Use Cases For The State Design Pattern
Here are some real-world application scenarios where the State pattern could be an excellent fit.
Workflow Engines
Workflow engines often have complex state transitions and different behaviors associated with each state. For example, a document in a document approval workflow might have states like Draft, Review, Approved, Rejected. The actions that can be performed on the document and the document's behavior will vary depending on its current state. The State pattern can encapsulate this varying behavior within state classes, making the workflow engine easier to manage and extend.
Certainly, here's how the State pattern could be implemented in a Workflow Engine. Here's a class diagram for it:
Here's how the diagram maps to the Workflow Engine:
Workflow
: This class represents a workflow. It maintains a reference to an instance of aWorkflowState
subclass which represents its current state.WorkflowState
: This is an interface that defines a method for processing a workflow. Each state of a workflow will provide its own implementation of this method.DraftState
,ReviewState
,ApprovedState
,RejectedState
: These classes implement theWorkflowState
interface. Each one represents a specific state of a workflow and provides an implementation of theprocess()
method appropriate for that state.The diagram shows that the
Workflow
class has an association with theWorkflowState
interface, and each specific workflow state class implements theWorkflowState
interface.
This design allows the behavior of the Workflow
instance to change dynamically
depending on its current state, in line with the State pattern.
Video Game Development
In video game development, the State pattern can be used to manage the states of game characters. For example, a character might have states like Idle, Running, Jumping, Attacking. The character's animations and behaviors will vary depending on its current state. The State pattern allows these differing behaviors to be encapsulated in separate state classes, making the code more organized and easier to understand.
Sure, let's represent the State pattern for a Video Game Character:
This diagram represents a video game character that could be in different
states (Idle, Running, Jumping, Attacking), and the behavior of the character
changes depending on its state. This is handled by the performAction
method,
which is implemented differently for each state. The GameCharacter
holds a
reference to the current CharacterState
and can change it dynamically by using
the setState
method.
Networking Software
In networking, TCP connections can be in different states - Established, Listen, Closed, Close Wait etc., and the rules for transitioning between states are complex. The State pattern can be used to manage these states and the transitions between them, making the code easier to understand and maintain.
This diagram represents a TCP connection that could be in different states (
Established, Listen, Closed, Close Wait), and the behavior of the connection
changes depending on its state. This is handled by the handle
method, which is
implemented differently for each state. The TCPConnection
holds a reference to
the current TCPState
and can change it dynamically by using the setState
method.
UI Development
In user interface (UI) development, the State pattern can be used to manage the states of UI components. For example, a button might have states like Idle, Hovered, Clicked, Disabled. The appearance and behavior of the button will change depending on its current state. The State pattern allows these differing behaviors to be encapsulated in separate state classes, simplifying the code and making it easier to manage.
This diagram represents a button that could be in different states (Default,
Hover, Pressed, Disabled), and the behavior and appearance of the button changes
depending on its state. This is handled by the handle
method, which is
implemented differently for each state. The Button
holds a reference to the
current ButtonState
and can change it dynamically by using the setState
method.
In all these scenarios, the common factor is that you have an object whose behavior varies depending on its current state, and the rules for transitioning between states can be complex. The State pattern encapsulates the varying behavior within separate state classes, and it manages state transitions, making the code easier to understand, extend, and maintain.
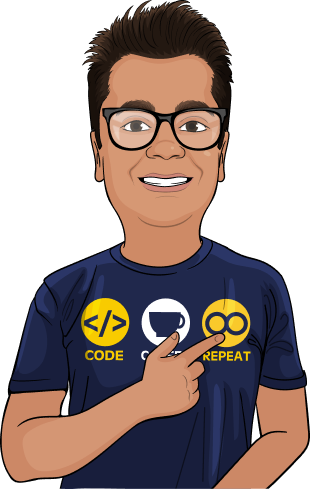
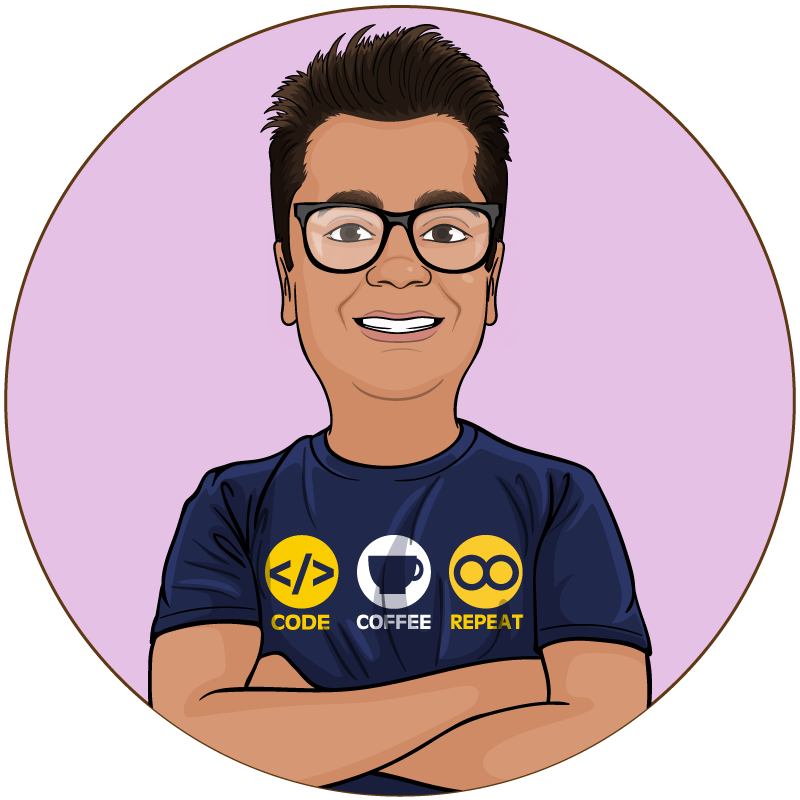
Time To Transition From JavaScript To TypeScript
Level Up Your TypeScript And Object Oriented Programming Skills. The only complete TypeScript course on the marketplace you building TypeScript apps like a PRO.
SEE COURSE DETAILSWhat Can You Do Next 🙏😊
If you liked the article, consider subscribing to Cloudaffle, my YouTube Channel, where I keep posting in-depth tutorials and all edutainment stuff for software developers.