Use Cases Of The Template Method Pattern
Real World Use Cases
Here are a few real-world scenarios where you might find the Template Method pattern useful:
Data Parsing and Processing
This diagram represents the class hierarchy for the "Data Parsing and
Processing" example. DataParser
is an abstract class that provides
the parseData
method as well as default implementations
for loadData
, validate
, and useData
methods. The parse
method is
declared as protected and abstract, indicating that it needs to be overridden by
each subclass.
JSONParser
, XMLParser
, and CSVParser
are concrete classes that
extend DataParser
. Each of these classes provides its own implementation of
the parse
method, as per the requirements of the specific data format.
Frameworks and Libraries
Here's a class diagram representing the "Frameworks and Libraries":
In this diagram, RequestHandler
is an abstract class that encapsulates the
overall flow of handling a request (pre-processing, handling, and
post-processing). The handleRequest
method is public and implements this
overall flow, while preProcess
and postProcess
methods provide default
implementations for pre-processing and post-processing steps. The handle
method is declared as protected and abstract, which means it needs to be
overridden by each subclass.
UserRequestHandler
and AdminRequestHandler
are concrete classes that
extend RequestHandler
. Each of these classes provides its own implementation
of the handle
method, tailored to the specific type of request it deals with (
user request or admin request).
Gaming
In this diagram, GameEntity
is an abstract class that encapsulates the overall
lifecycle of a game entity (appear, move, attack, and destroy). The gameLoop
method is public and implements this overall flow, while appear
and destroy
methods provide default implementations for the appearance and destruction of
the game entities. The specificMovement
and specificAttack
methods are
declared as protected and abstract, which means they need to be overridden by
each subclass.
ZombieEntity
and AlienEntity
are concrete classes that extend GameEntity
.
Each of these classes provides its own implementation of the specificMovement
and specificAttack
methods, reflecting their unique behaviors in the game.
In a gaming scenario, suppose you have different kinds of enemies that all have a similar lifecycle: they appear on the screen, move around, attack the player, and finally get destroyed. However, each type of enemy might do these things in a slightly different way. The Template Method pattern would allow you to define the overall lifecycle in a superclass, with subclasses providing their own implementations for each step.
The main benefit of the Template Method pattern in all these scenarios is that it allows you to encapsulate the parts of the process that are the same, while providing flexibility to handle the parts that are different. This makes your code more reusable, easier to manage, and easier to understand.
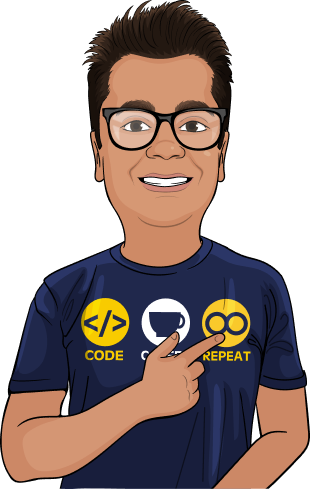
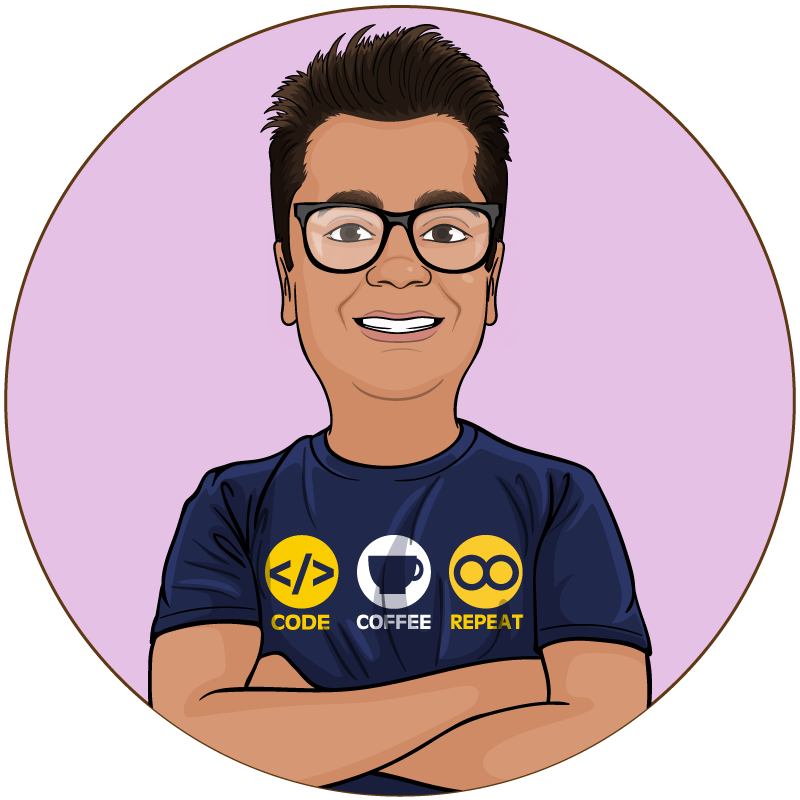
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.