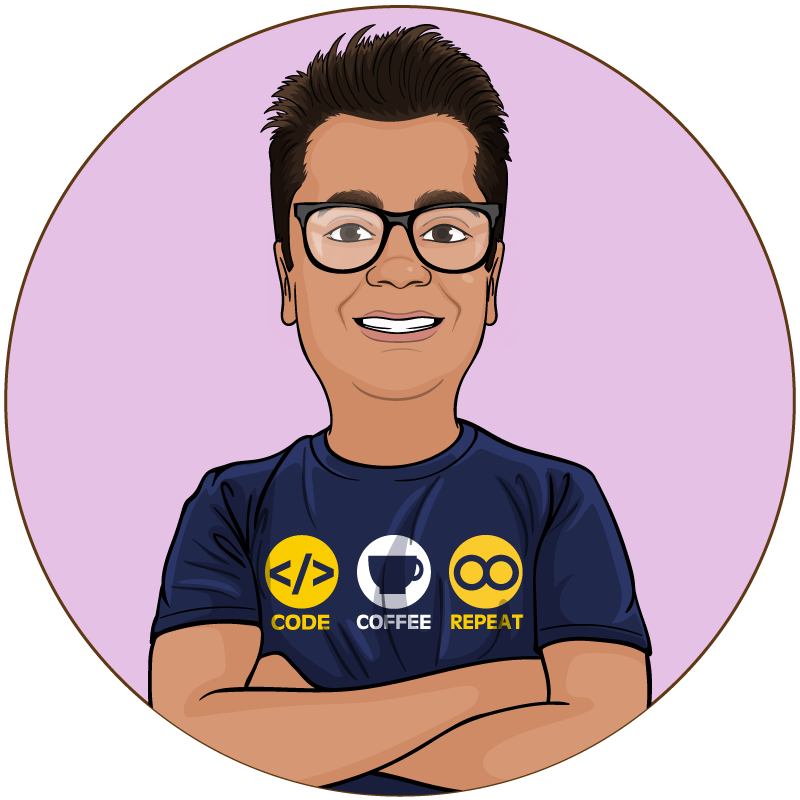
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for ssoftware developers.